hubot-line-messaging
This is a Hubot adapter for Line Messaging API and it supports Line Business Center. This adapter currently only supports Reply Message API, but will include more features recently.

Installation
Getting started
Because it is an adapter of hubot, first you must install hubot on your device. Follow the guidance on hubot official document.
Then install this adapter to your project by running
npm install --save hubot-line-messaging
Configuration
There are several environment variables need to be set in your Node.js server
HUBOT_NAME=${your_bot_name}HUBOT_LINE_TOKEN=${your_token}LINE_CHANNEL_SECRET=${CHANNEL_SECRET}# optional # If USER_PROFILE is SET (!==0), you can get additional user profile in res.message.user USER_PROFILE=${USER_PROFILE}
If you are using Heroku, the command will be
heroku config:add HUBOT_NAME=${your_bot_name}heroku config:add HUBOT_LINE_TOKEN=${your_token}heroku config:add LINE_CHANNEL_SECRET=${CHANNEL_SECRET}# optional heroku config:add USER_PROFILE=${USER_PROFILE}
Start robot
Once you configure environment variables on your system well, start your robot as bellow
./bin/hubot -a line-messaging
Usage
Hello World
Create a new file - robot.js under your ./scripts/
folder. Add customize scipts as following in your robot.js
"use strict"; module{ robot;}
Restart your robot, then send a message - @botname hello
in line application. After that, you will receive a response - world
in line application.
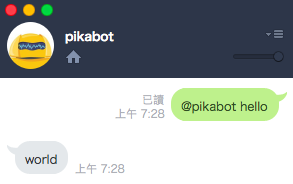
Notice that we support only REPLY MESSAGE API for now, so use res.reply()
or res.emote()
only. res.send()
will reserved until PUSH MESSAGE API is officially supported.
Listen Message from LINE
Text Message
As mentioned in Hubot's document, if your robot's name is BB8, wake it up like below:
- @BB8 ${keyword}
- BB8, ${keyword}
- BB8: ${keyword}
It won't response unless you include robot's name in the beginning of your message.
Complicated Message
Types of supports:
- ImageMessage
- VideoMessage
- AudioMessage
- LocationMessage
- StickerMessage
The following is an example of how to listen and respond to complicated message types (sticker, location, image, ...etc).
var StickerMessage = StickerMessage // Echo the same sticker to Line// Customize a matcher for specific message typevar { // Not implement listener, so should CatchAllMessage.message var stickerMsg = messagemessage; if stickerMsg && stickerMsgtype && stickerMsgtype === 'sticker' ifstickerMsgstickerId === '1' return true return false;}robot;
Respond Basic Message to LINE
There are several message type which is defined in LINE's document. You can require one of those like below
var LineMessaging = var SendSticker = LineMessagingSendStickervar SendLocation = LineMessagingSendLocationvar SendImage = LineMessagingSendImagevar SendVideo = LineMessagingSendVideovar SendAudio = LineMessagingSendAudiosvar SendText = LineMessagingSendText
Text
Text is a common message type, can call it in two way.
// Generate a Text objectlet text1 = 'This is a text'let text2 = 'Second Line' // Reply messageres;
or
// Simply using stringres;
Image
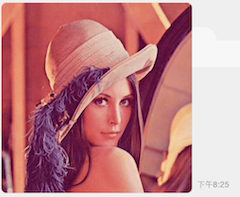
let originalContentUrl = 'https://placeholdit.imgix.net/~text?txtsize=45&txt=480%C3%97480&w=480&h=480';let previewImageUrl = 'https://placeholdit.imgix.net/~text?txtsize=23&txt=240%C3%97240&w=240&h=240'; // Reply messageres;
Video
let originalContentUrl = 'https://example.com/original.mp4'let previewImageUrl = 'https://example.com/preview.jpg' // Reply messageres;
Audio
let originalContentUrl = 'https://example.com/original.m4a'let duration = 240000 // Reply messageres;
Location
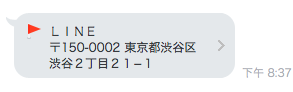
// title, address, latitude, longitudelet location = 'LINE' '〒150-0002 東京都渋谷区渋谷2丁目21−1' 3565910807942215 13970372892916203 ; // Send it as common wayres;
Sticker
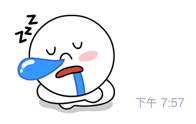
// stickerId, packageIdlet sticker = '1' '1'; // Send it as common wayres;
Respond Template Message to LINE
Template message is composed of template and actions.
Mix Template and Actions
Template includes buttons, confirm and carousel. Actions includes postback, message and uri.
Buttons
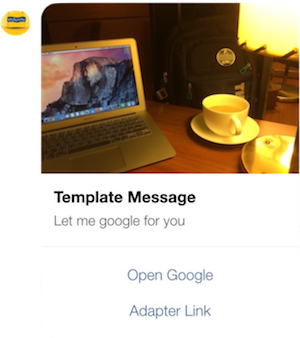
let msg = BuildTemplateMessage action'uri' label: 'Open Google' uri: 'https://www.google.com.tw/' action'uri' label: 'Adapter Link' uri: 'https://github.com/puresmash/hubot-line-messaging' ;res;
Confirm
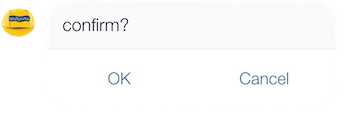
let msg = BuildTemplateMessage action'uri' label: 'OK' uri: 'https://www.google.com.tw/search?q=ok' action'message' label: 'Cancel' text: 'cancel request' ;res;
Carousel
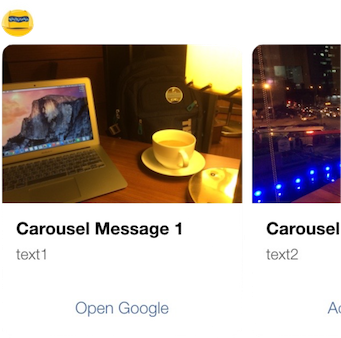
let msg = BuildTemplateMessage action'uri' label: 'Open Google' uri: 'https://www.google.com.tw/' action'uri' label: 'Adapter Link' uri: 'https://github.com/puresmash/hubot-line-messaging' ;res;
Remark
Debug on Heroku
heroku config:add HUBOT_LOG_LEVEL=debugheroku logs --tail