useFetch
🐶 React hook for making isomorphic http requests
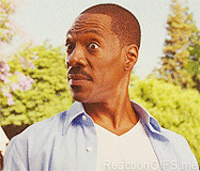
Examples
Installation
yarn add use-http or npm i -S use-http
Usage
Basic Usage
import useFetch from 'use-http' { const options = // accepts all `fetch` options onMount: true // will fire on componentDidMount const todos = const addTodo = todos if todoserror return 'Error!' if todosloading return 'Loading...' return <> <button =>Add Todo</button> todosdata
Destructured methods
var data loading error request = // want to use object destructuring? You can do that toovar data loading error request = request
Relative routes
const request = request
Helper hooks
import useGet usePost usePatch usePut useDelete from 'use-http' const data loading error patch =
Abort
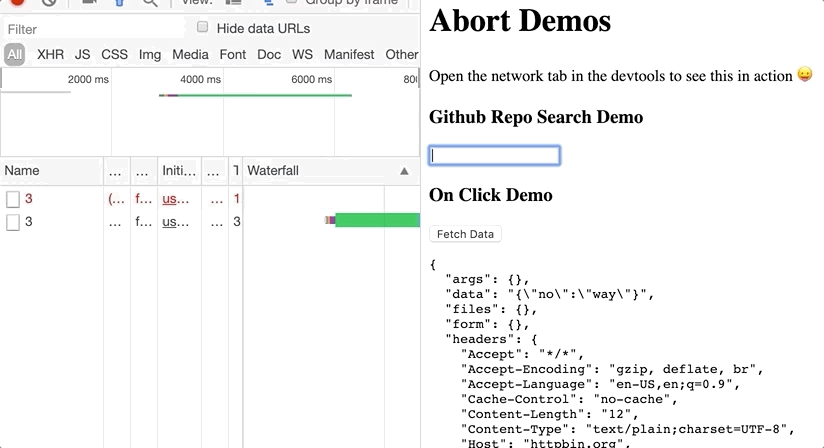
const githubRepos = // the line below is not isomorphic, but for simplicity we're using the browsers `encodeURI`const searchGithubRepos = githubRepos <> <input = /> <button =>Abort</button> githubReposloading ? 'Loading...' : githubReposdataitems</>
Hooks
Option | Description |
---|---|
useFetch |
The base hook |
useGet |
Defaults to a GET request |
usePost |
Defaults to a POST request |
usePut |
Defaults to a PUT request |
usePatch |
Defaults to a PATCH request |
useDelete |
Defaults to a DELETE request |
Options
This is exactly what you would pass to the normal js fetch
, with a little extra.
Option | Description | Default |
---|---|---|
onMount |
Once the component mounts, the http request will run immediately | false |
baseUrl |
Allows you to set a base path so relative paths can be used for each request :) | empty string |
const data loading error request get post patch put delete // don't destructure `delete` though, it's a keyword del // <- that's why we have this (del). or use `request.delete`} =
or
const data loading error request = const get post patch put delete // don't destructure `delete` though, it's a keyword del // <- that's why we have this (del). or use `request.delete`} = request
Credits
use-http is heavily inspired by the popular http client axios
Feature Requests/Ideas
If you have feature requests, let's talk about them in this issue!
Todos
- Make work with React Suspense current example WIP
- Allow option to fetch on server instead of just having
loading
state - Allow option for callback for response.json() vs response.text()
- add
timeout
- add
debounce
- if 2nd param of
post
or one of the methods is astring
treat it as query params - error handling if no url is passed
- tests
- port to typescript
- badges, I like the way these guys do it