Blockstream JS Client 🙂
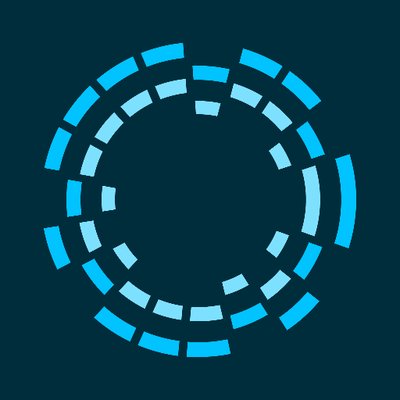
Overview
Find the Documentation for the Blockstream Satellite API here
Install
npm install blockstream-js-client
Usage
Based on the node environment, this package will shuttle between the main network or the test network.
const B = ; // Create an Order on the main or test blockstream networkconst auth_token uuid = await B; // Edit BidB ; // Monitor the status of the order / Get orderB ; // Delete OrderB ; // Get pending, sent and queued ordersB ; // Get information about the c-lightning node APIB ; // Subscribe to channels (The only available channel now is the "transmissions" channel)B ;
API
This uses some TS definitions.
| means OR
? means OPTIONAL
-
createOrder
: Create an Order on the main or test blockstream networkUsage: createOrder({bid : string, file? : string, message?: string})
bid: Amount in millisatoshi. Required.
file: Link to an attachment. Optional.
message: Message to describe the order. Optional.Returns:
// Successerror?: false;auth_token: string;uuid: string;lightning_invoice_id: string;description: string;status: string;msatoshi: string | number;payreq: string;expires_at: number;created_at: number;metadata: object;// Errorerror?: true;message: string; -
increaseBid
: Increase the bid for a particular orderUsage: increaseBid({auth_token : string, uuid : string, bid_increase : string })
auth_token: Authentication token. Required.
uuid: Universally unique identifier. Required.
bid_increase: Amount to increase bid by, Millisatoshi. Required.Returns:
// Successerror?: false;auth_token: string;uuid: string;lightning_invoice_id: string;description: string;status: string;msatoshi: string | number;payreq: string;expires_at: number;created_at: number;metadata: object;// Errorerror?: true;message: string; -
getOrder
: Fetch the details of a particular orderUsage: getOrder({uuid: string, auth_token: string})
uuid: Required.
auth_token: Required.Returns:
//Successerror?: false;bid: number | string;message_digest: string;status: string;uuid: string;created_at: Date;started_transmission_at: Date;ended_transmission_at: Date;tx_seq_num: string;unpaid_bid: number | string;// Errorerror?: true;message: string; -
getOrders
: Get orders that have either been sent, pending or queued.Usage: getOrders({state: string, limit?: number, before?: string})
state: 'pending' | 'sent' | 'queued'. Required.
limit: Limit length of response. Optional. Defaults to 20.
before: The date to query with. Optional.Returns:
//Success error?: false data: error?: false; bid: number | string; message_digest: string; status: string; uuid: string; created_at: Date; started_transmission_at: Date; ended_transmission_at: Date; tx_seq_num: string; unpaid_bid: number | string; ; // Error error?: true; message: string;
-
deleteOrder
: Delete a Particular OrderUsage: deleteOrder({uuid: string, auth_token: string})
uuid: Required.
auth_token: Required.Returns:
//Success error?: false message: string // Error error?: true; message: string;
-
getInfo
: Get information about the c-lightning node.Usage: getInfo()
Returns:
//Success error: false; id: string; alias: string; color: string; number_of_peers: string | number; number_of_active_channels: string | number; number_of_inactive_channels: string | number; number_of_pending_channels: string | number; binding: Array<object>; msatoshi_fees_collected: string | number; fees_collected_msat: string; lightning_directory: string; address: Array<object>; version: string; blockheight: string | number; network: string; // Error error?: true; message: string;
-
subscribeToChannels
: Subscribe to server-sent event channels. The only channel available now, is the transmissions channel.
Usage: subscribeToChannels(channels: Array)channels: Array of channels. Required.
Returns:
//Success error?: false message: string // Error error?: true; message: string;