React Sidebar Component
Demo
Check the demo.
Installation
You can install react-side-bar
by executing this command:
npm install --save react-side-bar
When the installation process ends, you are ready to import react-side-bar
to your project.
How to Use
To use react-side-bar
you have to import the component in your project:
;
You can add more properties to react-side-bar
, see the list of available properties in Properties section.
Adding a Button to Open the Sidebar
If you want to add a button for opening and closing react-side-bar
(hamburguer menu for example), you need to reference the state of the sidebar, e.g.:
{ thisstate = opened: false // (or true) }
This way, there are two mandatory methods you need to define
- onOpen (function)
- onClose (function)
The purpose of this properties is to keep the opened property updated. To achieve this, you need to trigger setState
to change the value of the opened property. For exmaple:
Close:
{ this;}
Open:
{ this;}
Moreover you can use this functions to add some extra functionality for your application, such as triggers, when opening or closing react-side-bar
.
Once you have the object with properties, you can init the component Sidebar
.
<Sidebar bar=<div>Amazing Sidebar</div> opened=thisstateopened onClose= { this } onOpen= { this } size=300/>
Output:
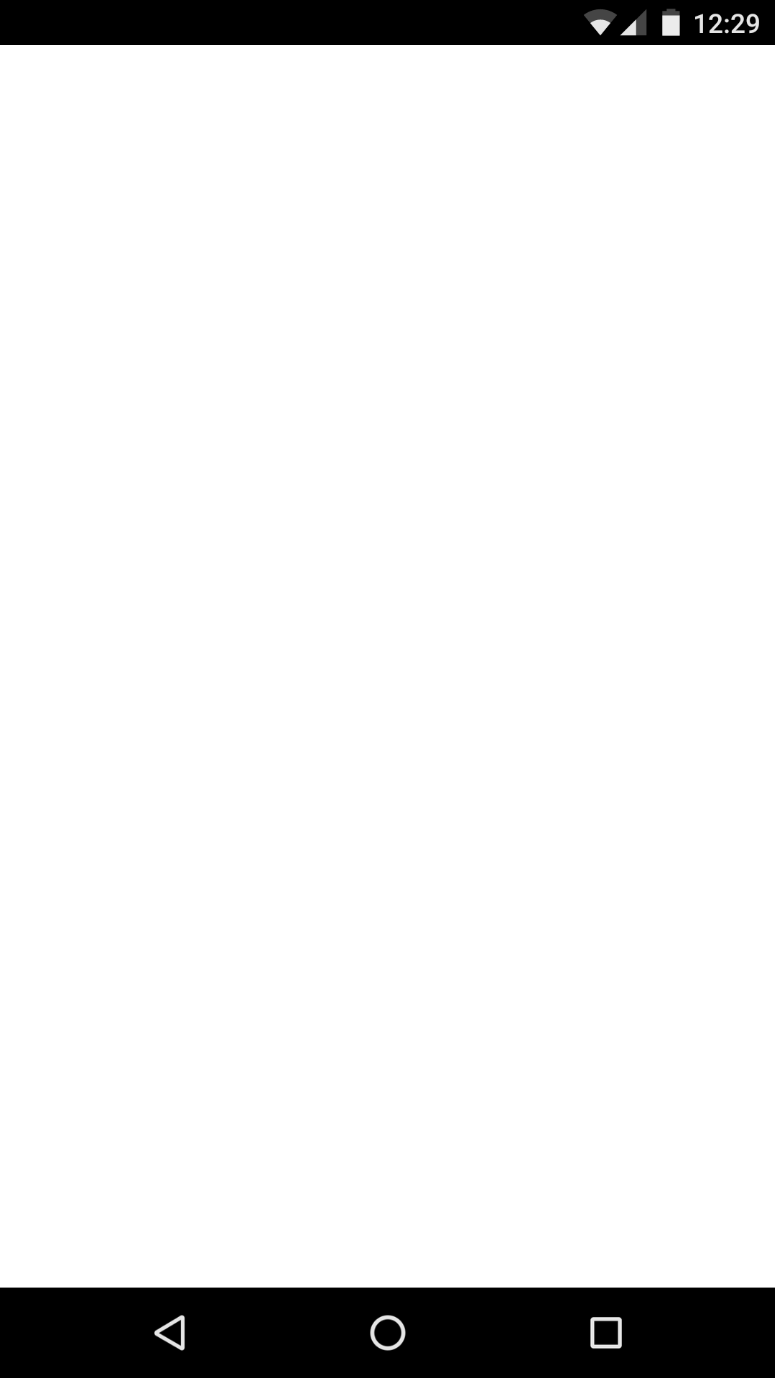

To add content for the application you just have to add children inside Sidebar
.
<Sidebar> <div className='topBar'>SIDEBAR</div> <div className='main'>Main</div></SideBar>
With style and some improvements, it could be like this:
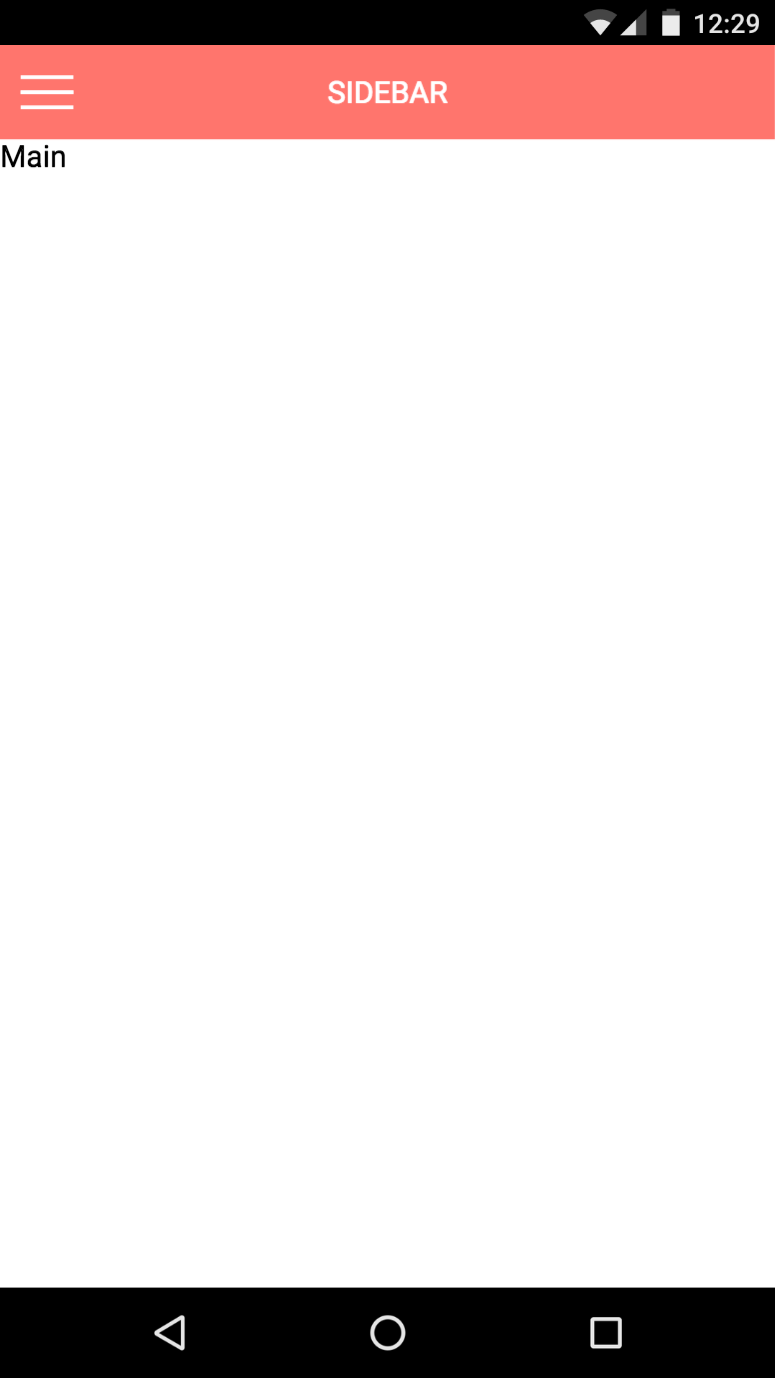

With react-side-bar
you can pass the topBar component (passed as a children in the example above) as a property to change the effect when opening.
<Sidebar bar=<div>Amazing Sidebar</div> topBar=<div className='topBar'>SIDEBAR</div> size=300> <div className='main'> Main </div></SideBar>
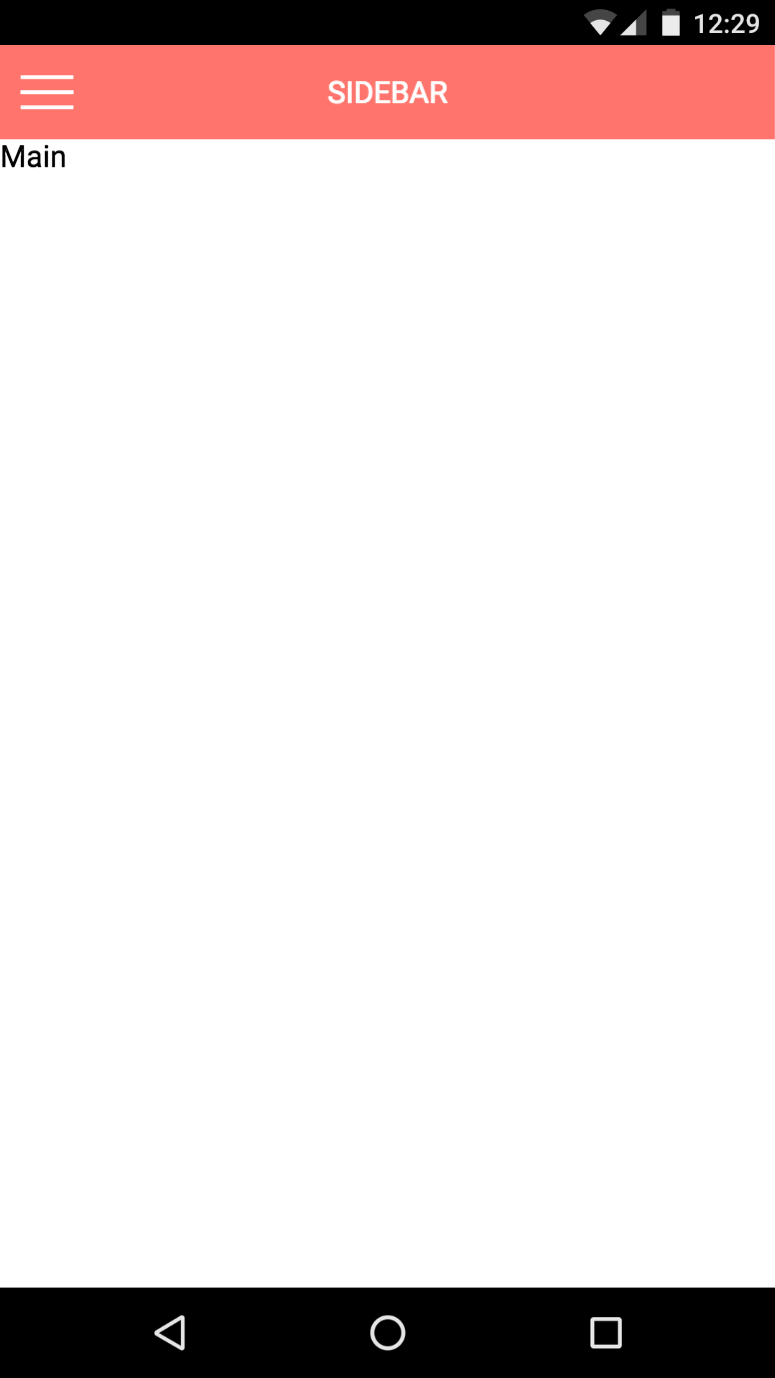

Properties
duration (number)
Default: 150
This is the time it takes to open or close the sidebar.
fx (string)
Default: cubic-bezier(0, 1, 0.85, 1)
This is the effect of the sidebar when opening, o can test the default effect in http://cubic-bezier.com or perhaps add another different effect. Effects
mode (string: Sidebar.BEHIND | Sidebar.OVER | Sidebar.PUSH)
Default: Sidebar.OVER
This property allows to change the opening mode of the sidebar, you can choose from three different:
- Sidebar.BEHIND
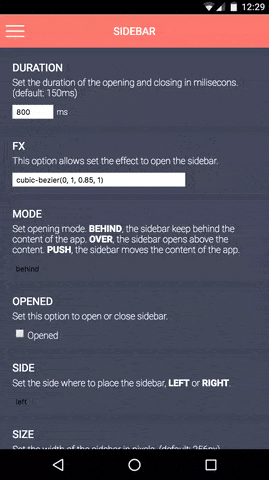
- Sidebar.OVER
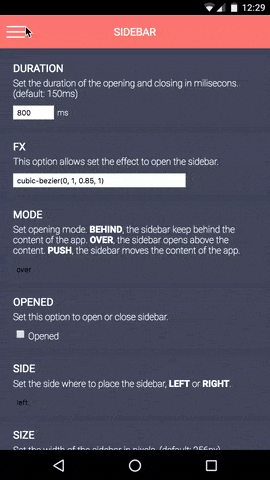
- Sidebar.PUSH
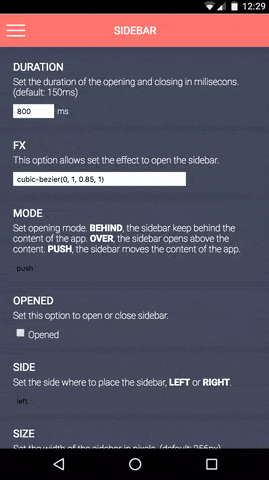
opened (boolean: true | false)
Default: false
This property shows or hides the sidebar. Depending on the sidebar state, its wrapper element features class="side-bar-content opened"
or class="side-bar-content closed"
class names.
side (string: Sidebar.LEFT | Sidebar.RIGHT)
Default: Sidebar.LEFT
This property allows to change the side of the opening of the sidebar.
size (number)
Default: 256
This property allows to change the width of the sidebar.
tolerance (number)
Default: 70
This property sets the tolerance to calculate the sensibility of the automatic opening of the sidebar.
touch (boolean: true | false)
Default: true
This property allows to enable or disable touch events. If its value is false you have to add another alternative method to open sidebar as a hamburguer menu.
touchSize (number)
Default: 80
With this property you can set the size of the touchable zone to start dragging the sidebar. The value of 0
means that you can drag the sidebar everywhere.
veilStyle (object)
Default: {}
You can define the final style of the veil over the content of application when the sidebar is open. While the sidebar is opening, this style have a opacity depending of the veil opening progress.
You can also use CSS to style the veil by applying styles to .side-bar-veil
.
Contribution
If you want to do your own changes, share with community and contribute with the project:
git clone https://github.com/mschez/react-side-bar.git
cd react-side-bar
npm install
npm run dev
Now you can check the demo in http://localhost:3000
Don't forget to check touch actions using the developer tools devices emulation.
Important
If you like this project, don't forget buy me a beer. ¡Thank you!