Bananahooks
"Collection" of custom React Hooks.
Requirements:
- React 16.8.0 or higher
Install
npm install bananahooks
or
yarn add bananahooks
Develop
yarn install
yarn test
yarn build
Releases
yarn build
npm version [patch|minor|major]
npm publish
usePromise
Custom React Hook that properly awaits a promise to resolve.
It automatically 'unsubscribes' if the component is unmounted.
Here's an example on CodeSandbox:
Usage
Import the function:
;
Basic usage:
const App = { const bananas = return <ul> bananas </ul> }
The first render will use the empty array passed as a default value.
The next render will -probably- have some bananas in the bananas
array.
Note that there is no need to useState
to keep track of bananas
.
Advanced usage:
Handling errors and loading state.
const App = { const bananas error pending = return <ul> pending && <li>Loading...</li> !pending && error && <li>Error! error</li>) !pending && !error && bananas </ul> }
On the first render pending
is true and the "loading" message is rendered.
The next time the promise will have resolved or rejected.
error
will be 'truthy' if the promise was rejected, and error message can be displayed.
In most cases the promise will have resolved and the bananas
array is displayed.
This project was bootstrapped with TSDX:
TSDX Bootstrap
This project was bootstrapped with TSDX.
Local Development
Below is a list of commands you will probably find useful.
npm start
or yarn start
Runs the project in development/watch mode. Your project will be rebuilt upon changes. TSDX has a special logger for you convenience. Error messages are pretty printed and formatted for compatibility VS Code's Problems tab.
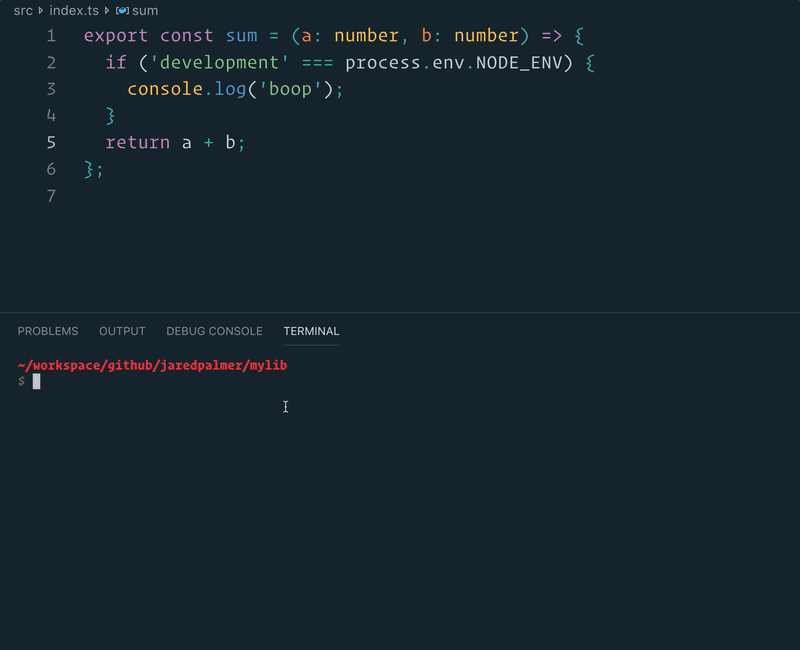
Your library will be rebuilt if you make edits.
npm run build
or yarn build
Bundles the package to the dist
folder.
The package is optimized and bundled with Rollup into multiple formats (CommonJS, UMD, and ES Module).
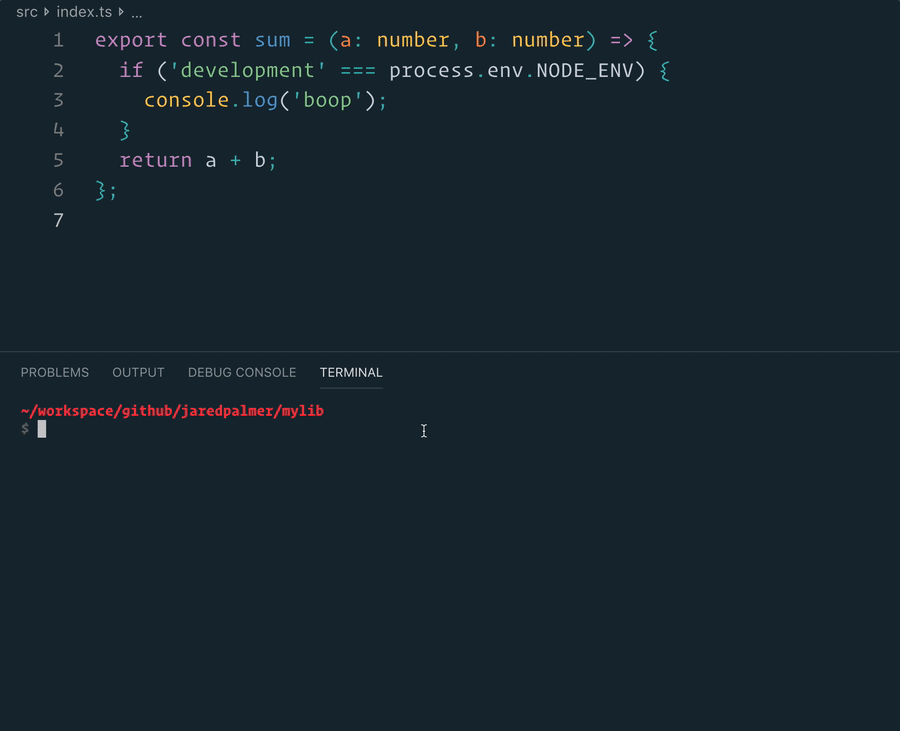
npm test
or yarn test
Runs the test watcher (Jest) in an interactive mode. By default, runs tests related to files changed since the last commit.