React Is In Viewport
The component allows to track the other React components whether or not it is in the Viewport.
Installation
To install, you can use npm or yarn:
$ npm install react-is-in-viewport$ yarn add react-is-in-viewport
Props
Name | Type | Default value | Description |
---|---|---|---|
children | React Node or string | React component or string that display in UI | |
delay | number | 100 | Delay time to execute scrolling event callback |
type | 'fit' or 'overlap' | fit | Mode to track component fits in the viewport or overlaps with viewport |
id | string | Identifier of Viewport | |
className | string | Custom CSS class | |
autoTrack | boolean | false | It will count how many seconds the user spending on the component |
waitToStartAutoTrack | number | After waiting how many seconds , then starting the auto track on the component |
The
autoTrack
only works withtype = fit
Here is how type = 'fit'
and type = 'overlap'
look like:
fit
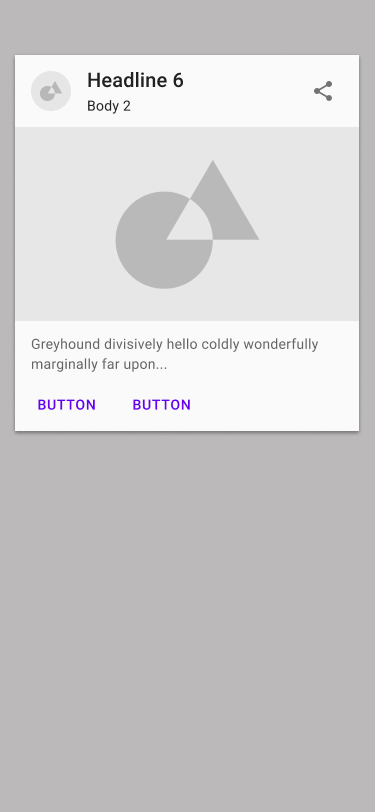
overlap
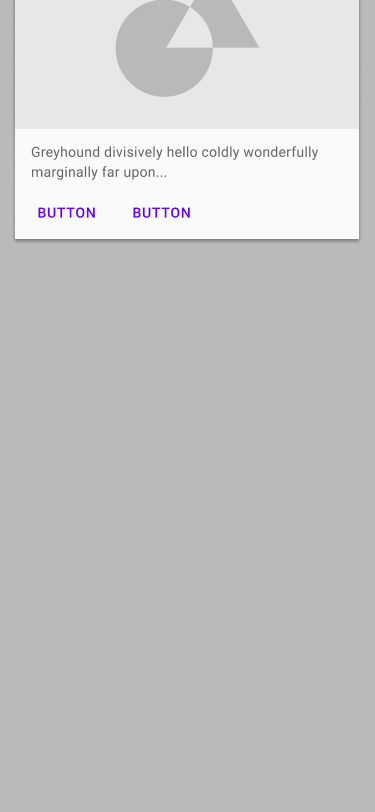
API
Name | Type | Parameter | Description |
---|---|---|---|
onLoad | void | When components first appear and fit in the viewport | |
onEnter | void | enterCount | When scrolling to a component, the enterCount increase 1 |
onLeave | void | leaveCount | When scrolling away from a component, the leaveCount increase 1 |
onFocusOut | void | focusCount | When component is not in the viewport, then onFocusOut called with seconds user spent on the component |
Example
With overlap
& fit
mode
https://codesandbox.io/s/react-is-in-viewport-fit-overlap-mode-zx897
import ReactDOM from 'react-dom';import React from 'react';import Viewport from 'react-is-in-viewport'; Component { return <div> <div => <Viewport ="fit" = = = > <div =></div> </Viewport> </div> <div => <Viewport ="overlap" = => <div =></div> </Viewport> </div> </div> ; } { console }; { console; }; { console; }; { console; }; { console; }; ReactDOM;
With autoTrack
& waitToStartAutoTrack
mode
https://codesandbox.io/s/react-is-in-viewport-autotrack-mode-9f9vz
import ReactDOM from 'react-dom';import React from 'react';import Viewport from 'react-is-in-viewport'; Component { return <div> <div => <Viewport = ="fit" = = = = > <div =></div> </Viewport> </div> <div => <Viewport ="overlap" = => <div =></div> </Viewport> </div> </div> ; } { console }; { console; }; { console; }; { console; }; { console; }; { console; }; ReactDOM;
License
This project is licensed under the MIT License - see the LICENSE file for details