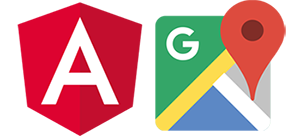
ng-world-map-svg
An Angular 7 plugin that allows:
Installing
$ npm i ng-world-map-svg
Adding angular material
Great now that we are sure the application loaded, you can stop it pressing “Ctrl + c” in the console you are running it, so we can add Angular Material to our application through the schematics.
$ ng add @angular/material
For more details click here.
Setup
...;... @
Click here to get API Key.
Basic Usage
my.component.html
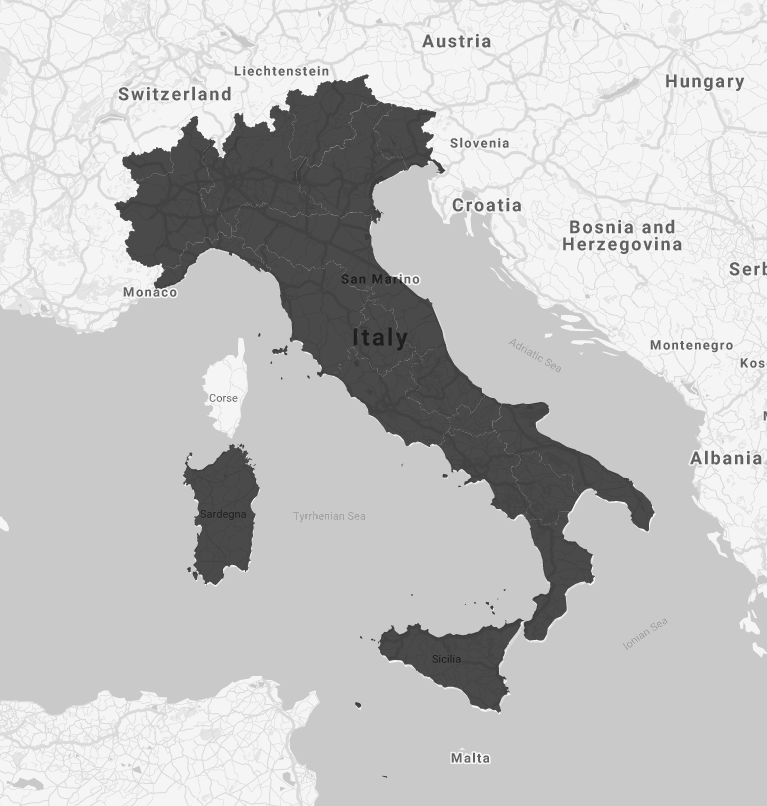
Advanced Usage - Without google map and with custom settings
app.module.ts
...;... @
my.component.html
my.component.ts
;; settings: SettingsVM = ; { } { //Google Map settings thissettingsgoogleMap = false; //Stroke settings thissettingsstrokeCountryColor = "azure"; thissettingsstrokeCountryWidth = "1px"; //Marker color setting thissettingsmarkerColor = "orange"; thissettingslistPoints = city: 'Torino' address: 'Via dell\'Arsenale 35' type: 'marker' city: 'Bari' address: 'Via Giovanni Amendola 162/A' type: 'info' ; }
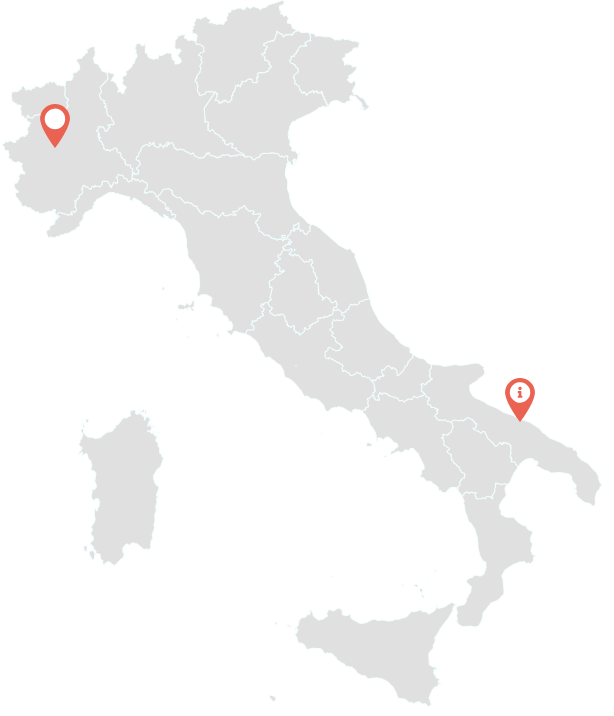
Advanced Usage - With custom settings
app.module.ts
...;... @
my.component.html
my.component.ts
;; settings: SettingsVM = ; { } { //Google Map settings thissettingsgoogleMap = true; thissettingszoomMap = 6; thissettingsstyleGoogleMap = "silver"; //Stroke settings thissettingsstrokeCountryColor = "black"; thissettingsstrokeCountryWidth = "1px"; //Marker color setting thissettingsmarkerColor = "black"; thissettingslistPoints = city: 'Torino' address: 'Via dell\'Arsenale 35' type: 'marker' city: 'Bari' address: 'Via Giovanni Amendola 162/A' type: 'info' city: 'London' address: 'NCP Car Park London Bridgedge' type: 'parking' ; }
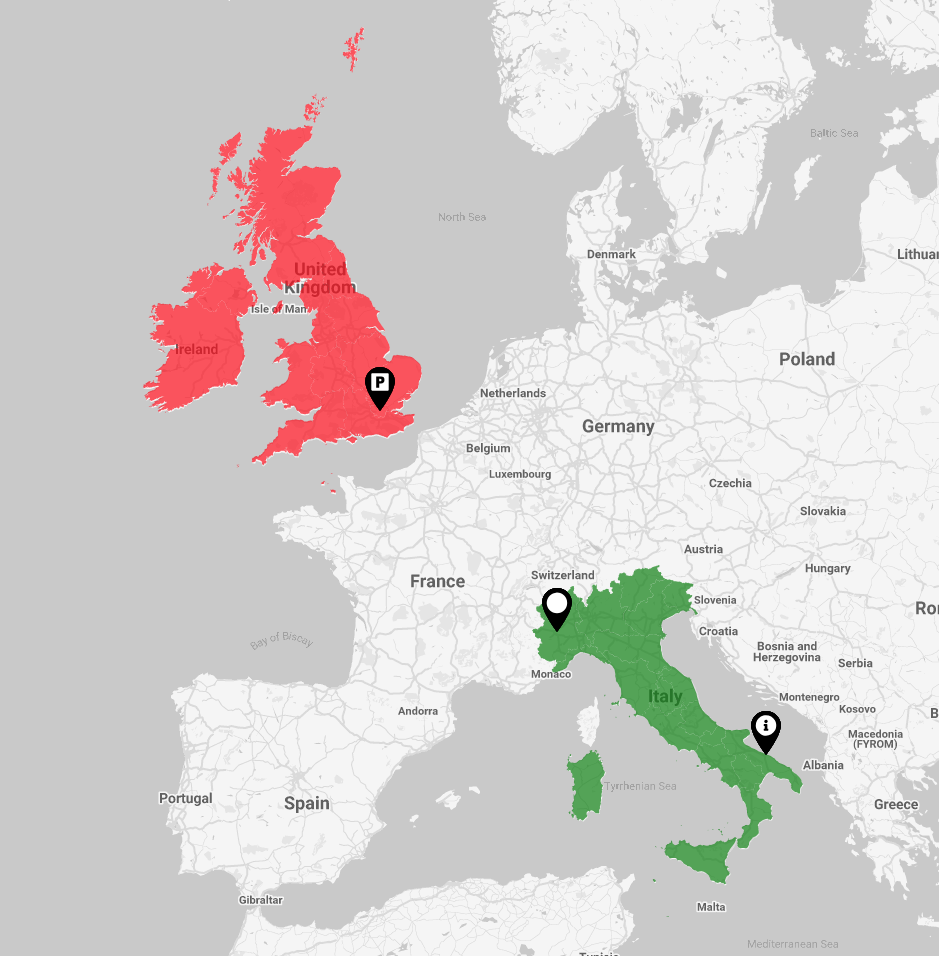
Advanced Usage - With custom settings and modal
my.component.html
Custom template for {{point?.city}}. Address: {{point.address}} Custom template for {{area}}.
my.component.ts
;; settings: SettingsVM = ; { } { //Google Map settings thissettingsgoogleMap = true; thissettingszoomMap = 6; thissettingsstyleGoogleMap = "silver"; //Stroke settings thissettingsstrokeCountryColor = "black"; thissettingsstrokeCountryWidth = "1px"; //Marker color setting thissettingsmarkerColor = "orange"; //Modal settings thissettingsopenModal = true; thissettingsmodalWidth = 50; thissettingslistPoints = city: 'Torino' address: 'Via dell\'Arsenale 35' type: 'marker' city: 'Bari' address: 'Via Giovanni Amendola 162/A' type: 'info' city: 'London' address: 'NCP Car Park London Bridgedge' type: 'parking' ; }
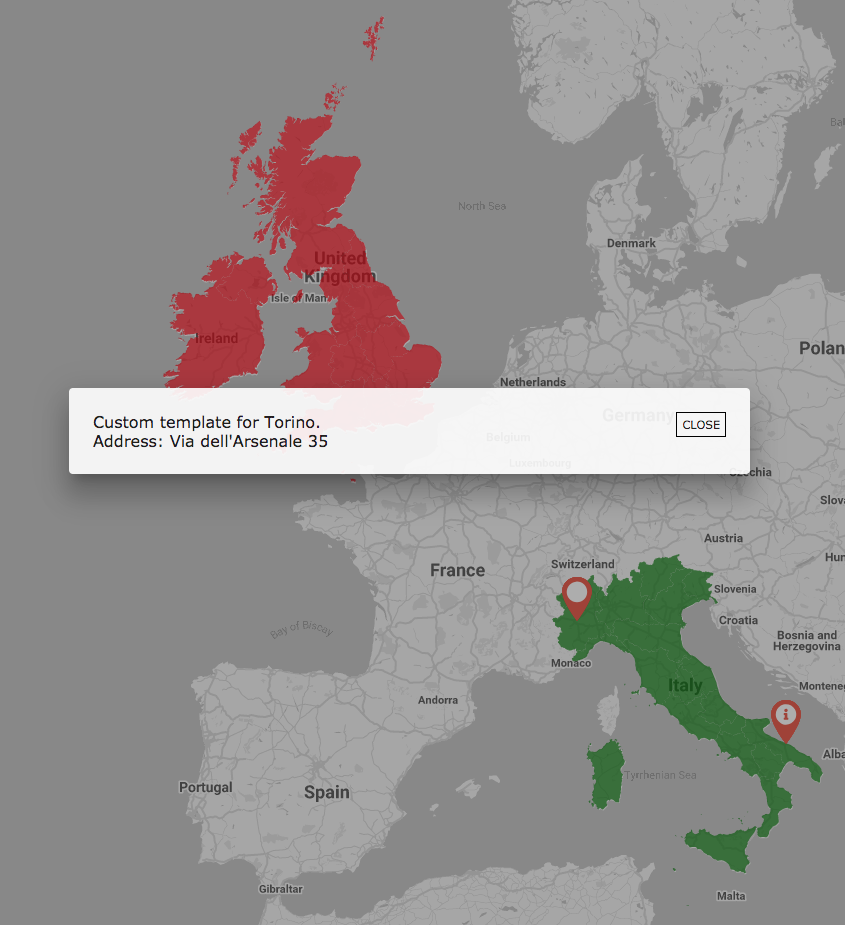
ICustomConfig Attributes Map
Options | Type | Default |
---|---|---|
country | string[] |
[] |
countryColors | string[] |
[] |
googleAPIKey | string |
'' |
SettingsVM Attributes Map
Options | Type | Default |
---|---|---|
googleMap | boolean |
true |
zoomMap | number |
6 |
styleGoogleMap | string |
silver |
strokeCountryColor | string |
white |
strokeCountryWidth | string |
1px |
markerColor | string |
orange |
listPoints | PointVM[] |
true |
openModal | boolean |
false |
modalWidth | number |
30 |
PointVM Attributes Map
Options | Type | Default |
---|---|---|
city | string |
'' |
address | string |
'' |
type | string |
marker |
ICustomConfig Attributes Map Details
country
This value indicates the code of the country to be displayed.
Value | Type | Country |
---|---|---|
IT | string |
Italy |
UK | string |
United Kingdom |
USA | string |
USA |
F | string |
France |
D | string |
Germany |
E | string |
Spain |
N | string |
Netherlands |
PRT | string |
Portugal |
CHE | string |
Switzerland |
IND | string |
India |
BRA | string |
Brazil |
J | string |
Japan |
AUS | string |
Australia |
A | string |
Austria |
HR | string |
Croatia |
CZ | string |
Czech Republic |
DK | string |
Denmark |
EST | string |
Estonia |
FIN | string |
Finland |
IS | string |
Island |
IRL | string |
Ireland |
PL | string |
Poland |
SettingsVM Attributes Map Details
googleMap
If the value is true
the google map is displayed along with the customized map of the various countries. If the value is false
, only the first nation image of the country
array is displayed.
zoomMap
This value sets the zoom level of the google map.
styleGoogleMap
This value sets the style of the google map. Allowed values are:
silver
retro
dark
night
aubergine
strokeCountryColor
This value sets the color of the line that separates the various areas of the displayed countries. It is used only if googleMap
is set to false
.
strokeCountryWidth
This value sets the width
of the line that separates the various areas of the displayed countries. It is used only if googleMap
is set to false
.
markerColor
This value sets the color of the map marker. Allowed values are:
orange
white
black
listPoints
This array contains the list of the various points to display on the map. it's an array of PointVM
type.
openModal
If the value is true
when a marker or area is clicked, a modal is opened that uses the template that is passed to it. If it is false
, the value in output is received through (clickPoint)
and (clickArea)
modalWidth
This value sets the width of the modal. It is used only if openModal
is set to true
. Note that this value is in percentage.
PointVM Attributes Map Details
type
This value indicates the type of pin to be used for places. Allowed values are:
marker
info
parking
restaurant
city
This value indicates the city.
address
This value indicates the address of place.