node-genius-ts
An incomplete Node library to make requests to the genius.com API.
⚠️ This library was developed for my specific usecase so it is missing a lot of functionality (and types) for things I don't need a the moment. Currently it can only get an album, an artist, a track, and tracks of an artist. I'm open for contributions though. If you're looking for a more complete library, check out: node-genius
or genius-api
. For lyrics scraping you could try out genius-lyrics-api
.
⚠️ Since the genius.com API docs are severely lackluster, these types are not guaranteed to be correct or stable. I sampled a lot of responses and used a tool to generate types from these samples. I also omitted some attributes that I didn't need. I still return the complete response from the genius.com API though.
⚠️ All requests are made with the text_format
option set to plain
, this is not configurable at the moment.
Installation
-
Add package
yarn add node-genius-tsor
npm install node-genius-ts -
Generate an API Key from docs.genius.com.
Usage
General
; ;
Environment Variables
Environment Variable | default |
---|---|
GENIUS_API_BASE_URL |
https://api.genius.com |
Functions
getAlbum
try catch err
Params
Option | type | default |
---|---|---|
albumId |
number |
required |
Returns
Option | type |
---|---|
response |
object |
response.album |
GeniusAlbum |
getArtist
try catch err
Params
Option | type | default |
---|---|---|
artistId |
number |
required |
Returns
Option | type |
---|---|
response |
object |
response.artist |
GeniusArtist |
getArtistTracks
try catch err
Params
Option | type | default |
---|---|---|
artistId |
number |
required |
options |
object |
{ page: 1, perPage: 50 } |
options.page |
number |
1 |
options.perPage |
number |
50 |
Returns
Option | type |
---|---|
response |
object |
response.nextPage |
number | null |
response.page |
number |
response.tracks |
GeniusTrack[] |
getTrack
try catch err
Params
Option | type | default |
---|---|---|
trackId |
number |
required |
Returns
Option | type |
---|---|
response |
object |
response.track |
GeniusTrack |
Local Development
This project was bootstrapped with TSDX.
Below is a list of commands you will probably find useful.
yarn dev
or npm run dev
Runs the project in development/watch mode. Your project will be rebuilt upon changes. TSDX has a special logger for you convenience. Error messages are pretty printed and formatted for compatibility VS Code's Problems tab.
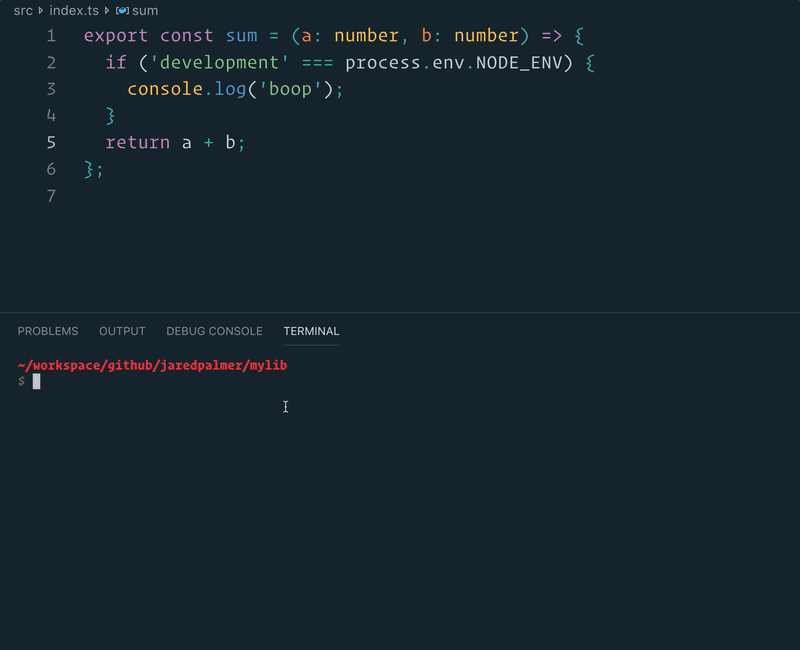
Your library will be rebuilt if you make edits.
yarn build
or npm run build
Bundles the package to the dist
folder.
The package is optimized and bundled with Rollup into multiple formats (CommonJS, UMD, and ES Module).
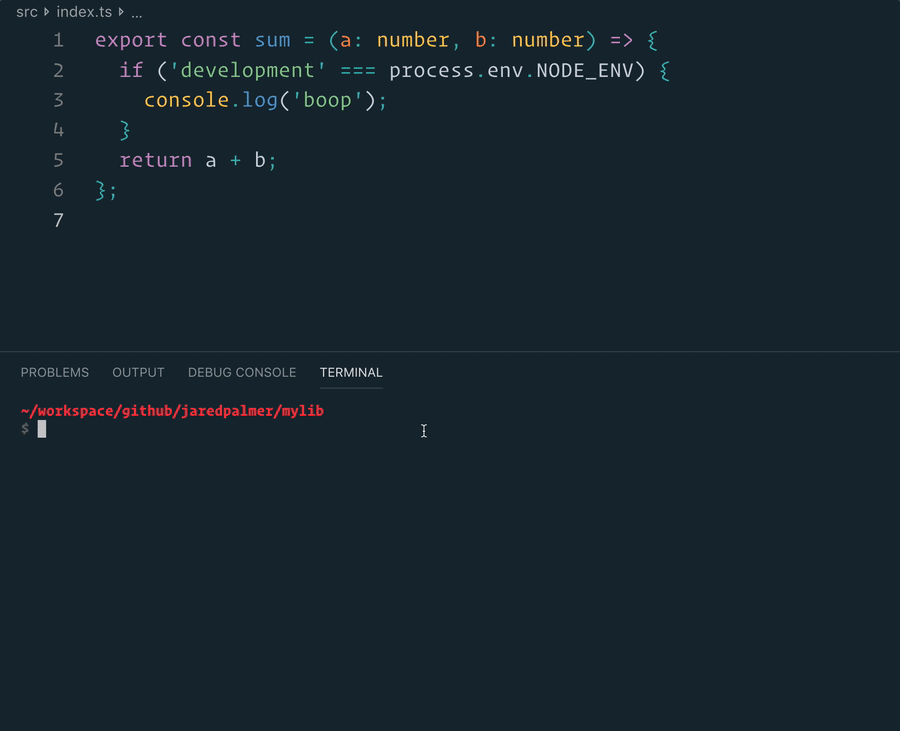
yarn test
or npm test
Runs the test watcher (Jest) in an interactive mode. By default, runs tests related to files changed since the last commit.
Todo
- Export extensible interfaces.