redux-type-actions 
Make redux actions easy to write and type-safe!
No more "UGLY_UPPER_CASE_UNDERSCORE_ACTION"
and bring auto complete
to the redux world!
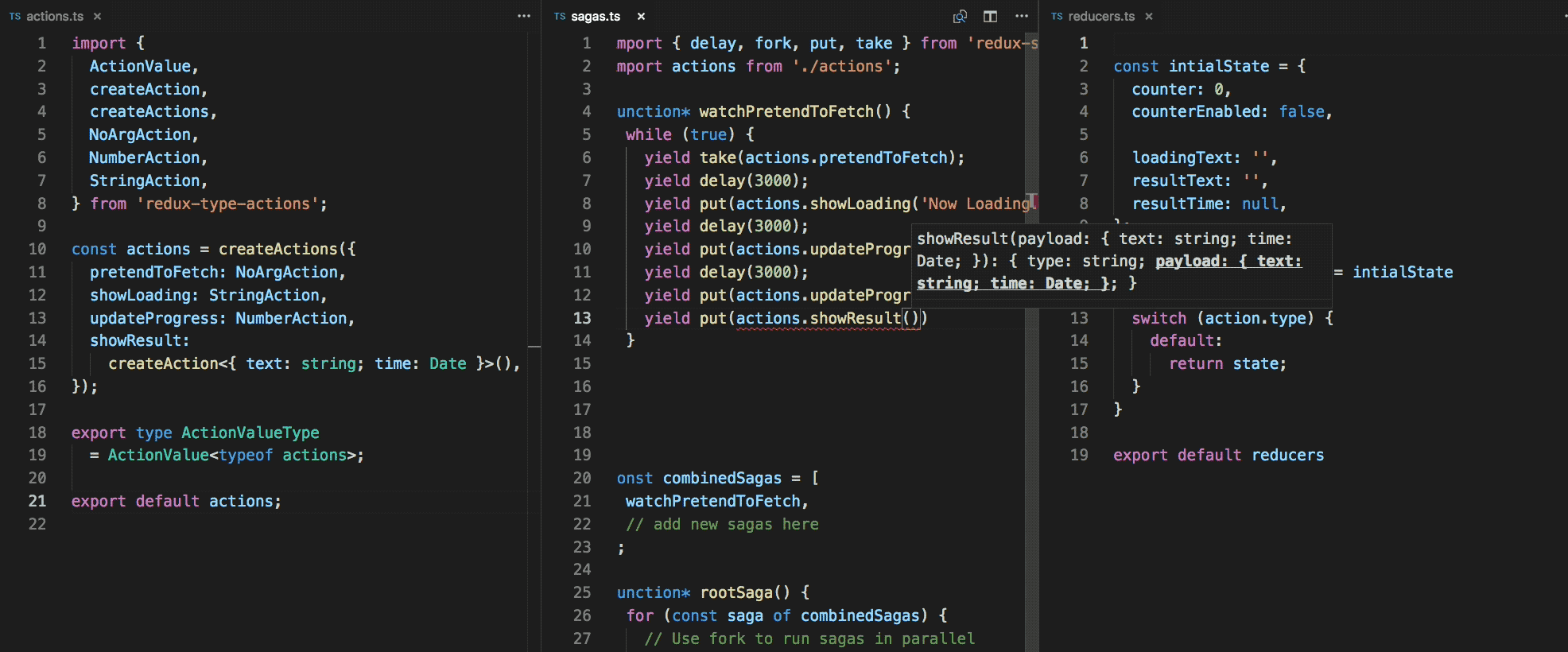
Installation
yarn add redux-type-actions
You don't need any configurations for react-native typescript projects. But if you don't have one,
# Create a new react-native project with typescript support
react-native init MyProject --template typescript
Configurations for web are WIP.
Usage
import {
ActionValue,
createAction,
createActions,
NoArgAction,
NumberAction,
StringAction,
ToggleAction,
} from 'redux-type-actions';
const actions = createActions({
pretendToFetch: NoArgAction,
showLoading: StringAction,
updateProgress: NumberAction,
showResult:
createAction<{ text: string; time: Date }>(),
});
export type ActionValueType
= ActionValue<typeof actions>;
export default actions;
import { ActionValueType } from './actions';
function reducers(state,
action: ActionValueType) {
switch (action.type) {
case 'showResult':
const { text, time } = action.payload;
return {
...state,
resultText: `${text} sent on ${time.toLocaleDateString()}`
}
default:
return state;
}
}
import { delay, fork, put, take } from 'redux-saga/effects';
import actions from './actions';
function* watchPretendToFetch() {
while (true) {
yield take(actions.pretendToFetch);
yield put(actions.showLoading('Now Loading...'));
yield delay(3000);
yield put(actions.updateProgress(50));
yield delay(3000);
yield put(actions.updateProgress(100));
yield put(
actions.showResult({
text: 'Hello',
time: new Date(),
}),
);
}
}