useComments
React hook to effortlessly add a comment section to your website, and start the discussion on your content.
https://use-comments.netlify.app/
Demo: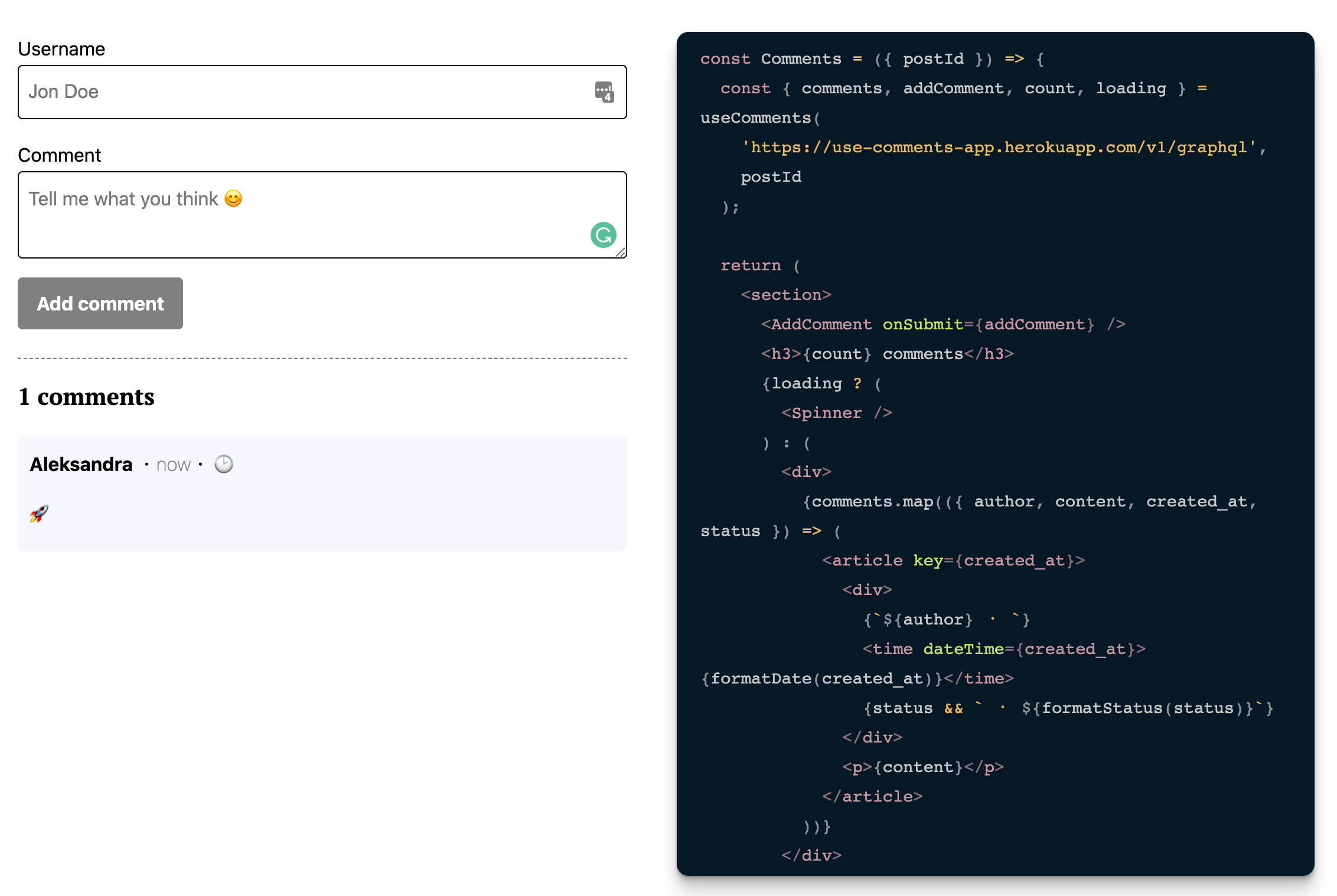
What is it?
🎃 Headless React Hook
useComments
just cares about the data. You write your own UI.
👺 Backed with Hasura
We need to keep all of these comments somewhere, right? Hasura, a GraphQL layer on top of a database, handles this for us! Don't worry, you'll get your Hasura up and running in 30 seconds!
🚀 Quick and easy
The setup will take less than 5 minutes.
Getting started
Let's add comments to a website in five simple steps!
-
Deploy Hasura
Click this 👇 to deploy a fresh Hasura instance.
Deploy Hasura to HerokuWe need to keep all of these comments somewhere, right? Hasura handles this for us.
Check out the docs for more details. You can find more options for one-click deployment there.
-
Set config vars in Heroku
In order to use our Hasura backend we need to set two enviromental variables in Heroku.
HASURA_GRAPHQL_ADMIN_SECRET
will make sure that your GraphQL endpoint and the Hasura console are not publicly accessible.HASURA_GRAPHQL_UNAUTHORIZED_ROLE
will allow unauthenticated (non-logged in) users add and view comments. -
Import database schema and metadata
In the next step we need to import database schema. Click here and copy paste the content.
We also need to import Hasura metadata to set all the permissions. Save this file, and import it in Hasura Console:
-
Install
use-comments
npm install use-comments
yarn add use-comments
or add it from CDN
<scriptcrossoriginsrc="https://unpkg.com/use-comments@0.1.3/dist/index.umd.js"></script><script type="module" async>const useComments = useComments;const comments error =;</script> -
Create beautiful UI for your comments
Start off from one of the examples or write it from scratch.
API Reference
useComments
Fetches comments from Hasura backend specified in hasuraUrl
on mount and
whenever config.limit
or config.offset
change.
Parameters
- hasuraUrl: URL of your Hasura instance. Your comments will be stored there.
- postId: Comments will be fetched for the post with identifier
postId
- config: Configurable offset and limit for the GraphQL query to Hasura. See
UseCommentsConfig
TypeScript Signature
;
UseComentsResult
Returns
Comment
UseCommentsConfig
Allows to implement pagination for the comments. Learn more about implementing pagination..
CommentStatus
When user is adding a new comment it will be in one of four states:
sending
— add comment request is still pending.added
— the comment was successfully added and is visible for other people.delivered-awaiting-approval
— the comment was successfully added, but it's not yet visible for other people. You can make comments to require approval before being visible to others. Read more about it here.failed
— adding a comment was unsuccessful.
declare ;
UseCommentsError