tʌɪm | measure execution time of functions and promises
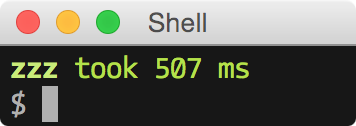
const reallyDumbSleep = { let i = 0; while i < 900000000 i++; } ;
it measures
- execution time of a function
- time until a Promise is resolved
- time until a callback function is invoked
install
$ npm install taim
usage
taim(label?, Function | AsyncFunction) → Function
Returns a decorated version of a function that when invoked, measures and prints the execution time of the function.
If the function returns a Promise or is an AsyncFunction, it will instead measure the time until the returned promise is resolved.
You can optionally pass a label that will shown in the output.
taim(label?, Promise) → Promise
Wraps a Promise (or a thenable) so that when it resolves, duration from
invoking taim
to the promise resolving is printed to stderr.
const p = Promise ; ; // Hello world!
taim.cb(label?, Function) → Function
Returns a decorated version of a function that when invoked with a callback function as the last argument, measures and prints the time until the callback is executed.
const sleeper = taim500 console
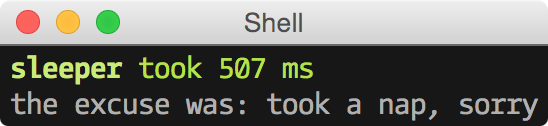
examples
const Promise = ;const request = Promise;const taim = ; // readURLs :: () -> Promise [String]const readURLs = ; // reqHead :: String -> Promise Responseconst reqHead = ; // checkURLs :: [String] -> Promise ()const checkURLs = urls const urls = ;;
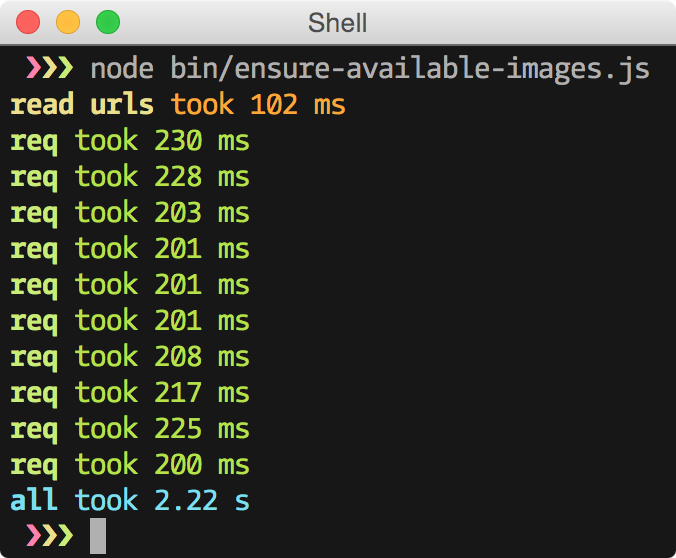
useful vim mappings
These require surround.vim:
" Surround a word with taim()nmap <buffer> <Leader>tr ysiwftaim<CR>f( " Surround a visual selection with taim()vmap <buffer> <Leader>tr Sftaim<CR>f( " Use without requiring separatelynmap <buffer> <Leader>tA ysiwfrequire('taim')<CR>f(vmap <buffer> <Leader>tA Sfrequire('taim')<CR>f(
changelog
1.1.0 - 2019-01-03
- Added: Support for async functions
See also treis
, a tool to debug and observe functions.