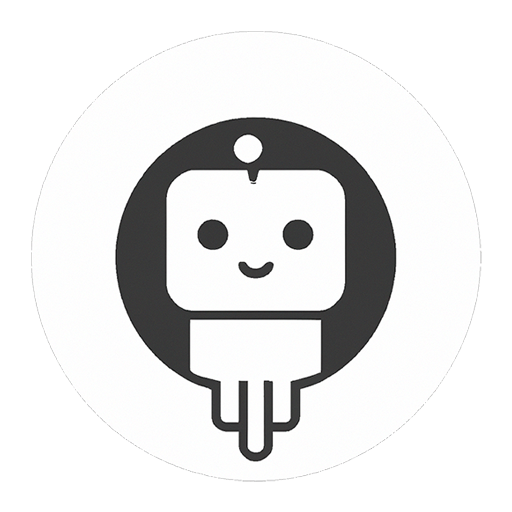
Discord User Control Library
Automate your discord account however you so choose
View Example
·
Report Bug
·
Request Feature
Table of Contents
About The Project
Automating discord is cumbersome when all the mature options seem to restrict access to user accounts. This prevents so many cool things from being able to work and I often found myself wanting to create these things so I decided to throw together a library that is fast and robust (hopefully).
Here are some use cases:
- Auto responding to messages
- Exporting your DM or guild messages.
- Leaving all your guilds
- Copying data you typically don't see
- Downloading media
- Auto filter your chat messages
The project is still fairly young so it's missing some vital features but for most it should suffice. If you need a particular feature implemented just create a feature request in the issues section.
Getting Started
To start using discord-self.js you will need to install it via npm or yarn. You will also need access to your discord token -> One of the methods
- npm
npm install discord-self.js
- yarn
yarn add discord-self.js
Usage and Examples
Using discord-self.js is simple, just provide it your token, initialize and login. After that, for most features, it won't matter if the websocket disconnects or not. Just request away.
- Login and retrieve all DM messages (for exporting for example)
import { Discord } from 'discord-self.js';
(async () => {
const discord = new Discord("DISCORD-TOKEN-HERE");
// Login
await discord.init();
if (!await discord.login()) throw new Error("Cannot login");
console.log("Logged in as " + discord.userTag);
// Get all DM channels
const channels = await discord.getDMChannels();
console.log(`Found ${channels.length} DM channels`);
// Get all messages from each channel
for (const channel of channels) {
const messages = await discord.getAllMessages(channel.id);
console.log(`Found ${messages.length} messages in channel ${channel.id}`);
}
})();
- Custom Emoji are parsed from content automatically
await discord.sendMessage("897219587714719748", ":balls_emoji:");
- Save all your valuable information
import { Utils, Discord } from 'discord-self.js';
import fs from 'fs';
(async () => {
const discord = new Discord("DISCORD-TOKEN-HERE");
await discord.init();
if (!await discord.login()) throw new Error("Cannot login");
fs.writeFileSync("user.json", Utils.jsonFormat(Object.assign({}, discord.sessionInfo.user, { country_code: discord.sessionInfo.country_code })));
fs.writeFileSync("user_settings.json", Utils.jsonFormat(discord.sessionInfo.user_settings));
fs.writeFileSync("sessions.json", Utils.jsonFormat(discord.sessionInfo.sessions));
fs.writeFileSync("relationships.json", Utils.jsonFormat(discord.sessionInfo.relationships));
fs.writeFileSync("guilds.json", Utils.jsonFormat(discord.sessionInfo.guilds));
fs.writeFileSync("connected_accounts.json", Utils.jsonFormat(discord.sessionInfo.connected_accounts));
fs.writeFileSync("payment_sources.json", Utils.jsonFormat(await discord.getPaymentSources()));
fs.writeFileSync("payments.json", Utils.jsonFormat(await discord.getPayments()));
})();
- Wait for rate limits (this is something that needs a lot of work)
import { Utils } from 'discord-self.js';
// ... etc
// <any> should be the type the function returns (if you want types)
await Utils.waitIfRateLimited<any>(async () => {
return await discord.deleteGuildRole(guild.id!, role.id!);
});
These are just a few examples but you can do most of the things a typical user can do and more.
Roadmap
Although I say it can do most things. There is still much to do
- Add testing to keep things professional
- Implement voice connection and streaming
- Implement some method for gracefully handling rate limits
- Cover most of the REST API
- Possibly wrap the event emitter up and add type information instead of raw websocket events
- Lazy guild loading. Super easy just need to find the time
Find somethings missing and it's not here? Open an issue and let me know what could be improved.
Contributing
Any contributions you can make are super appreciated.
If you have a suggestion that would make this better, please fork the repo and create a pull request. You can also simply open an issue with the tag "enhancement". Don't forget to give the project a star! Mwah!
- Fork the Project
- Create your Feature Branch (
git checkout -b feature/AmazingFeature
) - Commit your Changes (
git commit -m 'Add some AmazingFeature'
) - Push to the Branch (
git push origin feature/AmazingFeature
) - Open a Pull Request
License
Distributed under the MIT License. See LICENSE for more information.