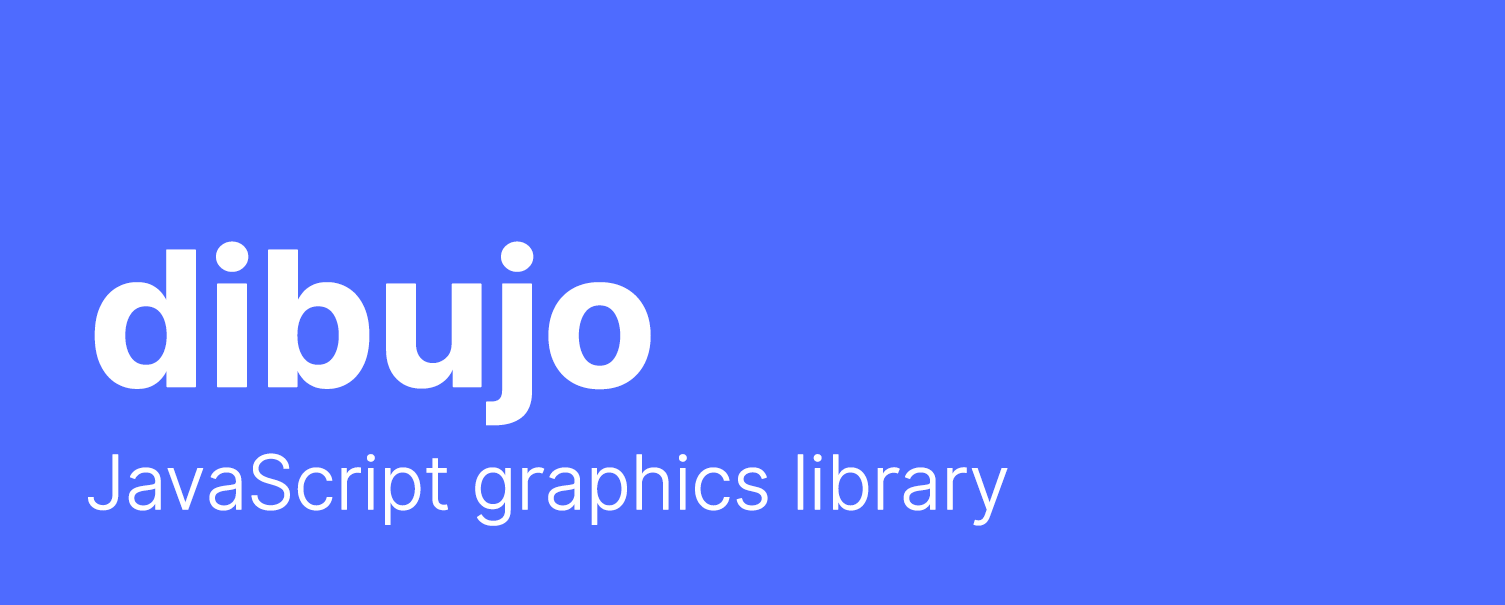
npm install dibujo
To start using dibujo you have to first create a dibujo entity
const render = new dibujo.Render()
after you have created the first dibujo instance you can create a scene but if you don't create a scene and add graphics to dibujo dibujo will use it's default scene to render the graphics you add.
A scene is a group of graphic objects, when you create a scene you can specify the background color
of the scene for example:
const scene = new dibujo.Scene('#FF00FF')
When you create a scene you can add graphics to the scene
const rect = new dibujo.Rect({
position: new dibujo.Vector(10, 10),
width: 100,
height: 100,
color: 'blue'
})
scene.add(rect)
And to see the scene the scene must be rendered so you have to add the scene to render in this way:
There are several kinds of Graphic objects and when you create a new graphic you have to chosse one of them
rigth now there are 7 kinds of diferent graphics:
- Rect
- Circle
- Picture
- Line
- Poligon
- Text
- Arc
const picture = new dibujo.Picture({
position: new dibujo.Vector(10, 10),
width: 100,
height: 100,
src: './apple.png'
})
Param |
Type |
Description |
position |
object |
This object contains the position of the image |
src |
string |
This will be the location where the image is saved |
width |
number |
The width |
opacity |
number |
The opacity |
height |
number |
The height |
const rect = new dibujo.Rect({
position: new dibujo.Vector(10, 10),
width: 100,
height: 100,
color: 'blue'
})
Param |
Type |
Description |
position |
object |
This object contains the position of the rect |
color |
string |
The color of the rect |
width |
number |
The width |
height |
number |
The height |
stroke |
boolean |
Draw stroke |
lineColor |
number |
Stroke color |
lineWidth |
number |
Line width |
const text = new dibujo.Text({
position: new dibujo.Vector(10, 10),
content: 'Hello World'
})
Param |
Type |
Description |
position |
Point |
The coordinates of the text |
content |
string |
The content |
style |
object |
This is the style of the text |
const line = new dibujo.Line({
start: new dibujo.Vector(10, 10),
end: new dibujo.Vector(20, 20),
color: 'blue'
})
Param |
Type |
Description |
start |
Point |
The start coordinate of the line |
end |
Point |
The end coordinate of the line |
color |
string |
This is the color of the line |
const poli = new dibujo.Poligon({
cords: [
{x: 10, y: 10},
{x: 20, y: 20},
{x: 10, y: 20}
],
color: 'blue'
})
Param |
Type |
Description |
cords |
Array |
Array of the vertex of the poligon |
fill |
boolean |
Fill the poligon |
stroke |
boolean |
Stroke the poligon |
strokeColor |
string |
This is the color of the lines of the poligon |
color |
string |
This is the color of the poligon |
const circle = new dibujo.Circle({
position: new dibujo.Vector(10, 10),
radius: 10,
color: 'blue'
})
Param |
Type |
Description |
position |
Point |
Position of the circle |
radius |
number |
Radius of the circle |
color |
string |
Color of the circle |
stroke |
boolean |
Show stroke |
lineWidth |
number |
Width of the line |
lineColor |
string |
Color of the line |
const arc = new dibujo.Arc({
position: new dibujo.Vector(10, 10),
radius: 10,
color: 'blue'
})
Param |
Type |
Description |
color |
string |
Color of the arc |
position |
Point |
Position of the arc |
radius |
number |
Radius of the arc |
lineWidth |
number |
Width of the line |
stroke |
boolean |
Show stroke |
lineColor |
string |
Color of the line |
eAngl |
number |
End angle of the arc |
aAngl |
number |
Start angle of the arc |