Copress
Change current release
upgrade
express 3.x to 4.x
About
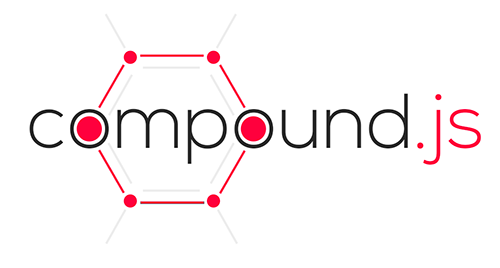
CompoundJS - MVC framework for NodeJS™. It allows you to build web application in minutes.
Compound modules now available at https://github.com/compoundjs
Full documentation is available at http://compoundjs.com/ and using man(1).
Installation
Option 1: npm
sudo npm install copress -g
Option 2: GitHub
sudo npm install taoyuan/copress
Usage
# initialize app compound init blog && cd blognpm install # generate scaffold compound generate crud post title content published:boolean # run server on port 3000 compound s 3000 # visit app open http://localhost:3000/posts
Short functionality review
CLI tool
$ compound help
Usage: compound command [argument(s)]
Commands:
h, help Display usage information
i, init Initialize compound app
g, generate [smth] Generate something awesome
r, routes [filter] Display application routes
c, console Debug console
s, server [port] Run compound server
install [module] Installs a compound module and patches the autoload file
compound init [appname][ option(s)]
options:
--coffee # Default: no coffee by default
--tpl jade|ejs # Default: ejs
--css sass|less|stylus # Default: stylus
--db redis|mongodb|nano|mysql|sqlite3|postgres
# Default: memory
compound generate smth
smth = generator name (controller, model, scaffold, ...can be extended via plugins)
more information about generators available here: http://compoundjs.github.com/generators
compound server 8000
equals to PORT=8000 node server
- run server on port 8000
compound console
run debugging console (see details below)
compound routes
print routes map (see details below)
Directory structure
On initialization directories tree generated, like that:
.
|-- app
| |-- assets
| | |-- coffeescripts
| | | `-- application.coffee
| | `-- stylesheets
| | `-- application.styl
| |-- controllers
| | |-- admin
| | | |-- categories_controller.js
| | | |-- posts_controller.js
| | | `-- tags_controller.js
| | |-- comments_controller.js
| | `-- posts_controller.js
| |-- models
| | |-- category.js
| | |-- post.js
| | `-- tag.js
| |-- tools
| | `-- database.js
| |-- views
| | |-- admin
| | | `-- posts
| | | |-- edit.ejs
| | | |-- index.ejs
| | | |-- new.ejs
| | |-- layouts
| | | `-- application_layout.ejs
| | |-- partials
| | `-- posts
| | |-- index.ejs
| | `-- show.ejs
| `-- helpers
| |-- admin
| | |-- posts_helper.js
| | `-- tags_helper.js
| `-- posts_helper.js
`-- config
|-- database.json
|-- routes.js
|-- tls.cert
`-- tls.key
HTTPS Support
Just place your key and cert into config directory, compound will use it.
Default names for keys are tls.key
and tls.cert
, but you can store in in another place, in that case just pass filenames to createServer function:
server.js
;
Few helpful commands:
# generate private key openssl genrsa -out /tmp/tls.key# generate cert openssl req -new -x509 -key /tmp/tls.key -out /tmp/tls.cert -days 1095 -batch
Routing
Now we do not have to tediously describe REST routes for each resource, enough to write in config/routes.js
code like this:
exports { map;};
instead of:
var ctl = ;app;app;app;app;app;app;app; var com_ctl = ;app;app;app;app;app;app;app;
and you can more finely tune the resources to specify certain actions, middleware, and other. Here are example routes for my blog:
exports { map; map; map; map;};
since version 0.2.0, it is possible to use generic routes:
exports { map; mapall':controller/:action';};
if you have custom_controller
with test
action inside it you can now do:
GET /custom/test
POST /custom/test
GET /custom/test/1 // also sets params.id to 1
for debugging routes described in config/routes.js
you can use compound routes
command:
$ compound routes
GET / posts#index
GET /:id posts#show
sitemap.txt GET /sitemap.txt posts#map
adminPosts GET /admin/posts.:format? admin/posts#index
adminPosts POST /admin/posts.:format? admin/posts#create
newAdminPost GET /admin/posts/new.:format? admin/posts#new
editAdminPost GET /admin/posts/:id/edit.:format? admin/posts#edit
adminPost DELETE /admin/posts/:id.:format? admin/posts#destroy
adminPost PUT /admin/posts/:id.:format? admin/posts#update
likesAdminPost PUT /admin/posts/:id/likes.:format? admin/posts#likes
Filter by method:
$ compound routes GET
GET / posts#index
GET /:id posts#show
sitemap.txt GET /sitemap.txt posts#map
adminPosts GET /admin/posts.:format? admin/posts#index
newAdminPost GET /admin/posts/new.:format? admin/posts#new
editAdminPost GET /admin/posts/:id/edit.:format? admin/posts#edit
Filter by helper name:
$ compound routes Admin
newAdminPost GET /admin/posts/new.:format? admin/posts#new
editAdminPost GET /admin/posts/:id/edit.:format? admin/posts#edit
likesAdminPost PUT /admin/posts/:id/likes.:format? admin/posts#likes
Helpers
In addition to regular helpers linkTo
, formFor
, javascriptIncludeTag
, formFor
, etc. there are also helpers for routing: each route generates a helper method that can be invoked in a view:
<%- link_to("New post", newAdminPost) %><%- link_to("New post", editAdminPost(post)) %>
generates output:
New postNew post
Controllers
The controller is a module containing the declaration of actions such as this:
; ; ; ; ; ; { Post;}
Generators
Compound offers several built-in generators: for a model, controller and for initialization. Can be invoked as follows:
compound generate what params
what
can be model
, controller
or scaffold
. Example of controller generation:
$ compound generate controller admin/posts index new edit update
exists app/
exists app/controllers/
create app/controllers/admin/
create app/controllers/admin/posts_controller.js
create app/helpers/
create app/helpers/admin/
create app/helpers/admin/posts_helper.js
exists app/views/
create app/views/admin/
create app/views/admin/posts/
create app/views/admin/posts/index.ejs
create app/views/admin/posts/new.ejs
create app/views/admin/posts/edit.ejs
create app/views/admin/posts/update.ejs
Currently it generates only *.ejs
views
Models
Checkout JugglingDB docs to see how to work with models.
CompoundJS Event model
Compound application loading process supports following events to be attached (in chronological order):
- configure
- after configure
- routes
- extensions
- after extensions
- structure
- models
- initializers
REPL console
To run REPL console use command
compound console
or its shortcut
compound c
It's just simple node-js console with some Compound bindings, e.g. models. Just one note
about working with console: Node.js is asynchronous by its nature, and it's great
but it made console debugging much more complicated, because you should use callbacks
to fetch results from the database, for example. I have added one useful method to
simplify async debugging using compound console. It's named c
. You can pass it
as a parameter to any function requiring callbacks, and it will store parameters passed
to the callback as variables _0, _1, ..., _N
where N is index in arguments
.
Example:
$ compound c
compound> User.find(53, c)
Callback called with 2 arguments:
_0 = null
_1 = [object Object]
compound> _1
{ email: [Getter/Setter],
password: [Getter/Setter],
activationCode: [Getter/Setter],
activated: [Getter/Setter],
forcePassChange: [Getter/Setter],
isAdmin: [Getter/Setter],
id: [Getter/Setter] }
Localization
To add another language to app just create a .yml file in config/locales
,
for example config/locales/jp.yml
, copy contents of config/locales/en.yml
to new
file and rename root node (en
to jp
in that case), also in lang
section rename
name
to Japanese (for example).
Next step - rename email files in app/views/emails
, copy all files *.en.html
and *.en.text
to *.jp.html
and *.jp.text
and translate new files.
NOTE: translation can contain %
symbol(s), that means variable substitution
If you don't need locales support you can turn it off in config/environment
:
app;
Logger
app; // force logger to log into `log/#{app.settings.env}.log`compoundlogger; // to log message
setup custom log dir:
app;
Configuring
Compound has some configuration options allows to customize app behavior
eval cache
Enable controller caching, should be turned on in prod. In development mode, disabling the cache allows the avoidance of server restarts after each model/controller change.
app; // in config/environments/development.jsapp; // in config/environments/production.js
model cache
Same option for models. When disabled, model files evaluated per each request.
app; // in config/environments/development.js
view cache
Express.js option, enables view caching.
app; // in config/environments/development.js
quiet
Write logs to log/NODE_ENV.log
app; // in config/environments/test.js
merge javascripts
Join all javascript files listed in javascript_include_tag
into one
app; // in config/environments/production.js
merge stylesheets
Join all stylesheet files listed in stylesheets_include_tag
into one
app; // in config/environments/production.js
Custom tools
Put your function to ./app/tools/toolname.js to be able to run it within application
environment as compound toolname
command via CLI. See example tool in generated
example: ./app/tools/dabatase.js
Optionally you can specify some usage information on your function to be able to see
it in list of available commands (using compound
command).
moduleexportshelp = shortcut: 'db' usage: 'db [migrate|update]' description: 'Migrate or update database(s)';
MIT License
Copyright (C) 2011 by Anatoliy Chakkaev <mail [åt] anatoliy [døt] in>
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.