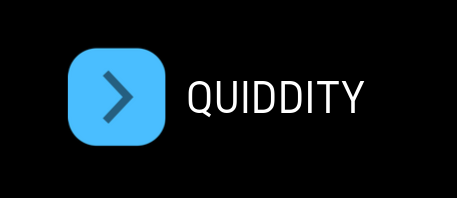
Simple queue server that uses MongoDB to store messages
How it works

- Quiddity is a simple server that connects to MongoDB and exposes few APIs like push, fetch & delete.
- A seperate light-weight agent consumes these APIs and allows the user to push/pull messages from the queue.
- The user can also have multiple agents working on the queue.
How to setup the agent/worker client
Install from NPM
npm install quiddity-agent
Usage
Intitalise the agent simply by creating a new instance -
const Queue =
Create a new queue by passing the queueName -
const queue = "testQueue"
Push new messages into queue -
queue// Above returns a promise
Pull new messages from queue -
queue
Error handling -
queue
Extra functions -
User can also pass a "where" clause which can filter queue messages based on query. All the MongoDB "find" query parameters are valid for this operation.
Check the documentation to understand how you can use this feature. - MongoDB find()
// You can pass them as optional params during initializationconst queue = "testQueue" foo: $gt: 10 // OR you can set them as follows //queuewhere = foo: $gt: 10 queue
To delete all messages from the queue -
queue// Returns the number of messages deleted
You can analyse the messages that end up in dead letter queue -
queue// Returns those messages which were not successfully processed// after maximum number of receives by consumers
Note -
You can also pass the following enviornment variables to configure the agent.
Enviornment Name | Default Value | Datatype |
---|---|---|
QUIDDITY_URL | "http://localhost:3000" | String |
POLLING_INTERVAL | 120000 (2 mins) | Number (in millis) |
-
QUIDDITY_URL
- This is the base url of the quiddity server.
- To check out how to setup the server, to go this repo GitHub
-
POLLING_INTERVAL
- If the queue is empty and there are no messages left, the agent will poll for new messages at a fixed interval.
- Developers can modify this polling time to suit their requirements.