Oceanwind
compiles tailwind like shorthand syntax into css at runtime
This library takes inspiration from Tailwind and utilizes Otion to provide means of efficiently generating atomic styles from shorthand syntax and appending them to the DOM at runtime.
Server side rendering and static extraction is also supported.
⚡️ Check out the live and interactive demo
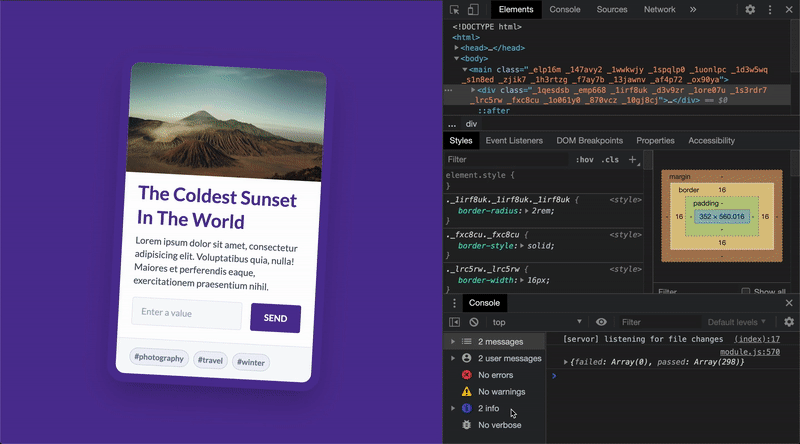
The aim here was to create a compiler that:
- 📖 Supports all existing Tailwind shorthand syntax outlined in the docs
- 💡 Generates only the styles required without building or purging
- 🗜 Is smaller than the average purged css file output from the Tailwind compiler
- ⏱ Has desirable perf characteristics at runtime
- ⚠️ Warns the developer when unrecognized or duplicate shorthand is used
The library currently weighs under 10kb and supports the vast majority of Tailwind directives and variants.
Usage
To use the library, first import the module then invoke the default export using tagged template syntax:
;documentbodyclassName = ow`h-full bg-purple-500 rotate-3 scale-95`;
Running the above code will result in the following happening:
- Shorthand syntax will be translated into CSS (e.g.
h-full -> { height: 100vh }
). - All resultant CSS will be merged into a single CSS-in-JS object
- Each style will be assigned a unique class and appended to a stylesheet
- A string is returned representing all the classes that were created
It is recommended to import the following css files which help normalize styles across browsers:
- The Tailwind reset available here
- The Tailwind prose helper available here
Extending the default theme
Importing and invoking oceanwind directly will cause it to use default theme for directives that require themed values (like bg-red-500
for example). To customize the theme, use the themed
export instead of the default export.
; const ow = ; ow`bg-red-500`; // will result in a hotpink background-color
Any custom theme provided to the themed
function will be deep merged with the default theme.
Function Signature
It is possible to invoke oceanwind in a multitude of different ways. For example:
// Function call passing a string; // Tag Template Literal (falsey interpolations will be omitted)ow`bg-red-500 rounded`;ow`bg-red-500 `; // Function call passing an array (falsey items will be omitted);; // Function call passing an object (keys with falsey values will be omitted);;
Variant Grouping
Directives with the same variants can be grouped using parenthesis. Oceanwind will expand the nested directives; applying the variant to each directive in the group before translation. For example:
Notice any directives within tagged template literals can span multiple lines
ow` sm:hover:( bg-black text-white ) md:(bg-white hover:text-black)`;
It is possible to nest groupings too, for example:
ow` sm:( bg-black text-white hover:(bg-white text-black) )`;
Two things to note here is that the outermost variant should always be a responsive variant (just like in tailwind hover:sm:
is not supported) and that nesting responsive variants doesn't make sense either, for example sm:md:
is not supported.
Catching Errors
By default warnings about missing or duplicate translations will be written to the console:
Clicking on the file path in dev tools will jump to the line in the file in the sources panel.
It is possible to make oceanwind throw an error rather just warning by opting into strict mode:
;
Example
Most of the time developers will be using a front end framework to render DOM elements. Oceanwind is framework agnostic but here is an example of how you might use it with preact and no build step.
⚡️ Check out the live and interactive demo
; ;; const html = htm; ;
Server-side rendering (SSR)
Oceanwind supports SSR through Otion. Consider the following example:
;;;;; const injector = ;; const html = htm;const ow = ;const style = main: ow`clearfix`; const app = html`hello oceanwind`;const appHtml = ;const styleTag = ; // Inject styleTag to your HTML now.
Oceanwind also exposes hydrate
from Otion for client-side hydration. See Otion documentation for further configuration options and usage instructions.
Acknowledgements
I'd like to thank both Adam Wathan and Kristóf Poduszló for their amazing work with Tailwind and Otion respectively, which made making this library somewhat a breeze. Also Phil Pluckthun who helped me deduce the initial grammar.