This is a Custom Object Formatter for rendering JSX objects in Chrome Devtools. Yes, you can see them in the console now!
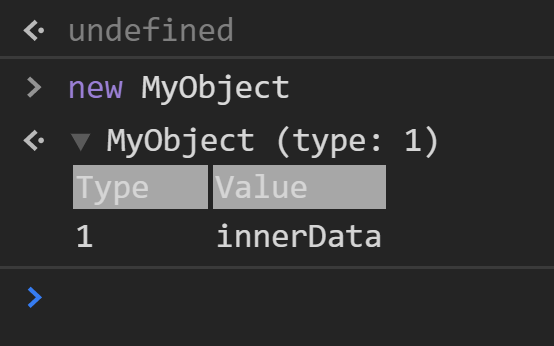
How to use
;React.installCustomObjectFormatternew MyObjectCustomFormatter;
Standard Custom Object Formatters features
div | span | ol | li | table | tr | td | object | |
---|---|---|---|---|---|---|---|---|
style | ✔ | ✔ | ✔ | ✔ | ✔ | ✔ | ✔ | ❌ |
object | ❌ | ❌ | ❌ | ❌ | ❌ | ❌ | ❌ | ✔ |
NON-Standard Custom Object Formatters features
- an
onClick
attribute is available for any tags exceptobject
. Due to technical limitation, theonClick
event will be only fired once. - an
img
tag. With attributessrc
(required),width
(optional) andheight
(optional) - a
code
tag. - a
br
tag. - a
variant
attribute is available for any tags exceptobject
. It can used to specify some default styles.
APIs
- Fragment (Used to support
<></>
syntax) - createElement (used to support JSX)
- createElementTyped (same as createElement, but have a more stricter type)
installCustomObjectFormatter(formatter)
(install the formatter to Chrome devtools)isJSXElement(x)
is it a JSX Elementconst [state, setState, forceRender] = useState(inspectedObject, initialStateCreator)
(used to preserve states between renders)
JSX Features
Basic usage
return <div ="opacity: 0.7;"> Content <span>Note</span> </div>
Display an object
return <span> The explicit way: <object = /> The implicit way: window If window is `null` renderer will ignore this element </span>
Array#map
return <span> data </span>