facemorph
Create animated GIFs that morph faces to each other in Node.js.
I wanted a simple way to take a set of pictures we take periodically of our newborn son and watch as he changed over time. All the potential solutions I found to date required a GUI to identify facial landmarks and/or the morphing didn't work that well, so I decided to combine the separate pieces of each step to create a relatively good face morphing into a single library with a dead simple API.
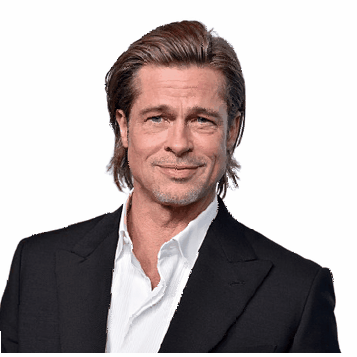
Assumptions/Limitations
- Each image in the created GIF must contain exactly one face. If no faces or more than one are detected, that picture is ignored in the output GIF.
- A minimum of two images are required to be provided in
createGif
(see below). - Faces are detected using the model provided in face-api.js. Human faces work best, where animal faces might or might not work.
- For best results, use images with white (or consistent) backgrounds.
Install
$ npm install facemorph
Simple Usage
API
const facemorph = const gifBuffer = await facemorphawait fspromises
CLI
$ node node_modules/facemorph/dist/tasks/createGif -i path/to/img1.jpg -i path/to/img2.png
Your new gif: /path/to/output/facemorph_#############.gif
API
constructor
// `frames: number = 20` is the number of frames between each face morph in the GIFconst facemorph =
createGif
Create a GIF with morphed faces between all provided images.
// images: MorfImages[] - Array of at least 2 images that can be a string, Readable stream, or raw Buffer// delayMs: number = 20 - The delay in milliseconds between each frame// repeat: number = 0 - Whether to repeat the GIF animation or not. -1: no repeat, 0 repeatawait facemorph // : Promise<Buffer>
createFrames
Create an array of Buffers that are images of all the frames to be used
to create an animated GIF of morphed faces. This is used inside of createGif
,
but could be used as a standalone if you want to take all the frames and do what
you'd like with them.
// images: MorfImages[] - Array of at least 2 images that can be a string, Readable stream, or raw Bufferawait facemorph // : Promise<Buffer[][]>
setFrames
Reset the number of frames between each face morph.
facemorph // : number