WordInput
is a react functional component for input hinted word.
It take value as the hinted word and callback functions onComplete/onEnter which return the word entered by user.
Working Demo
LIVE DEMO
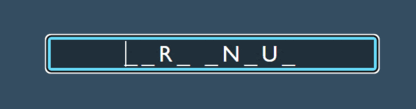
Installation
This module is installed via npm:
npm install react-word-input
Usage
import './App.css';
import { WordInput } from 'react-word-input';
function App() {
const print = (word) => {
console.log(word);
}
return (
<div className="App">
<WordInput className='word' value={'W_R_ __P_T'} onChange={print} autoFocus />
</div>
);
}
export default App;
API
Name | Type | Required | Default | Description |
---|---|---|---|---|
onChange | Function | false | - | Returns word everytime when word is changed |
onComplete | Function | false | - | Returns word when word is filled |
onEnter | Function | false | - | Returns word when user press enter |
value | String | true | - | The value of the hinted word |
className | String | false | - | Class for input element |
disabled | Boolean | false | false | Disables the input |
autoFocus | Boolean | false | false | Auto focuses input on initial page load |
spellCheck | Boolean | false | false | Check is word spells correct or not |