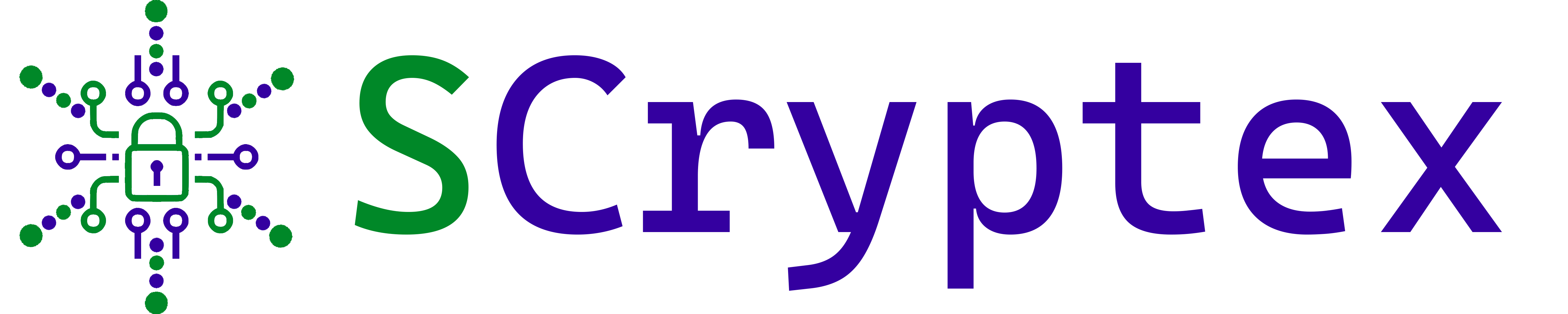
npm i scryptex
import * as SCryptex from "index"; // esm
const SCryptex = require("index"); // cjs
let data = "test", data0 = data, data1 = "Test";
let h = SCryptex.hash(data); // data will be hashed
console.log(SCryptex.compareHash(data0, h)); // true
console.log(SCryptex.compareHash(data1, h)); // false
let {privateKey, publicKey} = SCryptex.generateRSAKeyPair();
let encrypted = SCryptex.RSAEncrypt(data, publicKey); // data will be encrypted
let decrypted = SCryptex.RSADecrypt(encrypted, privateKey);
Generating keys and encrypting
let {privateKey, publicKey, data: encrypted} = SCryptex.RSAEncrypt(data); // data will be encrypted
let rsa = new SCryptex.RSA(); // Keys will be generated
let rsa = new SCryptex.RSA(publicKey, privateKey); // or with generated keys
let rsa = new SCryptex.RSA(publicKey); // or with public key (Decryption is not available)
let encrypted = rsa.encrypt(data); // data will be encrypted
let decrypted = rsa.decrypt(encrypted);