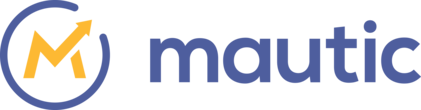
npm install mautic --save
Unofficial Node.js bindings to the Mautic REST API - https://developer.mautic.org/#rest-api
Built with ❤ by FieldControl and contributors - We are hiring
Status
❗️ | WIP: Working in progress and we need you, pull requests are welcome. |
---|
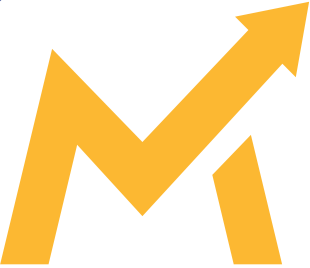
Installation
This client is intended for server side use only.
npm install mautic --save
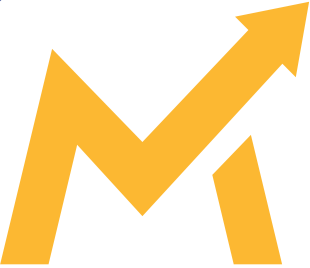
Playground
You can play and watch everything working at bin/playground.js:
node bin/playground.js
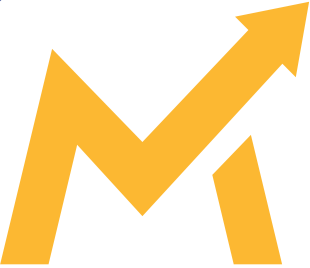
Usage
const Mautic = const client = baseUrl: 'http://mautic-instance/api' auth: username: 'luiz' password: 'shhhhhhhhhhhhhh!:x'
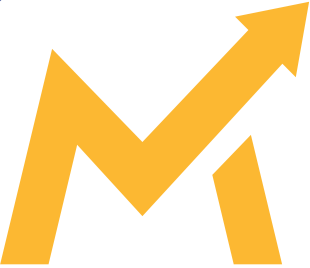
Resources
Contact
// Get contact by idconst response = await clientcontacts
// List contactsconst response = await clientcontacts
// List contacts with a searchconst response = await clientcontacts
// Create contactconst response = await clientcontacts
// Delete contactconst response = await clientcontacts
// Edit contactconst response = await clientcontacts
// If the given id exists, clear all contact info and push with new ones, if not, create a contactconst response = await clientcontacts
// Add points to a contactconst response = await clientcontacts
// Remove points of a contactconst response = await clientcontacts
// Get notesconst response = await clientcontacts
// Get Activity Eventsconst response = await clientcontacts
// Get Companies of a contactconst response = await clientcontacts
// Add UTM tagsconst response = await clientcontacts
// Remove UTM tagconst response = await clientcontacts
// List segments of a contactconst response = await clientcontacts
Segment
// Add contact to segmentconst response = await clientsegments // segmentId, contactId
// Remove contact of segmentconst response = await clientsegments // segmentId, contactId
Company
// Get company by idconst response = await clientcompanies // companyId
// List companiesconst response = await clientcompanies
// Create a companyconst response = await clientcompanies // company
// Edit a companyconst response = await clientcompanies // companyId, company
// If the given id exists, clear all company info and push with new ones, if not, create a companyconst response = await clientcompanies // companyId, company
// Delete a companyconst response = await clientcompanies // companyId
// Add a contact to a companyconst response = await clientcompanies // companyId, contactId
// Remove a contact of a companyconst response = await clientcompanies // companyId, contactId
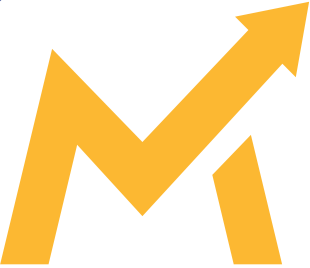
Pull Requests
- Add tests! Your patch won't be accepted if it doesn't have tests.
- Document any change in behaviour. Make sure the README and any other relevant documentation are kept up-to-date.
- Create topic branches. Don't ask us to pull from your master branch.
- One pull request per feature. If you want to do more than one thing, send multiple pull requests.
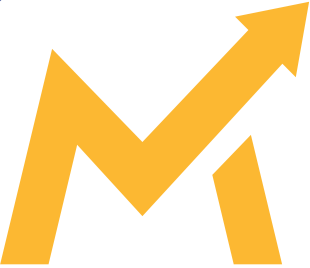
License
This project is licensed under the MIT License - see the LICENSE file for details.
Open source, from Field Control with ❤ - We are hiring!