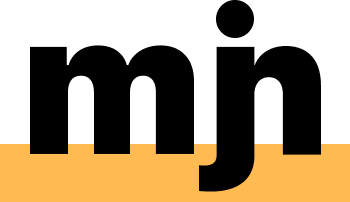
Hello {maybe(user, "name.first_name")}!
Simple utility to check if a key or a value exists in an object.
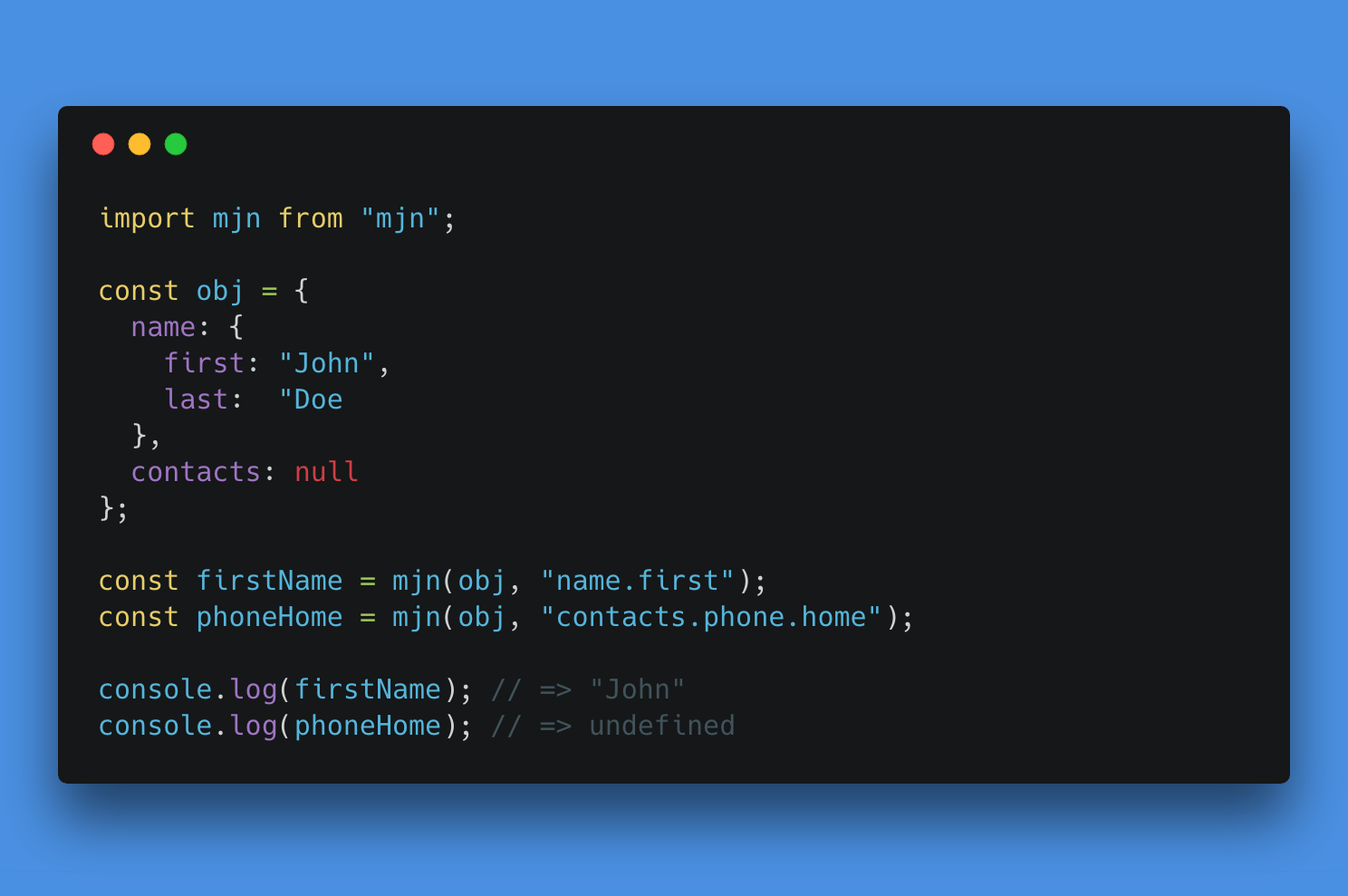
No
cannot get property x of undefined
. Just returnsvoid 0
(undefined) if a value or a key does not exist. Highly inspired from Java's Optional Type.
Index
Installation
yarn
yarn add mjn
npm
npm install --save mjn
cdn
Usage
Simple Example
; // Or import the library as you wish using npm or CDN script tag! const myObject = user: name: "John" surname: "Doe" birthday: "1995-01-29" contacts: email: "foo@bar.com" phone: "000 0000000" languages: "english" "italian" ; const a = ;const b = ;const c = ;const d = ;const e = ; console; // => Johnconsole; // => italianconsole; // => won't log anything!console; // => "no value!"console; // => "I can be a function!"
Real World React Example
import React from "react";import ReactDOM from "react-dom";import maybe from "mjn"; const user = name: first_name: "John" last_name: "Doe" contacts: phone: "+00 000 0000000" email: "john@doe.do" ; const App = <div ="App"> <h1>Hello !</h1> <h2> </h2> <p> </p> </div>; const rootElement = document;ReactDOM;
Demos
React.js
Vue.js
Vanilla JS
Contributors ✨
Thanks goes to these wonderful people (emoji key):
Michele Riva 💻 💼 |
Paolo Roth 💻 |
Mattia Astorino 🎨 |
This project follows the all-contributors specification. Contributions of any kind welcome!