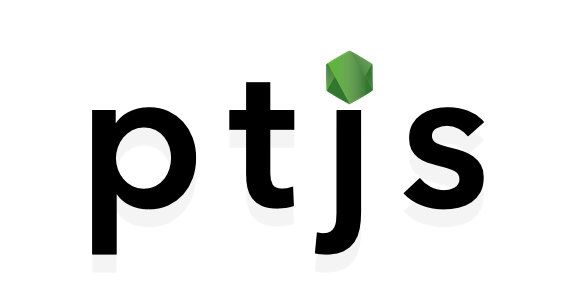
Torch and TorchVision, for your Node servers. Get up and running with PyTorch models within your NodeJS infrastructure in seconds.
Getting Started • Key Features • Development • Misc • License
Getting Started
via yarn
Assuming nothing's broken: yarn add pytorchjs
The same old PyTorch models, in NodeJS
Run your PyTorch models in Javascript, just like you would in Python.
; const load = torch;const DataLoader = torchutilsdata;const ImageFolder = torchvisiondatasets; const Compose Resize InvertAxes Normalize = torchvisiontransforms; const squeezeNet = ;const transforms = height: 224 width: 224 0485 0456 0406 0229 0224 0225 ; const loader = "./test/resources/dataset" 1 transforms;const results = await ;
More Examples
Additional examples of both setup and usage involving features like Torchvision Transforms and CUDA (in development) may be found here.
Key Features
- Run your PyTorch models in a Javascript environment, without worrying about setting up Torchscript or downloading custom binaries
- Deploy your model using configurations identical to what you used during training
- Built-in CUDA support
- CUDA support is a work in progress
- Support for TorchVision, including transforms, dataset classes, and pre-trained models
- Support for TorchVision models is a work in progress
Development
yarn install
should allow you to install project dependenciesyarn test
to run the test suite for this project
Misc
- This project uses arition's fork of torch-js to run TorchScript - check the project out if you're curious about how we do it!
- Distributed under the MIT license. See LICENSE for more information.
- This project was originally developed as a part of COMSW4995 - Open Source Development at Columbia University.