catch-react-error
English | 简体中文
Why we create catch-react-error
Introduction
This package supports both React
And React Native
.
This project make it easy to protect your react source code。
We combine decorators and React Error Boundaries together.
The React Error Boundaries don't support the Server Side Rendering,so we use try/catch
to deal such condition.
The catch-react-error takes only one argument,React Error Boundary Component which we provide DefaultErrorBoundary as the default one.
const catchreacterror = {};
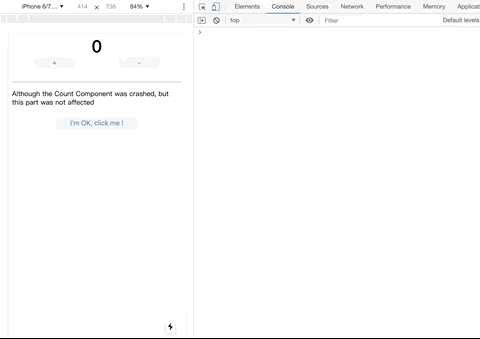
Demo
we provide some demo, so you can understand the library more clearly
client side render
cd example-clientnpm installnpm run dev
server side render
cd example-servernpm installnpm run dev
How to use
1.install catch-react-error
npm install catch-react-error --save
2. Install ES7 Decorator babel plugin
npm install --save-dev @babel/plugin-proposal-decoratorsnpm install --save-dev @babel/plugin-proposal-class-properties
babel plugin configuration
3. import catch-react-error
import catchreacterror from "catch-react-error";
4. Use @catchreacterror on class component
@Component { const count = thisprops; if count === 3 throw "count is three"; return <h1>count</h1>; }
5. Use @catchreacterror on functionc component
{ if count === 3 throw "count is three"; return <h1>count</h1>;} const SaleCount = Count;
6. Add a CustomErrorBoundary component
- First, create an normal Error Boundary Component and change it
Component { ; thisstate = hasError: false; } static { return hasError: true; } { //do something as needed ; } { if thisstatehasError return <h1> Something with my appfallback ui </ h1>; } return thispropschildren; }
- Second, pass it as the argument
@Component
or
const SaleCount = Count;
License
MIT.