Colorix
Colorix provides a simple way to define and layer color presets, and helps you recognize and track ANSI escape sequences in your code. Each module uses template literals (and a bit of magic 🪄) to construct a type representation of the color sequences applied to your strings.
import cx from 'colorix'
const goblinInk = cx('bgGreen', 'black', 'bold')
console.log(goblinInk('hello goblin', '!'))
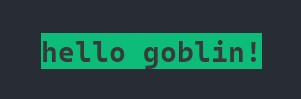
With TypeScript, always know when a string has hidden characters
declare const goblinMessage: Colorix<['bgGreen', 'black', 'bold'], ['hello goblin', '!']>
// => `\u001B[42;30;1mhello goblin!\u001B[0m`
This is useful with primitive strings as well
declare const goblinMessage: Colorix<['bgGreen', 'black', 'bold'], [string, ...string[]]>
// => `\u001B[42;30;1m${string}\u001B[0m`
How it works
// create a theme by passing colors to the first function.
declare const cx: <Colors extends Color[]>(
...colors: Colors
) => // then pass stringifiable values to the second function to colorize them.
<Strings extends Stringifiable[]>(
...strings: Strings
) => // the returned value is an ansified string.
Colorix<Colors, Strings>
Installation
Add colorix
to your project using your favorite package manager.
NPM
PNPM
Yarn
How to support terminals without color
safe
, colorixSafe
, cxs
You can use safe
to check if the terminal supports color before applying a preset.
import cx, { safe } from 'colorix'
const errorInk = cx('bold', 'red')
console.log(errorInk('That tasted purple...'))
// "\u001B[mThat tasted purple...\u001B[0m"
console.log(safe(errorInk)('That tasted purple...'))
// "That tasted purple..." | "\u001B[mThat tasted purple...\u001B[0m"
Alternatively, use colorixSafe
/ cxs
for an Ink
preset that only applies colors if the terminal supports it.
import { cxs, colorixSafe } from 'colorix'
const safeErrorInk = cxs('bold', 'red') // or colorixSafe('bold', 'red')
console.log(safeErrorInk('That tasted purple...'))
Bonus Features
ColorixError
Fun way to colorize error messages without worrying about the terminal supporting color, or importing colorix
/ cx
/ safe
into many files.
import { ColorixError } from 'colorix'
const BasicError = new ColorixError(
'simple message that is always safe to display'
)
const PrettyError = new ColorixError((cx) =>
cx(
'white',
'bgBlue',
'dim',
'bold'
)(
'Pretty Message',
cx('reset')(' '),
cx('underline', 'green')(
'npmjs.com/package/colorix',
cx('reset', 'italic')(' '),
'(this message will always be stripped of color if supportsColor is false)'
)
)
)
throw PrettyError
PrettyError
For an "out-of-the-box" solution, use PrettyError
. It provides the same naming and fallback message behavior as Colorix
without an api for customizing the rest of the error.
import { PrettyError } from 'colorix'
const IOError = PrettyError('IOError', 'An unknown IO error occurred.')
try {
throw new IOError()
} catch (err) {
console.log(err)
}
try {
throw new IOError('Illegal write operation.', 'more info...')
} catch (err) {
console.log(err)
}
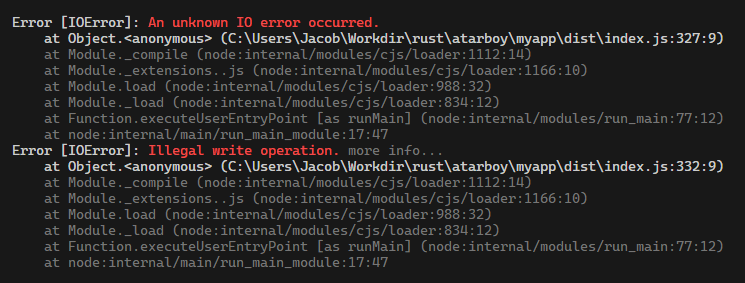
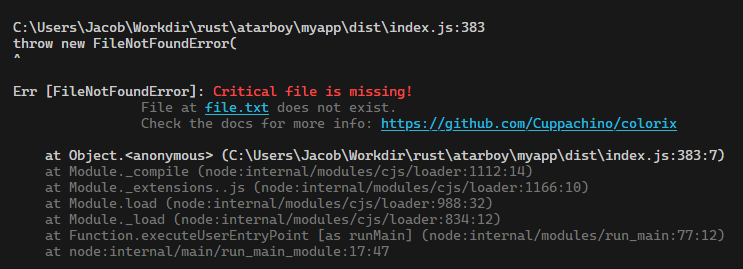
Exports
default
, colorix
, cx
Constants
Name |
Description |
CSI |
control sequence introducer ("\x1b[" ) |
SGRT |
select graphic rendition terminator ("m" ) |
FOREGROUND |
readonly foreground lookup object |
BACKGROUND |
readonly background lookup object |
MODIFIER |
readonly modifier lookup object |
COLORS |
readonly color lookup object (foreground, background, and modifiers) |
hasBasicColors |
boolean indicating if the terminal supports basic colors |
has256Colors |
boolean indicating if the terminal supports 256 colors |
has16mColors |
boolean indicating if the terminal supports 16 million colors |
supportsColor |
boolean indicating if the terminal supports any color |
Types
Generic |
Description |
Colorix |
utility for creating literals wrapped in ANSI sequences |
ColorSequence |
utility for creating literal ANSI sequences |
Alias |
Description |
ResetSequence |
literal reset sequence that is always appended to the end of a color sequence |
ColorTable |
readonly record of color aliases and color codes |
Color |
a foreground, background, or modifier color (keyof ColorTable ) |
ColorCode |
an SGR color code |
Foreground |
a foreground color |
Background |
a background color |
Modifier |
a modifier color |
Color Tables
Foreground |
Code |
Foreground Bright |
Code |
"black" |
30 |
"gray" |
90 |
"red" |
31 |
"redBright" |
91 |
"green" |
32 |
"greenBright" |
92 |
"yellow" |
33 |
"yellowBright" |
93 |
"blue" |
34 |
"blueBright" |
94 |
"magenta" |
35 |
"magentaBright" |
95 |
"cyan" |
36 |
"cyanBright" |
96 |
"white" |
37 |
"whiteBright" |
97 |
Background |
Code |
Background Bright |
Code |
"bgBlack" |
40 |
"bgGray" |
100 |
"bgRed" |
41 |
"bgRedBright" |
101 |
"bgGreen" |
42 |
"bgGreenBright" |
102 |
"bgYellow" |
43 |
"bgYellowBright" |
103 |
"bgBlue" |
44 |
"bgBlueBright" |
104 |
"bgMagenta" |
45 |
"bgMagentaBright" |
105 |
"bgCyan" |
46 |
"bgCyanBright" |
106 |
"bgWhite" |
47 |
"bgWhiteBright" |
107 |
Modifier |
Code |
"reset" |
0 |
"bold" |
1 |
"dim" |
2 |
"italic" |
3 |
"underline" |
4 |
"inverse" |
7 |
"hidden" |
8 |
"strikethrough" |
9 |