This is a maintained version of react-router v2
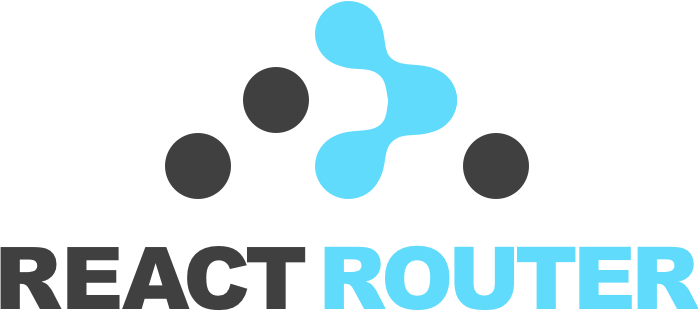
React Router is a complete routing library for React.
React Router keeps your UI in sync with the URL. It has a simple API with powerful features like lazy code loading, dynamic route matching, and location transition handling built right in. Make the URL your first thought, not an after-thought.
Docs & Help
Browser Support
We support all browsers and environments where React runs.
Installation
Using npm:
$ npm install --save react-router-two-legacy
Then with a module bundler like webpack that supports either CommonJS or ES2015 modules, use as you would anything else:
// using an ES6 transpiler, like babel // not using an ES6 transpilervar Router = Routervar Route = Routevar Link = Link
The UMD build is also available on unpkg:
You can find the library on window.ReactRouter
.
What's it look like?
const App = const About = const NoMatch = const Users = const User = // Declarative route configuration (could also load this config lazily// instead, all you really need is a single root route, you don't need to// colocate the entire config).
See more in the Introduction, Guides, and Examples.
Versioning and Stability
We want React Router to be a stable dependency that’s easy to keep current. We take the same approach to versioning as React.js itself: React Versioning Scheme.
Thanks
Thanks to our sponsors for supporting the development of React Router.
React Router was initially inspired by Ember's fantastic router. Many thanks to the Ember team.