Task
A javascript data type for async requests. Very similar to the data.task and fun-task with some modifications. Published as jordalgo-task
Installing
npm install jordalgo-task
Example
;const task = { const id = ; return { ; };}; const cancel = task;
Quick Details
- The functions passed to run are always called async
- You can't complete a Task more than once e.g. you can't call sendFail and then call sendSuccess (an error will be thrown).
- Functions passed to Task can optionally create a cancel (like above) otherwise cancel will be an no-op.
- It's lazy! The function passed on Task creation is only called when
run
is invoked. - There is no error catching in this Task implementation. Errors are not thrown or caught from within a Task. There are failure values but these are not the same thing as errors -- think of them as "bad news".
Chaining
{ return { // AJAX request to get a user with id ; };} { return { // AJAX request using username ; };} ;
Memoization
A promise-like feature that allows you to hang on to values already processed within a Task. Computations don't get re-run.
var count = 0;const task = { const id = ; return { ; };}; const taskOnce = task; taskOnce;taskOnce;
Parallel Tasks
Parallelize multiple Tasks. Returns an array of successs. If one of the Tasks sends a fail then the cancelation functions for all other Tasks (not yet completed) will be called.
var count = 0; { var order = ++count; return sendFail { var id = ; return { ; }; };} Taskall ;
Specifications compatibility
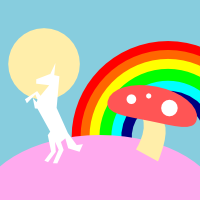
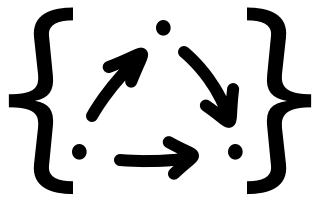
Task is compatible with Fantasy Land and Static Land implementing:
How is this Task different than Data.Task or Fun-Task
- This Task throw an error if you attempt to call sendFail or sendSuccess if either has already been called.
- This Task offers a memoization method that allow you to treat Tasks more like promises so computations don't get called more than once if multiple parts of your code call
run
on an Task. - No special or magical error catching involved.
Credits
A lot of code was inspired and stolen directly from data.task (Quildreen Motta) and fun-task (Roman Pominov).