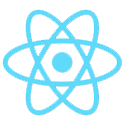
react-device-context
ReactJS Context to detect current device detailsDescription
react-device-context
is a ReactJS context module for exposing User Agent details to be accesible around the application.
Installation
npm install react-device-context
Usage
First, you must wrap your application root component with the DeviceContextProvider
;;; ; ReactDOM.render , document.getElementById'app';
When your application is wrapped with the DeviceContextProvider
, you will be able to consume the API.
API
- Types
- Hooks
- Providers
type Browser
The browser type enumerates the browsers supported by this module. Currently the module supports the following browsers:
Browser | Value |
---|---|
Chrome | 'Chrome' |
Edge | 'Edge' |
Edge Multiplatform | 'EdgeChromium' |
Firefox | 'Firefox' |
Internet Explorer | 'IE' |
Opera | 'Opera' |
Safari | 'Safari' |
Supported browsers with its corresponding value from the Browser
type.
;;
DeviceFormat
DeviceFormat
refers to the device type. Current supported devices are Desktop
and Mobile
.
Hooks
useCurrentBrowser() => Browser | null
useCurrentBrowser
hook returns the current browser as a Browser
type value if the browser is
supported. Otherwise returns null
.
useCurrentFormat() => DeviceFormat | null
useCurrentFormat
hook returns the current device format as a Format
type value.
useDeviceContext() => Context<IDeviceContext>
useDeviceContext
exposes the DeviceContext interface.
The DeviceContext
interface has only one member which is the deviceProfile
, which is used by useCurrentBrowser
and useCurrentFormat
under the hood.
deviceProfile
implements the DeviceProfile
interface which has the following members:
Property | Type |
---|---|
format | DeviceFormat | null |
browser | Browser | null |
useIsMobile() => boolean
A hook that returns true
if the current instance is running in a mobile device, otherwise returns false
.
Providers
<DeviceContextProvider>
The DeviceContextProvider
is the provider for the main context of this module, its recommended to wrap your application with this provider
in order to consume the API.
Contributions
Any contribution is welcome, feel free to open an issue or a pull request. A changelog is beign kept in order to keep track of every change for each release, refer to keep-a-changelog for guiderlines.
License
Licensed under the MIT License.