react-slots
- A simple react component that uses slots to render children
Installation
# npm
npm i @misa198/react-slots
# yarn
yarn add @misa198/react-slots
Usage
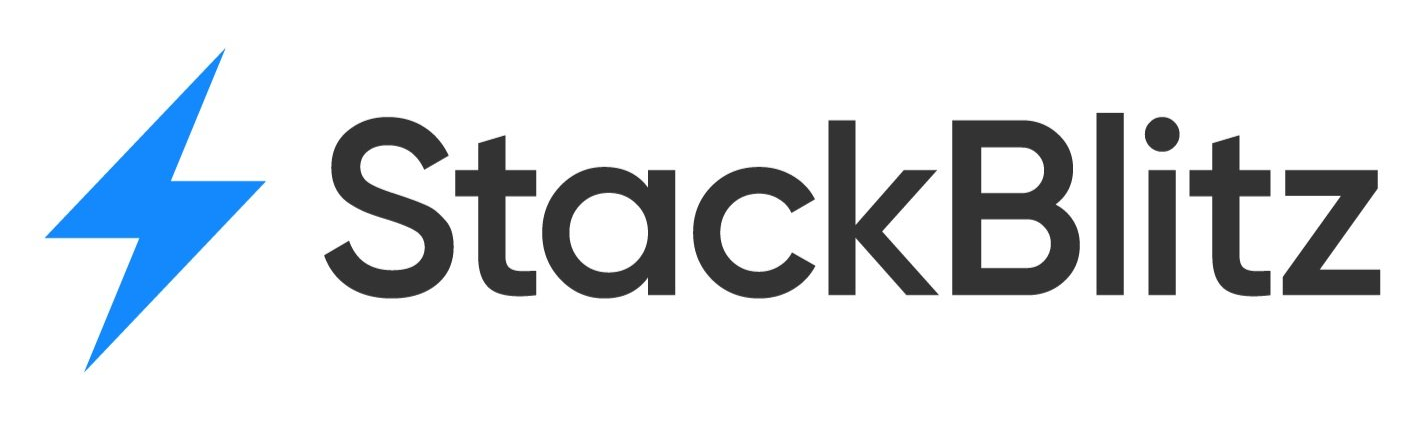
// Parent.tsx
import { Slot, useSlots } from '@misa198/react-slots';
import { PropsWithChildren } from 'react';
export default ({ children }: PropsWithChildren) => {
const slots = useSlots(children);
return (
<div>
<Slot {...slots.slot1} />
<Slot {...slots.slot2} />
<Slot {...slots.slot3} />
</div>
);
};
// App.tsx
import { SlotWrapper } from '@misa198/react-slots';
import { useState } from 'react';
export default () => (
<Parent>
<SlotWrapper name="slot1">
<div>Slot 1</div>
</SlotWrapper>
<SlotWrapper name="slot2">
<div>Slot 2</div>
</SlotWrapper>
<SlotWrapper name="slot3">
<div>Slot 3</div>
</SlotWrapper>
</Parent>
);
Server Side Rendering
SlotWrapper
components must be children of the component when rendered. With the above example:
-
If
App.tsx
is the server side component andParent.tsx
is the client side component, theSlot
components will not be rendered. -
If both
App.tsx
andParent.tsx
are server side components or client side components, theSlot
components will be rendered.