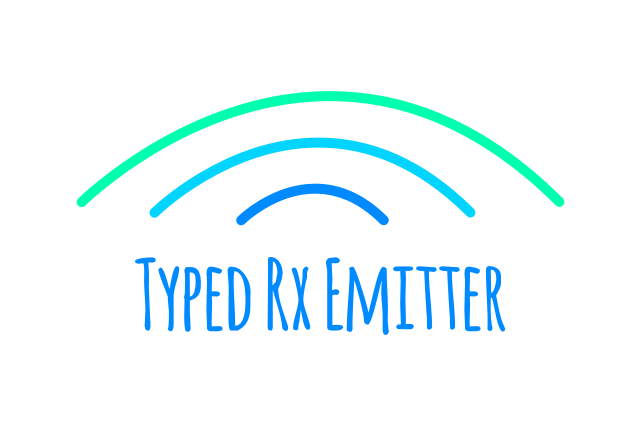
Highlights
- 100% typesafe:
- Statically enforces that channels in
.on()
are defined - Statically enforces that channels in
.emit()
are defined - Statically enforces that emitters are called with the correct data given their Message name
- Statically enforces that listeners are called with the correct data given their Message name
- Statically enforces that channels in
- Supports all RxJS Observable methods
- Supports RxJS versions 4, 5, and 6
- Preforms dynamic analysis to detect and warn about cycles in emitters
- 3.7kb gzipped & minified (using RxJS6), including RxJS
Installation (with RxJS v5.x or v6.x - recommended)
# Using Yarn: yarn add typed-rx-emitter rxjs # Using NPM: npm install typed-rx-emitter rxjs --save
Installation (with RxJS v4.x)
# Using Yarn: yarn add typed-rx-emitter@^0.3 # Using NPM: npm install typed-rx-emitter@^0.3 --save
Usage
// Enumerate messages // Listen on an event (basic)emitter .on'OPEN_MODAL' .subscribeconsole.log`Change modal visibility: ` // _ is a boolean // Listen on an event (advanced) emitter .on'INCREMENT_COUNTER' .pipe filter_ > 3, // _ is a number debounceTime100 .subscribeconsole.log`Counter incremented to ` // _ is a number // Listen on all eventsemitter .all .subscribeconsole.log'Something changed' // Trigger an event - throws a compile time error unless id and value are set, and are of the right typesemitter.emit'OPEN_MODAL', true // Event is misspelled - throws a compile time erroremitter.emit'INCREMENT_CONTER'
See a complete browser usage example here.
Options
Emitter
takes an optional options
argument. options
is an object, and each key in the object is optional:
Option | Default | Description |
---|---|---|
Error |
CyclicalDependencyError |
Custom Error subclass for cycle warning |
isDevMode |
false |
Perform dynamic analysis to warn about cycles? |
Tests
npm test