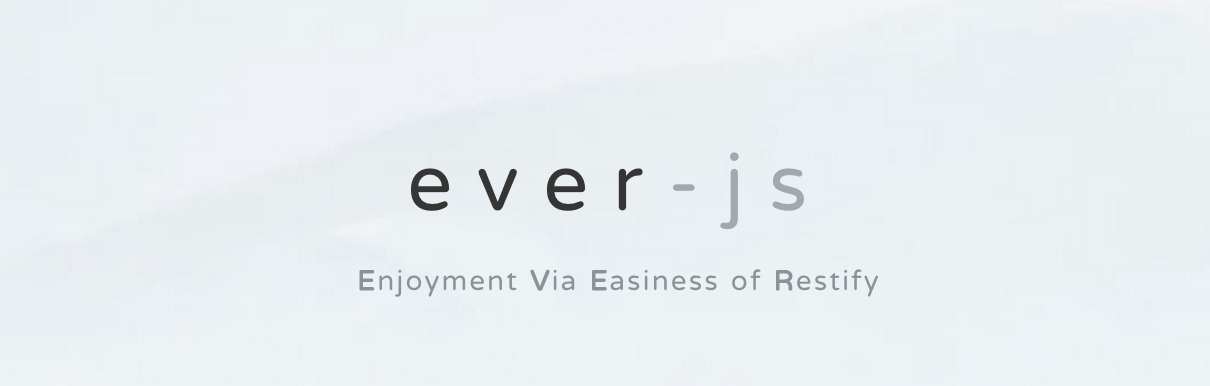

Enjoyment Via Easiness of Restify - js
Restify.
This framework is completely based on- Installing the ever-js
- Folder structure
- Global Variables
- Handlers
- Routes
- Library
- Configuration
- User Filters
- User Middleware
- Kernel
- main.js
- package.json
Installing the framework
Please note that, -g is required to use.
npm install -g ever-js
Building the first app
Follow the below mentioned steps to create the first application.
mkdir MyFirstAppcd MyFirstApp/
Then run following command to initialise framework.
everjs init
Folder structure
MyFirstApp/ |-routes/ |--Routes.js |-lib/ |--SampleLib.js |-configuration/ |-global/ |--common.json |--default.json |--production.json |--RestifyConfig.js |-handlers/ |--MongoHandler.js |-filters/ |--SampleFilters.js |-middleware/ |--SampleMw.js |-kernel/ |--Banner.js |--Kernel.js |--Server.js |--main.js |--package.json
Global Variables
Variables | Usage |
---|---|
_ | This is Lodash module. eg: _.forEach |
GlobalConfig | Configureation values based on the environment(default.json or production.json) and common.json To access environment based setting values. GlobalConfig.[setting value] eg : GlobalConfig.db.mongo To access Common Values in commong.js GlobalConfig.Common.[setting value] eg: GlobalConfig.Common.db_handlers |
Dbh | Contains the Database handlers. Eg: Dbh.Mongo |
Lib | Contains modules defined in lib/ folder. Eg: Lib.SampleLib.sampleFunction(); (not avaialbe to use inside lib/ ) |
handlers/
This contains all the handler modules.
MongoHandler.js
This handler manage Mongo Database connections.
Usage of Mongo Hanlder.
{ DbhMongo;}
routes/
This contains all the routing information. These settings are stored in Routes.js
Structure of the Routes.js as follows.
moduleexports = { var userFilters = dependenciesUserFilters; var userMiddleware = dependenciesuserMiddleWare; return GET: POST: PUT: DELETE: }
-
GET holds all the routings uses http GET method
-
POST holds all the routing uses http POST method
-
PUT holds all the routing uses http PUT method
-
DELETE holds all the routing uses http DELETE method
-
Adding a new route Following is the syntax for adding a route.
HTTP_METHOD : config: path: 'Patter of the route' isSecure: true | false method: userMiddlewareMiddleware NameMethod Name filters: filter 1 filter 2 ...
- config
-
path - Pattern of the route
-
isSecure - If set true, a authentication function must be set in the config section as mentioned below section.
-
method - Holds middleware to execute on particular pattern
-
filters - Executes before the Middleware defined in the method section.
The sample Route file is as follows.
moduleexports = { var userFilters = dependenciesUserFilters; var userMiddleware = dependenciesuserMiddleWare; return GET: config: path: '/get-sample-route-no-filter' isSecure: false method: userMiddlewareSampleMwgetWithoutFilters filters: config: path: '/get-sample-route-with-filter' isSecure: true method: userMiddlewareSampleMwgetWithFilters filters: userFiltersSampleFilterssampleFilter POST: PUT: DELETE: }
lib/
Node modules added to this folder can be access via User Middleware and filters.
Syntax for accessing the Lib files.
LibModule file name
Example:
sampleFunction() in SampleLib.js module accessible as follows.
LibSampleLib;
configuration//
This folder contains the main config file RestifyConfig.js
- AuthFunction section
{
In this section, if you do not have any authentication filter, use as follows.
return false;
Else, follow below mentioned syntax,
return dependenciesfiltersFilter nameAuthentication
example:
return dependenciesfiltersSampleFilterssampleAuthFilter;
- AppConfig section
This section is dedicate to Restify Create Server paramters
{
You can add create server parameters as follows,
certificate: ... key: ...
This section has the
- Application name retrieves from package.json
- Server Port, which is default to 8312 or can be set using an environment variable : RESTIFY_PORT
- Listen address, which is to all the connections or can be set using an environment variable: RESTIFY_ LISTEN_IP
name: dependenciespackageJsonname port : 8312 || processenvRESTIFY_PORT address : "0.0.0.0" || processenvRESTIFY_LISTEN_IP
- RestifyMiddlewareSet section
This section is used to load the Restify plugins
{
Following plugins are loaded as default settings
dependenciesrestifyObject dependenciesrestifyObject dependenciesrestifyObject dependenciesrestifyObject
- RestifyPre section
This section is used to load Restify's pre() modules.
{
Use the following syntax to load the load the modules.
dependenciesrestifyObjectpre filter|pre
Example :
return dependenciesrestifyObjectpre dependenciesrestifyObject ;
configuration/global/
Contains all the configuration values based environment. If NODE_ENV is NOT defined, default.json is loaded. for Production configuration values.
export NODE_ENV=production
File name | Descriptions | Environment |
---|---|---|
default.json | contains none production environment values. |
default environment. |
production.json | contains production enviroment values. | Production |
common.json | Contains common values for both the enviroments | All |
default.json
production.json
common.json
db_handlers contains the information of each Database handler. libPath must be defined relative to the kernel/
filters/
Contains the functionality which executes prior to assigned user middleware.
Node modules added to this folder can be access from Routes.js.
Format of the filter function is very important !!!
Please follow below mentioned format for filter function.
{ //Your logic goes here ;}
sample filter :
{ console; ;}
Syntax for accessing the filter files from Routes.js.
userFiltersFilter file namefilter
Example:
sampleFilter() in SampleFilters.js module accessible as follows.
userFiltersSampleFilters;
middleware/
Contains the functionality which executes based on the route patterns.
Node modules added to this folder can be access from Routes.js.
Format of the Middleware function is very important !!!
Please follow below mentioned format for filter function.
{ //Your logic goes here}
sample Middleware :
{ res;}
Syntax for accessing the filter files from Routes.js.
userMiddlewareMiddleware file nameMiddleware
Example:
getWithoutFilters() in SampleMw.js module accessible as follows.
userFiltersSampleMwgetWithoutFilters;
kernel/
Contains the heart of the framework. Try not to edit this.
But, be my guest to suggest any modification.
- Kernel.js - Loads all the dependencies and pass them to modules.
- Server.js - This is where Restify server loads all the routes, lib, middleware, filter and configs.
- Banner.js - Just prints my logo :)
main.js
Executes the kernel of the framework. Not much to see.
package.json
Default package.json for the current application. You can add any dependencies which required. But, please do not remove any existing ones.