Foglet-core
Easy use of WebRTC Networks with embedded network management and simple communication primitives.
Read the documentation or read the API Documentation
Features
Communication primitives:
- Broadcast (to all peers in your network, with an anti-entropy mechanism not enabled by default). We ensure single delivery and a causal relation between 2 consecutive messages from a same site.
- Unicast (to one direct neighbor)
- Multicast (to one or several direct neighbors)
- Streaming over our Broadcast and Unicast
- Multiple communication channel per network
We only support Data Channel for the moment.
- Experimental Media Unicast and Broadcast
Warning: unicast media is working only for a period of time defined by the delta parameter in the RPS
Network management:
- An adapter on ran3d/spray-wrtc as Random Peer Sampling Network (keeping log(NetworkSize) peers around you)
- An adapter on Cyclon as Random Peer Sampling Network (keeping "maxPeers" peers around you)
- Overlay Networks or Networks can be created with: ran3d/n2n-overlay-wrtc
- Disable WebRTC for testing purposes (or simulation) by using our Simple-peer moc.
Installation
Prerequisite: a browser compatible with WebRTC
npm install --save foglet-core
The foglet library is distributed with its sources and a bundle for an in-browser usage.
Getting started
Creates a new HTML file and insert the foglet bundle in it:
<!-- or use the minified bundle, foglet.bundle.min.js -->
Then, foglet library is available in the variable foglet
:
const Foglet = fogletFoglet;
If you do not provide a list of ice servers, your example will work in localhost but not on the Web.
To be begin with, let's write a simple piece of JS code:
Then, open the HTML file and look into the developpers console. You should see that your foglet has been connected to the RPS.
Or for the fast version:
git clone https://github.com/RAN3D/foglet-core.gitcd foglet-corenpm installnpm run build
-
Open tests/examples/example.html in a browser supporting WebRTC and the devConsole
-
Try to play with
testunicast()
andtestbroadcast()
or try the signaling example using a signaling server:
- Just run a simple http server with an embedded signaling server serving the tests/examples/example-signaling.html:
npm run example
- open http://localhost:8000/signaling
Signaling server
In order to run this library, you have to provide the address of a signaling server using the signaling.address
option and a signaling.room
in order to create a private network. This server will be used to establish the first connection between the new peer and the the network.
This server must be compatible with the foglet library. The library foglet-signaling-server provides an example implementation of such signaling server.
Developpment
We offer another library which lets you to build/test/run your own application with a signaling server: https://github.com/ran3d/foglet-scripts.git
# Clone and install git clone https://github.com/ran3d/foglet-core.gitnpm install # Build the bundle (webpack stack) npm run build # Lint using [standard](https://standardjs.com/) npm run lint # Mocha, chai stack with a simple-peer-moc for mocking webrtc features npm run test # Run a server serving examples and a signaling server on http://localhost:8000/ npm run example
Contributors:
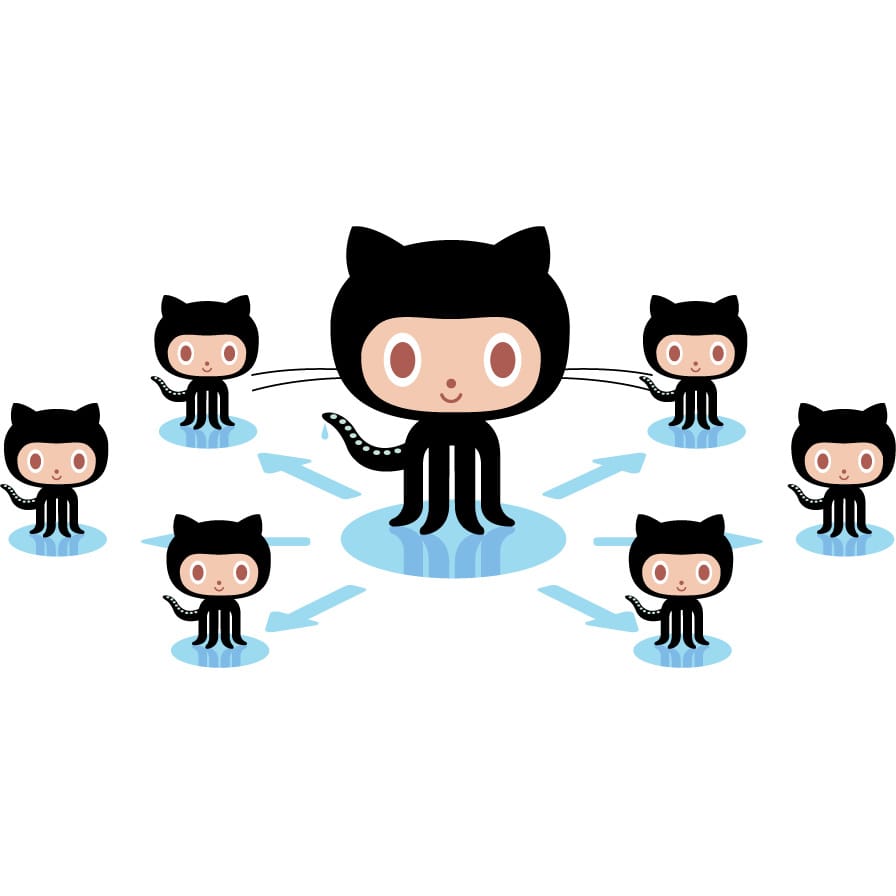
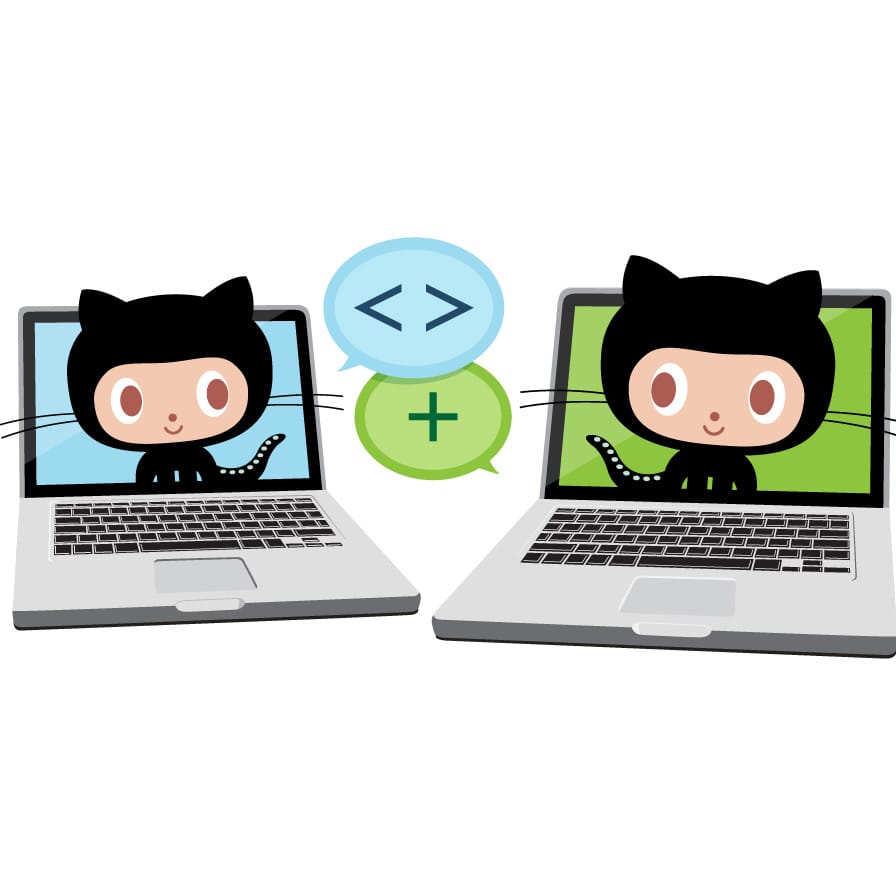
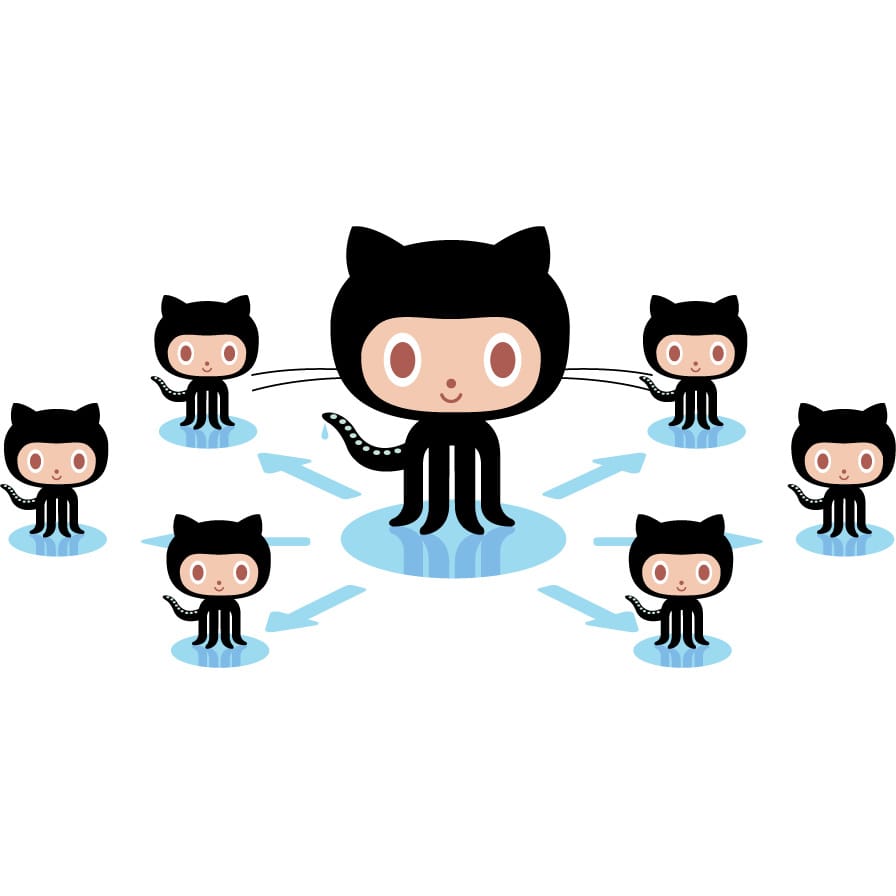