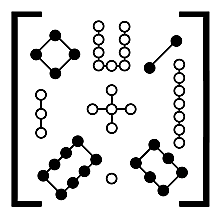
Loshu.js
Linear Algebra for Javascript
Online REPL | API Reference
Loshu.js is a linear algebra library for JavaScript. It not only provides convienient manipulation of matrices and vectors, but is also capable of more advanced operations such as matrix decompositions, eigenvalue and vectors, solution and approximation to linear systems, and many more!
(Optionally) accelerated using WebAssembly.
Features
- Basic matrix and vector math: multiplication, inverse, norm, ...
- Matrix factorizations: QR, SVD, Polar, LDL, Cholesky, LUP, ...
- Solving linear systems: Gauss-Jordan elimination, Least squares approximation, ...
- Miscellaneous utilities: affine transformation, n-dimensional point/line/plane distance, ...
- Pretty print matrices
- Simple to use, documented interface
- Try it out in your browser with the online REPL
- Additional WebAssembly implementation for certain matrix math (C++ → emscripten) for up to 100x speed boost
Quick Start
For Browser
To use the library, simply include the following to your html:
To enable Web Assembly acceleration of some functionalities such as eigen()
, inv()
and svd()
, include the loshuwasm.js
script before loshu.js
, like so:
You will see a web assembly backend initialized
message in console if the wasm module is detected. Otherwise you can manually turn it on using lo.options.usewasm=true
.
You can also download the scripts from the links above and host them yourself, but you'll also need to download here the .wasm
binary and put it in the same folder.
For Node.js
To use the library with node.js, download and require
it like so:
const lo =
To enable Web Assembly acceleration of some functionalities such as eigen()
, inv()
and svd()
, Download the wasm binary
as well as the wrapper and put them under the same folder. You will see a web assembly backend initialized
message in the console.
Examples
let A = 123456789; // matrix is just JS array (row major)let B = lo; // generate a 3x3 matrix of random valueslet C = lo // array multiplication let USV = lo; // singular value decompositionlo; // pretty print the matrices let l = lo // fit a line to data pointslo; // display the line equation let M = lo; // create a 45° rotation matrix around y axislet u = 123; // some vectorlet v = lo; // apply the transform
Function List
References
The development of this library is largely inspired by the textbook, David Poole's Linear Algebra: A Modern Introduction.
Besides Wikipedia and Wolfram MathWorld, the following online resources have also been very helpful in explaining algorithms.
- http://pi.math.cornell.edu/~web6140/TopTenAlgorithms/QRalgorithm.html
- https://www.mathworks.com/matlabcentral/fileexchange/38303-linear-algebra-package
- http://buzzard.ups.edu/courses/2014spring/420projects/math420-UPS-spring-2014-buffington-polar-decomposition.pdf
- http://webhome.auburn.edu/~tamtiny/lecture 10.pdf
- https://www.cs.cmu.edu/~venkatg/teaching/CStheory-infoage/book-chapter-4.pdf
- http://math.mit.edu/~gs/linearalgebra/ila0403.pdf