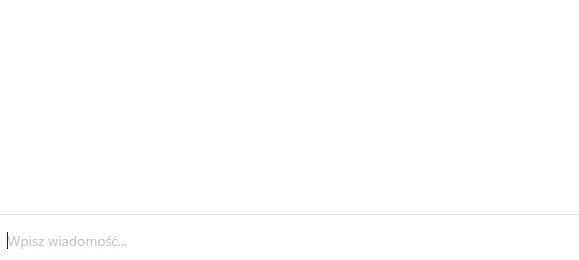
Chat Buttons
An extension for facebook-chat-api
, which provides slightly better UX for your chat bot by adding buttons.
The Problem
Current use of facebook chat bots, works by sending a text command. Unfortunately it's not enough intuitive. The workaround are buttons, which help with some UX problems.
How it works
After sending an url, facebook gets informations about website by searching meta tags. These meta tags are a way to express what a given website is about. This is called prefetching.
For example, if you send an url to website, which looks like this:
You will get this card. As you see, it has title and description.
Chat Buttons
handles these meta informations which goes to facebook and handles if button has been clicked.
Installing
NOTE: To use buttons, you will need to have a public server.
To install Chat Buttons, run in terminal:
$ npm install fb-chat-api-buttons
Quick start
;;; ; ;; loginbotCredentials,; app.listen3000,;
Documentation
Class ChatButtons
new ChatButtons(options: IOptions)
Example:
;;
ChatButtons.setApi
Arguments:
api: any
Example:
loginbotCredentials,;
ChatButtons.send
Arguments:
btn: IButton
threadID: string
Example:
buttons.send , threadID;
IOptions
IButton