It provides calendar data that you can customize and use as you wish. (in React)
- If you want to use it in a Next.js app router project, use
'use client'
.
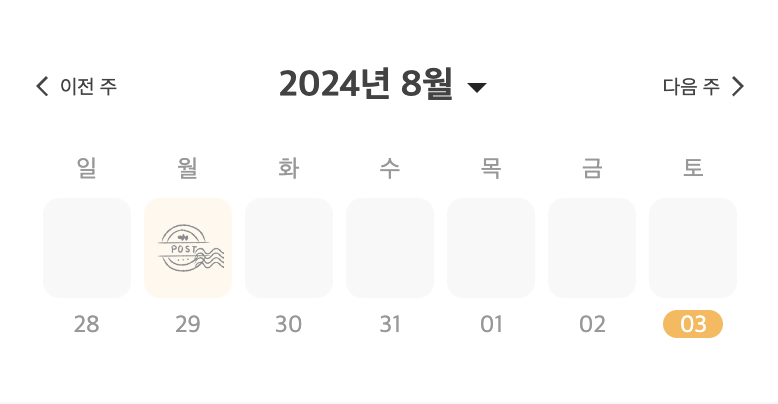
npm i react-custom-calendars
yarn add react-custom-calendars
- All dates are handled in UTC to ensure consistent calendar display worldwide
- Date formatting is handled by date-fns library, supporting various format patterns
-
initialDate
should be provided as a Date
object
import { MonthCalendar } from "react-custom-calendars";
function App() {
const { monthCalendarData } = MonthCalendar({
type: "dd", // date-fns format string
initialDate: new Date(1996, 3, 29), // optional
});
return (
<ul>
{monthCalendarData.map((weeks, weekIndex) => (
<li
key={weekIndex}
style={{
listStyle: "none",
display: "flex",
gap: "10px",
}}
>
{weeks.map((date, dateIndex) => (
<p key={dateIndex} style={{ width: "20px" }}>
{date}
</p>
))}
</li>
))}
</ul>
);
}
export default App;
- You can return date data in monthly calendar format.
- In a week, the last date of the previous month or the start date of the next month is returned as 0.
- If you want to use the included data, you can use WeekCalendar.
- example
// MonthCalendar example
const { currentDate, monthCalendarData, moveToPeriod, moveToNext, moveToPrev } =
MonthCalendar({
type: "dd", // date-fns format string
initialDate: new Date(1996, 3, 29), // optional
});
// Move to next month
moveToNext();
// Move to previous month
moveToPrev();
// Move 2 months forward
moveToPeriod(2);
name |
required |
type |
Description |
Example |
type |
O |
string |
Format of returned date Detail format
|
yyyy-MM-dd , dd , ... |
initialDate |
X |
Date |
Standard for date data to be returned (if there is no value, today's date is returned) |
new Date(2024, 8, 15) |
name |
type |
Description |
currentDate |
string |
Standard for date data to be returned |
setCurrentDate |
function |
A function that changes the currentDate using a Date object as parameter |
monthCalendarData |
string[][] |
Data that can be displayed on a calendar based on currentDate, all dates formatted according to the type parameter |
moveToPeriod |
function |
Moves the calendar by specified number of months. Positive numbers move forward, negative numbers move backward (e.g., moveToPeriod(1) moves to next month, moveToPeriod(-2) moves back two months) |
moveToNext |
function |
Moves the calendar to the next month currentDate |
moveToPrev |
function |
Moves the calendar to the previous month currentDate |
- You can use this when you want to include the last dates of the previous month within a week, or the start dates of the next month.
- For this, use
monthlyWeekGrid
- If you just want a week, use
weekDates
.
- example
// WeekCalendar example
const {
currentDate,
weekDates,
monthlyWeekGrid,
moveToPeriod,
moveToNext,
moveToPrev,
} = WeekCalendar({
type: "dd", // date-fns format string
initialDate: new Date(1960, 1, 1), // optional
});
// Move to next week
moveToNext();
// Move to previous week
moveToPrev();
// Move 2 weeks forward
moveToPeriod(2);
name |
required |
type |
Description |
Example |
type |
O |
string |
Format of returned date Detail format
|
yyyy-MM-dd , dd , ... |
initialDate |
X |
Date |
Standard for date data to be returned (if there is no value, today's date is returned) |
new Date(2024, 8, 15) |
name |
type |
Description |
currentDate |
string |
Standard for date data to be returned |
setCurrentDate |
function |
A function that changes the currentDate using a Date object as parameter |
weekDates |
string[] |
Simple array of dates for current week (Sunday to Saturday) formatted according to the type parameter |
monthlyWeekGrid |
string[][] |
Grid format data that includes dates from adjacent months, all dates formatted according to the type parameter |
moveToPeriod |
function |
Moves the calendar by specified number of weeks. Positive numbers move forward, negative numbers move backward (e.g., moveToPeriod(1) moves to next week, moveToPeriod(-2) moves back two weeks) |
moveToNext |
function |
Moves the calendar to the next week currentDate |
moveToPrev |
function |
Moves the calendar to the previous week currentDate |