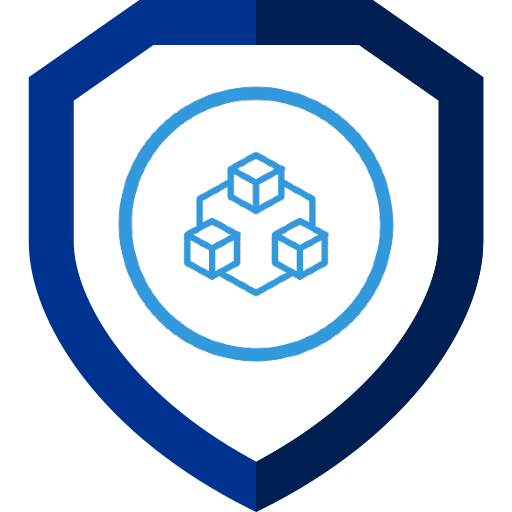
tRPC permissions as another layer of abstraction!s!
Explore the docs »
Report Bug
·
Request Feature
Table of Contents
tRPC Shield helps you create a permission layer for your application. Using an intuitive rule-API, you'll gain the power of the shield engine on every request. This way you can make sure no internal data will be exposed.
- 0.2.0 and higher
- 0.1.2 and lower
npm install trpc-shield
yarn add trpc-shield
- Don't forget to star this repo 😉
import * as trpc from '@trpc/server';
import { rule, shield, and, or, not } from 'trpc-shield';
import { Context } from '../../src/context';
// Rules
const isAuthenticated = rule<Context>()(async (ctx, type, path, input, rawInput) => {
return ctx.user !== null
})
const isAdmin = rule<Context>()(async (ctx, type, path, input, rawInput) => {
return ctx.user.role === 'admin'
})
const isEditor = rule<Context>()(async (ctx, type, path, input, rawInput) => {
return ctx.user.role === 'editor'
})
// Permissions
const permissions = shield<Context>({
query: {
frontPage: not(isAuthenticated),
fruits: and(isAuthenticated, or(isAdmin, isEditor)),
customers: and(isAuthenticated, isAdmin),
},
mutation: {
addFruitToBasket: isAuthenticated,
},
});
export const t = trpc.initTRPC.context<Context>().create();
export const permissionsMiddleware = t.middleware(permissions);
export const shieldedProcedure = t.procedure.use(permissionsMiddleware);
For a fully working example, go here.
export const permissions = shield<Context>({
user: {
query: {
aggregateUser: allow,
findFirstUser: allow,
findManyUser: isAuthenticated,
findUniqueUser: allow,
groupByUser: allow,
},
mutation: {
createOneUser: isAuthenticated,
deleteManyUser: allow,
deleteOneUser: allow,
updateManyUser: allow,
updateOneUser: allow,
upsertOneUser: allow,
},
},
});
Generates tRPC Middleware layer from your rules.
All rules must be created using the rule
function.
- All rules must have a distinct name. Usually, you won't have to care about this as all names are by default automatically generated to prevent such problems. In case your function needs additional variables from other parts of the code and is defined as a function, you'll set a specific name to your rule to avoid name generation.
// Normal
const admin = rule<Context>()(async (ctx, type, path, input, rawInput) => true)
// With external data
const admin = (bool) => rule<Context>(`name-${bool}`)(async (ctx, type, path, input, rawInput) => bool)
Property | Required | Default | Description |
---|---|---|---|
allowExternalErrors | false | false | Toggle catching internal errors. |
debug | false | false | Toggle debug mode. |
fallbackRule | false | allow | The default rule for every "rule-undefined" field. |
fallbackError | false | Error('Not Authorised!') | Error Permission system fallbacks to. |
By default shield
ensures no internal data is exposed to client if it was not meant to be. Therefore, all thrown errors during execution resolve in Not Authorised!
error message if not otherwise specified using error
wrapper. This can be turned off by setting allowExternalErrors
option to true.
allow
,deny
are tRPC Shield predefined rules.
allow
and deny
rules do exactly what their names describe.
and
,or
andnot
allow you to nest rules in logic operations.
and
rule allows access only if all sub rules used return true
.
chain
rule allows you to chain the rules, meaning that rules won't be executed all at once, but one by one until one fails or all pass.
The left-most rule is executed first.
or
rule allows access if at least one sub rule returns true
and no rule throws an error.
race
rule allows you to chain the rules so that execution stops once one of them returns true
.
not
works as usual not in code works.
You may also add a custom error message as the second parameter
not(rule, error)
.
import { shield, rule, and, or } from 'trpc-shield'
const isAdmin = rule<Context>()(async (ctx, type, path, input, rawInput) => {
return ctx.user.role === 'admin'
})
const isEditor = rule<Context>()(async (ctx, type, path, input, rawInput) => {
return ctx.user.role === 'editor'
})
const isOwner = rule<Context>()(async (ctx, type, path, input, rawInput) => {
return ctx.user.role === 'owner'
})
const permissions = shield<Context>({
query: {
users: or(isAdmin, isEditor),
},
mutation: {
createBlogPost: or(isAdmin, and(isOwner, isEditor)),
},
})
tRPC Shield allows you to set a globally defined fallback error that is used instead of Not Authorised!
default response. This might be particularly useful for localization. You can use string
or even custom Error
to define it.
const permissions = shield<Context>(
{
query: {
items: allow,
},
},
{
fallbackError: 'To je napaka!', // meaning "This is a mistake" in Slovene.
},
)
const permissions = shield<Context>(
{
query: {
items: allow,
},
},
{
fallbackError: new CustomError('You are something special!'),
},
)
Shield allows you to lock-in access. This way, you can seamlessly develop and publish your work without worrying about exposing your data. To lock in your service simply set fallbackRule
to deny
like this;
const permissions = shield<Context>(
{
query: {
users: allow,
},
},
{ fallbackRule: deny },
)
This project exists thanks to all the people who contribute.
We are always looking for people to help us grow trpc-shield
! If you have an issue, feature request, or pull request, let us know!
A huge thank you goes to everybody who worked on Graphql Shield, as this project is based on it.
Also thanks goes to flaticon, for use of one of their icons in the logo: Shield icons created by Freepik - Flaticon