webpack-reporter-plugin
A plugin to customize webpack's output
Table of Contents
Description
There are currently 2 ways of customizing webpack's output, you can set the “stats” option to configure which bundle information you want to display or you can write a plugin (e.g. ProgressPlugin, webpackbar, friendly-errors-webpack-plugin). The second approach gives you more control over the output but requires a good knowledge of how webpack works internally. This plugin abstract over webpack's internals helping writing custom reporters.
Install
You can install the plugin from npm using the following command:
npm install test-webpack-reporter-plugin --save-dev
or
yarn add test-webpack-reporter-plugin -D
Usage
You can use it like any other plugin in your webpack configuration:
webpack.config.js
moduleexports = // ... plugins: // each parameter is optional hooks: defaults: true // wheter or not include the default hooks [default: true] compiler: done: true // listen this hook emit: false // don't listen this hook compilation: buildModule: 5 // log this hook once every 5 times contentHash: '2ms' // log this hook at most once every 2ms reporters: // one or more custom reporters // this is the default one, used if no reporter is given ;
Here's an example of how the output will look like (coloured for readability):
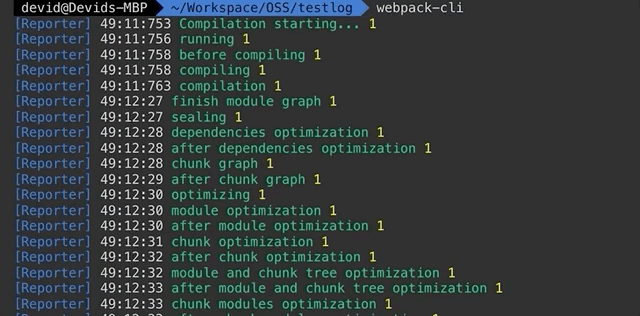
Configuration
This plugin accepts an obect as parameter containing two properties:
reporters (optional)
An array containing one or more reporters that will log the events emitted by this plugin. If not set, a default one will be used.
const MyReporter = ;//... reporters: ;
hooks (optional)
An object used to configure which webpack hooks the plugin should listen and log. It can have those properties:
-
defaults (optional)
Tells the plugin if it should listen to a predefined set of hooks (e.g.
compilation.done
). Setting it tofalse
will exclude every default hook, otherwise its default value istrue
. -
compiler (optional)
Tells the plugin which compiler hooks should be included or excluded
hooks:compiler:beforeRun: false // don't log this hookdone: true // listen this hook; -
compilation (optional)
Tells the plugin which compilation hooks should be included or excluded
hooks:compilation:seal: false // don't log this hookrecord: true // log this hook;
Throttling
Some hooks like compilation.buildModule
may be called many times during a webpack compilation, it is possible to limit the frequency of logging for specific hooks setting a "throttle value" that could be:
-
integer
Meaning that the hook will be logged once every given number of times the hook is called (e.g. once very 2 times)
hooks:compilation:buildModule: 2; -
string
A string encoding a milliseconds value (e.g. "2ms", '20ms') meaning that the hook will be logged once every given milliseconds
hooks:compilation:buildModule: '2ms';
Custom Reporters
This plugin can be extended with one or more reporters. A custom reporter is similar to a usual webpack plugin:
reporter.js
{ // Adds a listener for a specific log reporterhooksinfo; reporterhooksstats; reporterhookserror; reporterhookswarn; } { // print something }
HookData Structure
The reporter plugin has 4 sync waterfall hooks (see tapable): stats
, info
, warn
and error
. Each hook callback receives some data with this structure:
// data emitted by each reporter hookconst hookData = hookId: 'compiler.done' // hook's id count: 0 // counter of times the hook is executed lastCall: 1561725682 // last hook trigger timestamp message: 'Compilation finished' // custom message context: ... // optional, hook context data: {} // custom hook data
Testing
npm run test
🤝 Contributing
Contributions, issues and feature requests are welcome!
Feel free to check issues page.
Show your support
Give a ⭐️ if this project helped you!
👤 Devid Farinelli
- Twitter: @misterdev_
- Github: @misterdev
📝 License
Copyright © 2019 Devid Farinelli.
This project is MIT licensed.
This README was generated with ❤️ by readme-md-generator