🛠 String-mask-jedi

This package allows you to create dynamic masks for the input field with the ability to control the cursor position.
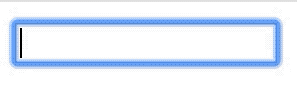
Install
npm install string-mask-jedi
yarn add string-mask-jedi
Usage
Create mask
import { createMask } from "string-mask-jedi";
const phoneMask = createMask("+0 (ddd) ddd-dd-dd", { d: /\d/ });
console.log(phoneMask.run("9998887766").value);
[createMask]
React hook
import * as React from "react";
import { createMask } from "string-mask-jedi";
import { useMask } from "string-mask-jedi/react";
const phoneMask = createMask("+0 (ddd) ddd-dd-dd", { d: /\d/ });
const App: React.FC = () => {
const { value, onChange, ref } = useMask(phoneMask);
return <input value={value} onChange={onChange} ref={ref} />;
};
[createMask]
[useMask]
React hook with use effector
import * as React from "react";
import { createMask } from "string-mask-jedi";
import { useMask } from "string-mask-jedi/react";
import { createEvent, restore } from "effector";
import { useStore } from "effector-react";
const phoneMask = createMask("+0 (ddd) ddd-dd-dd", { d: /\d/ });
const updateValue = createEvent<MaskResult>();
const $value = restore(updateValue, { value: "", cursor: 0 });
const App: React.FC = () => {
const rawValue = useStore($value);
const { value, onChange, ref } = useMask(phoneMask, () => [
rawValue,
updateValue,
]);
return <input value={value} onChange={onChange} ref={ref} />;
};
[createMask]
[useMask]
[UseStateHandler]
API
createMask
type CreateMask = (
stringMask: string,
translations?: Translations,
options?: Partial<Omit<Config, "tokens">>,
) => Mask;
[Translations]
[Config]
[Mask]
Token
Object witch cached value letter when process value after mask running.
interface Token {
value: string;
additional: boolean;
}
State
Object current state mask in processing value after mask running.
interface State {
remainder: string;
tokens: Token[];
cursor: number;
}
[Token]
GetMatch
Method fot get RegExp
for each token.
type GetMatch = (state: State, index: number) => RegExp;
Mask
Restult createMask
.
interface Mask {
run: MaskRun;
config: Config;
}
[MaskRun]
[Config]
MaskResult
Result run mask.
interface MaskResult {
value: string;
cursor: number;
}
TokenConfig
Token config for each letter in created mask.
interface TokenConfig {
getMatch: GetMatch;
defaultValue: string;
additional: boolean;
}
[GetMatch]
Config
Config for create mask.
Please note. createMask
automatically created config by stringMask
, translations
and options
.
interface Config {
tokens: TokenConfig[];
converters: Converter[];
}
[TokenConfig]
[Converter]
Converter
Method for converting result after
type Converter = (tokens: Token[], configTokens: TokenConfig[]) => void;
[Token]
[TokenConfig]
Translation
type Translation = string | RegExp | GetMatch | TokenConfig | Mask;
[GetMatch]
[TokenConfig]
Translations
interface Translations {
[key: string]: Translation | Translation[];
}
[Translation]
MaskRun
type MaskRun = (value: string, cursor?: number) => MaskResult;
[MaskResult]
API for React
useMask
React hook for use mask.
type useMask = <T = HTMLInputElement>(
mask: Mask,
useState: UseStateHandler = React.useState,
) => UseStringMaskResult<T>;
[Mask]
[UseStateHandler]
[UseStringMaskResult]
UseStateHandler
Сtate management handler
type UseStateHandler = (
initialState: MaskResult,
) => [MaskResult, (state: MaskResult) => any];
UseStringMaskResult
Result react hook useMask
.
interface UseStringMaskResult<T = any> {
value: string;
onChange: (
event: React.ChangeEvent<HTMLInputElement | HTMLTextAreaElement>,
) => void;
ref: React.RefObject<T>;
}
Examples
See storybook with examples code.
Tests
npm install
npm run test
yarn install
yarn test