featureflow-angular

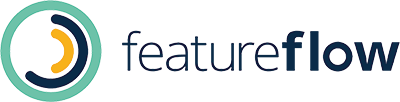
Featureflow Angular Service
Get your Featureflow account at featureflow.io
Installation
Using NPM
$ npm install --save featureflow-angular
Get Started
-
add FeatureflowAngularModule
to app NgModule
.
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { FeatureflowAngularModule } from 'featureflow-angular';
@NgModule({
declarations: [
],
imports: [
BrowserModule,
FeatureflowAngularModule
],
providers: [],
})
class MainModule {}
Use
import { Component } from '@angular/core';
import { FeatureflowAngularService } from 'featureflow-angular';
@Component({
template: `
<div *ngIf="featureflowService.evaluate('my-feature-key').isOn()">
<h2>I will be seen when the feature is on</h2>
<p>And this is some extra text</p>
</div>
<div *ngFor="let item of features | keyvalue">
{{item.key}}:{{item.value}}
</div>`
})
export class YourComponent {
features: any;
featureflow: any;
constructor(
private featureflowService: FeatureflowAngularService
) {
featureflowService.init(API_KEY, {id: 'uniqueUserId1'}, null);
this.features = featureflowService.getFeatures();
this.featureflow = featureflowService.client();
}
}
-
That's it.
-
If you want to update your component when the evaluated feature changes in realtime,
us featureChanged$ subscription from featureflowService
import { ChangeDetectionStrategy, ChangeDetectorRef, Component, OnDestroy } from '@angular/core';
import { Subscription } from 'rxjs';
@Component({
changeDetection: ChangeDetectionStrategy.OnPush
})
export class YourComponent {
features: any;
featureflow: any;
updateFeatures: Subscription = null;
constructor(
private ref: ChangeDetectorRef
) {
this.updateFeatures = this.featureflowService.featureChanged$.subscribe(features => {
this.features = featureflowService.getFeatures();
this.ref.detectChanges();
});
}
ngOnDestroy() {
this.updateFeatures.unsubscribe();
}
}
Example
Update the SDK key to match your JS Client Environment SDK Key in featureflow-angular-examaple/src/app/app.component.ts
featureflowService.init('js-env-YOUR-KEY-HER', {id: '1'}, null);
Start the example project
cd projects/featureflow-angular-example
ng serve
Try toggling features in the featureflow dashboard for your project and environment.
Developing
This project consists of 2 modules:
- /projects/featureflow-angular - the angular service library
- /projects/featureflow-angular-example - the example implementation
to build the library, from the root directory run
ng build featureflow-angular
then run the example
cd projects/featureflow-angular-example
ng serve
Publishing
npm run package
npm publish ./dist/featureflow-angular/featureflow-angular-xx.xx.xx.tgz
Running unit tests
Run ng test featureflow-angular
to execute the unit tests via Karma.