NOTE: This README.md file temporarily placeholdes the repo, please be patient for the author to document Luna and refer to the code below at the meanwhile.
Luna 🌙 ― A High-Level Programming language.
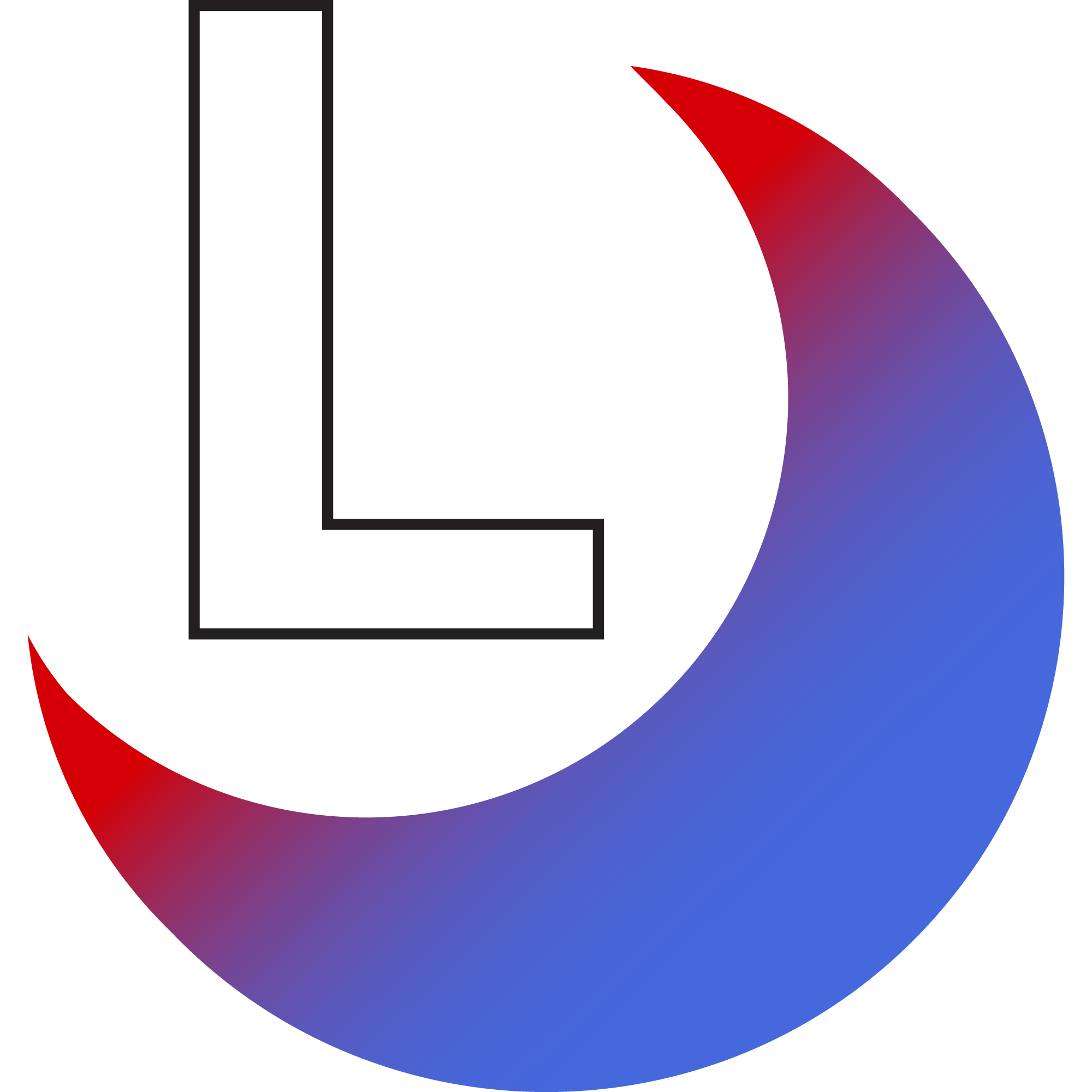
Luna
Luna: An elegant, versatile programming language with efficient scripting capabilities, built in TypeScript for simplicity and productivity in both general programming and automation tasks 🤖.
Get Releases · View Examples · Report Bug · Request Feature
Table of Contents
• 📰 News
- ✅ Added support for asyncronous
lambda
expressions. - ✅ Added "use" statements:
use(external as custom) from 'file.lnx'
- ✅ Improved speed & performance ⚡.
- ✅ Added
embed
statements, you can now embed code from other files inside your main file:embed "path/to/file.ln";
- ✅ Added TapStatements, to execute files without the need to import (performance optimisation):
tap "exec/this/code.ln";
• 🔍 About The Project
Luna is a high-level programming language. It is designed to provide an elegant and productive coding experience for developers. Luna offers a clean and intuitive syntax, making it easier to write efficient and readable code. The language supports a variety of programming paradigms, including procedural, object-oriented, and functional programming styles. Luna is built with a focus on simplicity and productivity, aiming to streamline the development process and enhance developer experience. It provides powerful abstractions and built-in libraries to facilitate common programming tasks.
Luna is an interpreted programming language, which means that code written in Luna is executed directly by an interpreter rather than being compiled into machine code. Here's a high-level overview of how Luna works:
-
Parsing: When you write Luna code, the interpreter first parses it to understand its structure and syntax. This involves breaking the code into tokens and building an abstract syntax tree (AST) representation.
-
Semantic Analysis: After parsing, Luna performs semantic analysis. This phase involves analyzing the AST to enforce language rules and perform type checking. The interpreter verifies that the code follows the correct usage of variables, functions, and types, ensuring its correctness.
-
Execution: Once the code passes semantic analysis, the Luna interpreter begins executing the code line by line. It evaluates expressions, assigns values to variables, and executes control flow statements such as conditionals and loops. During execution, Luna can interact with input and output streams, read from files, and perform other operations as needed.
-
Runtime Environment: Luna provides a runtime environment that includes built-in functions, libraries, and data structures. Developers can utilize these features to perform common tasks without having to implement them from scratch.
Luna's interpreter handles the execution of code dynamically, allowing for flexibility and quick development cycles. This dynamic nature makes it suitable for scripting tasks and rapid prototyping. It also supports concurrency and asynchronous programming using mechanisms like coroutines or event-driven programming.
Overall, Luna aims to provide an elegant and productive programming experience, enabling developers to write clean and expressive code while leveraging the power of the underlying interpreter to execute their programs efficiently.
• 🤔 Why Luna?
Most developers choose us because of the Following Reasons:
- Luna offers a clean and intuitive syntax that promotes readability and ease of understanding.
- The language prioritizes simplicity and productivity, allowing developers to write code more efficiently.
- Luna provides powerful abstractions and built-in libraries, reducing the need for extensive boilerplate code.
- It supports multiple programming paradigms, including procedural, object-oriented, and functional programming.
- Luna's focus on elegance and flexibility makes it an excellent choice for building a wide range of applications, from web development to system programming.
• 🤖 How does Luna 🌙 function?
Luna's functioning follows a precise sequence: first, the written code undergoes tokenization, breaking it into individual tokens. These tokens are then parsed to form statements, which are organized into an Abstract Syntax Tree (AST). This AST is then preprocessed, embedding any inline code fragments. The interpreter then steps in, evaluating the AST and executing the embedded code, resulting in a final result object known as a Runtime Value. This sequential process ensures that the code's logic is accurately understood, processed, and executed, producing the desired outcomes in Luna's unique programming environment.
Red flags 🚩:
Luna is 7.28x slower than Python (exactly 7.28060546875x)
Relation between the runtime of Luna $(t_{l})$ and the runtime of Python $(t_{p})$ and the Luna-Python Time Scalar $(\Delta_{l,p} \approx 7.28060546875)$
Like so: $t_{p}(t_{l}) = \Delta_{l,p} * t_{l}$
Luna has a max of recursive function call of $n = 2428 \pm \Delta R, \ \ \Delta R \in \mathbb{Z}, \ \ |\Delta R| \approx 1$
UPDATE 🔥: When Compiled, Luna gets 20x faster than Python ⚡!
🛠️ Install the Luna API 🌙 : • 🛠️ Getting Started
luna:~$ npm i -g lunascript # Installation (API & CLI)...
luna:~$ luna --help # Show Help Menu...
import { Luna, ... } from 'lunascript';
let script = `
name = input("What's your name? ");
print("Hello, {name}!")
`;
let luna = new Luna();
let output = luna.run(lunascript);
console.log(output); // object {RuntimeValue}
• 🕹️ Code Examples
✱ Functions in Luna:
fn read book {
print("Reading {book}...")
}
read("Luna Docs") # Reading Luna Docs...
✱ Functions with default values:
fn sum x=(1) y=(1) {
if isdef x && isdef y: x + y
else null
}
print(sum(2, 4)) # 6
✱ Constant Definition:
# Defining a constant
pi: const = 3.141
fn area r {
pi * r ** 2
}
print(area(2))
✱ Reactivity in Luna:
# Reactivity
a = "foo"
b: react<a> = lambda {
a.replace("o", '')
}
print(b) # "f"
a = "Hellooo" # // Reactivity... b's function got triggered
print(b) # "Hell"
✱ Anonymous functions Assignment:
# User defined function:
fn sum x y {
x + y
}
# Function assigned to variable
sum = fn sum x y {
x + y
}
# Anonymous function:
a = lambda x y {
x + y
}
✱ Actions in Luna 🌙
# Note:
a = "foo"
# is same as:
a: var = "foo"
# Those are called Actions, and the variable's action is "var" by default...
# Constant:
a: const = 2.718
a = 2 # NameError: Assignment to constant variable 'a'.
✱ Use-case of Luna's Reactivity feature:
# Reactivity
x = 1
doubled: react<x> = x * 2
x = 2
print(doubled) // Output: `4`
✱ Export variables & functions:
// Export function:
out fn sum x y {
^^^
return x + y
}
// Export variable (using the out action):
x: out = 5
^^^
✱ Importing variables & functions:
// Import a module:
use "maths.lnx" as math;
// Import specific components:
use (cos, sin as Sinus, tan, PI as pi) from "math.lnx"; // Modules have extension ".lnx"...
// Call a file without importing it:
tap "file.ln";
// Embedding a file into the current code (code-preprocessor):
embed "file.ln";
✱ Implementing Math in Luna:
$$\Huge e^x = \sum_{n=0}^{\infty} \frac{x^n}{n!}$$
# The Maclaurin series of the exponential function e^x:
use (fact as factorial) from "factorial.lnx"
fn expo x {
precision = 100
result = 0
n = 0
while n < precision {
result += x**n / factorial(n)
n += 1
}
result
}
print(expo(0)) # 1
✱ Implementing Luna's reactivity with Math:
$$\Huge \psi(\theta) = \frac{e^{2\theta}}{log(4\theta)} + \theta^2 + 2\theta!$$
use (E as e, factorial, Log as log) from "math.lnx";
theta = 1
psi_theta: react<theta> = (e ** 2 * theta) / log(4 * theta) + theta**2 + factorial(2 * theta)
print(psi_theta) // Output: `15.2729565249...`
theta = 2
print(psi_theta) // Output: `68.4570428413...`
📖 Documentation
You can check Luna documentation here (GitHub).
📚 API
You can check Luna Application Programming Interface (API) here (GitHub).
📜 Licence (MIT)
Copyright (c) 2022 Luna (https://www.github.com/rhpo/luna) Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
👋 Contact:
• Email ― luna@ramey.ml
• Phone Number ― +213 553 23 84 10
• Discord ― (Luna ― High-level programming language)
👤 About the author:
• Name ― Ramy Hadid.
• Age ― 18 years old.
• Nationality ― 🇩🇿 Algeria.
• Linkedin ― (Ramy Hadid)
• Instagram ― @ramyhadid
• Discord ― ramy#1539
• GitHub ― @rhpo
• Email (personal) ― me@ramey.ml
• Programming Languages ― C# • Ruby • TypeScript • Python • LunaScript.
Written by @rhpo with ❤️.