[WIP] Got + Ky => Kot
Kot is isomorphic HTTP client based on Ky for the browser and Got for Node.js.
Install
$ npm install kot
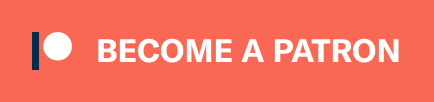
Usage
; async { console; //=> `{data: '🐈'}`};
API
kot(input, [options])
Returns a Response
object with Body
methods added for convenience. So you can, for example, call kot.json()
directly on the Response
without having to await it first. Unlike the Body
methods of window.Fetch
; these will throw an HTTPError
if the response status is not in the range 200...299
.
kot.get(input, [options])
kot.post(input, [options])
kot.put(input, [options])
kot.patch(input, [options])
kot.head(input, [options])
kot.delete(input, [options])
Sets options.method
to the method name and makes a request.
input
Type: string
URL
The URL to fetch. Must be an absolute URL.
options
Type: Object
json
Type: Object
Shortcut for sending JSON. Use this instead of the body
option. Accepts a plain object which will be JSON.stringify()
'd and the correct header will be set for you.
searchParams
Type: string
Object<string, string|number>
URLSearchParams
Default: ''
Search parameters to include in the request URL. Setting this will override all existing search parameters in the input URL.
prefixUrl
Type: string
URL
When specified, prefixUrl
will be prepended to input
. The prefix can be any valid URL, either relative or absolute. A trailing slash /
is optional, one will be added automatically, if needed, when joining prefixUrl
and input
. The input
argument cannot start with a /
when using this option.
Useful when used with kot.extend()
to create niche-specific Kot-instances.
; // On https://example.com async { await ; //=> 'https://example.com/api/unicorn' await ; //=> 'https://cats.com/unicorn'};
retry
Type: number
Default: 2
Retry failed requests made with one of the below methods that result in a network error or one of the below status codes.
Methods: GET
PUT
HEAD
DELETE
OPTIONS
TRACE
Status codes: 408
413
429
500
502
503
504
It adheres to the Retry-After
response header.
timeout
Type: number
Default: 10000
Timeout in milliseconds for getting a response.
throwHttpErrors
Type: boolean
Default: true
Throw a HTTPError
for error responses (non-2xx status codes).
Setting this to false
may be useful if you are checking for resource availability and are expecting error responses.
kot.extend(defaultOptions)
Create a new kot
instance with some defaults overridden with your own.
; // On https://my-site.com const api = kot; async { await api; //=> 'https://example.com/api/users/123' await api; //=> 'https://my-site.com/status'};
defaultOptions
Type: Object
kot.HTTPError
Exposed for instanceof
checks. The error has a response
property with the Response
object.
kot.TimeoutError
The error thrown when the request times out.
Compatibility
Node.js 8 or the latest version of Chrome, Firefox, and Safari.
Related
- got - Simplified HTTP requests for Node.js
- ky - Tiny and elegant HTTP client based on the browser Fetch API
Maintainers
License
MIT