babel-plugin-react-css-modules
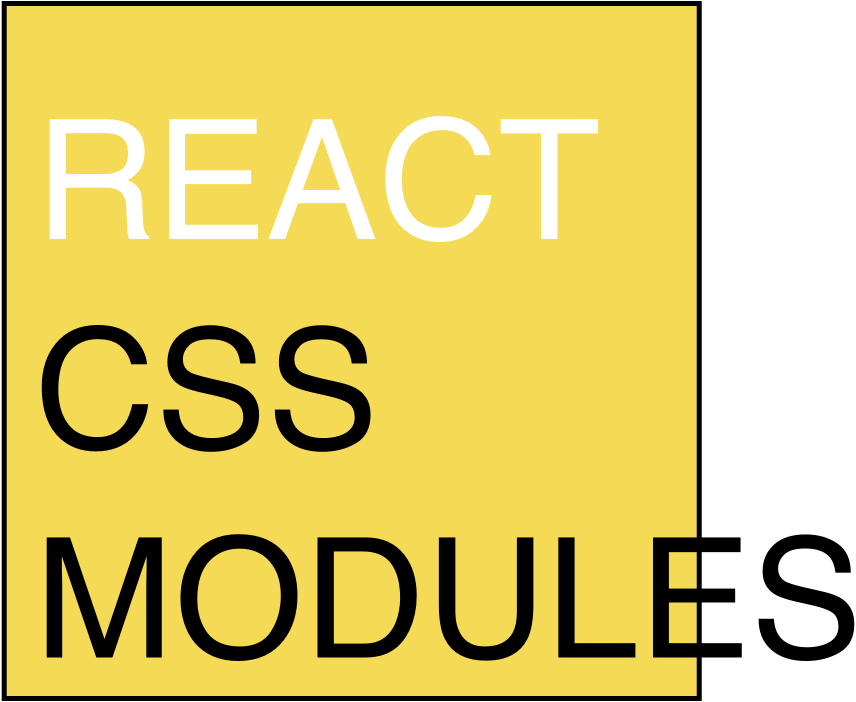
Transforms styleName
to className
using compile time CSS module resolution.
In contrast to react-css-modules
, babel-plugin-react-css-modules
has a lot smaller performance overhead (0-10% vs +50%; see Performance) and a lot smaller size footprint (less than 2kb vs 17kb react-css-modules + lodash dependency).
- CSS Modules
- Difference from
react-css-modules
- Performance
- How does it work?
- Conventions
- Configuration
- Installation
- Example transpilations
- Limitations
- Have a question or want to suggest an improvement?
- FAQ
CSS Modules
CSS Modules are awesome! If you are not familiar with CSS Modules, it is a concept of using a module bundler such as webpack to load CSS scoped to a particular document. CSS module loader will generate a unique name for each CSS class at the time of loading the CSS document (Interoperable CSS to be precise). To see CSS Modules in practice, webpack-demo.
In the context of React, CSS Modules look like this:
;; Component { return <div className=stylestable> <div className=stylesrow> <div className=stylescell>A0</div> <div className=stylescell>B0</div> </div> </div>; }
Rendering the component will produce a markup similar to:
<div class="table__table___32osj"> <div class="table__row___2w27N"> <div class="table__cell___1oVw5">A0</div> <div class="table__cell___1oVw5">B0</div> </div></div>
and a corresponding CSS file that matches those CSS classes.
Awesome!
However, there are several disadvantages of using CSS modules this way:
- You have to use
camelCase
CSS class names. - You have to use
styles
object whenever constructing aclassName
. - Mixing CSS Modules and global CSS classes is cumbersome.
- Reference to an undefined CSS Module resolves to
undefined
without a warning.
babel-plugin-react-css-modules
automates loading of CSS Modules using styleName
property, e.g.
;; Component { return <div styleName='table'> <div styleName='row'> <div styleName='cell'>A0</div> <div styleName='cell'>B0</div> </div> </div>; }
Using babel-plugin-react-css-modules
:
-
You are not forced to use the
camelCase
naming convention. -
You do not need to refer to the
styles
object every time you use a CSS Module. -
There is clear distinction between global CSS and CSS modules, e.g.
<div className='global-css' styleName='local-module'></div>
react-css-modules
Difference from react-css-modules
introduced a convention of using styleName
attribute to reference CSS module. react-css-modules
is a higher-order React component. It is using the styleName
value to construct the className
value at the run-time. This abstraction frees a developer from needing to reference the imported styles object when using CSS modules (What's the problem?). However, this approach has a measurable performance penalty (see Performance).
babel-plugin-react-css-modules
solves the developer experience problem without impacting the performance.
Performance
The important metric here is the "Difference from the base benchmark". "base" is defined as using React with hardcoded className
values. The lesser the difference, the bigger the performance impact.
Note: This benchmark suite does not include a scenario when
babel-plugin-react-css-modules
can statically construct a literal value at the build time. If a literal value of theclassName
is constructed at the compile time, the performance is equal to the base benchmark.
Name | Operations per second (relative margin of error) | Sample size | Difference from the base benchmark |
---|---|---|---|
Using className (base) |
9551 (±1.47%) | 587 | -0% |
react-css-modules |
5914 (±2.01%) | 363 | -61% |
babel-plugin-react-css-modules (runtime, anonymous) |
9145 (±1.94%) | 540 | -4% |
babel-plugin-react-css-modules (runtime, named) |
8786 (±1.59%) | 527 | -8% |
Platform info:
- Darwin 16.1.0 x64
- Node.JS 7.1.0
- V8 5.4.500.36
- NODE_ENV=production
- Intel(R) Core(TM) i7-4870HQ CPU @ 2.50GHz × 8
View the ./benchmark.
Run the benchmark:
git clone git@github.com:gajus/babel-plugin-react-css-modules.gitcd ./babel-plugin-react-css-modulesnpm installnpm run buildcd ./benchmarknpm installNODE_ENV=production ./test
How does it work?
- Builds index of all stylesheet imports per file (imports of files with
.css
or.scss
extension). - Uses postcss to parse the matching CSS files.
- Iterates through all JSX element declarations.
- Parses the
styleName
attribute value into anonymous and named CSS module references. - Finds the CSS class name matching the CSS module reference:
- If
styleName
value is a string literal, generates a string literal value. - If
styleName
value is ajSXExpressionContainer
, uses a helper function (getClassName
) to construct theclassName
value at the runtime.
- If
- Removes the
styleName
attribute from the element. - Appends the resulting
className
to the existingclassName
value (createsclassName
attribute if one does not exist).
Configuration
Configure the options for the plugin within your .babelrc
as follows:
Options
Name | Type | Description | Default |
---|---|---|---|
context |
string |
Must match webpack context configuration. css-loader inherits context values from webpack. Other CSS module implementations might use different context resolution logic. |
process.cwd() |
exclude |
string |
A RegExp that will exclude otherwise included files e.g., to exclude all styles from node_modules exclude: 'node_modules' |
|
filetypes |
?FiletypesConfigurationType |
Configure postcss syntax loaders like sugarss, LESS and SCSS and extra plugins for them. | |
generateScopedName |
?GenerateScopedNameConfigurationType |
Refer to Generating scoped names. If you use this option, make sure it matches the value of localIdentName in webpack config. See this issue |
[path]___[name]__[local]___[hash:base64:5] |
removeImport |
boolean |
Remove the matching style import. This option is used to enable server-side rendering. | false |
webpackHotModuleReloading |
boolean |
Enables hot reloading of CSS in webpack | false |
handleMissingStyleName |
"throw" , "warn" , "ignore" |
Determines what should be done for undefined CSS modules (using a styleName for which there is no CSS module defined). Setting this option to "ignore" is equivalent to setting errorWhenNotFound: false in react-css-modules. |
"throw" |
attributeNames |
?AttributeNameMapType |
Refer to Custom Attribute Mapping | {"styleName": "className"} |
skip |
boolean |
Whether to apply plugin if no matching attributeNames found in the file |
false |
autoResolveMultipleImports |
boolean |
Allow multiple anonymous imports if styleName is only in one of them. |
false |
Missing a configuration? Raise an issue.
Note: The default configuration should work out of the box with the css-loader.
Option types (flow)
type FiletypeOptionsType = | +syntax: string +plugins?: $ReadOnlyArray<string | $ReadOnlyArray<string mixed>>|; type FiletypesConfigurationType = key: string: FiletypeOptionsType; type string; type GenerateScopedNameConfigurationType = GenerateScopedNameType | string; type AttributeNameMapType = key: string: string;
Configurate syntax loaders
To add support for different CSS syntaxes (e.g. SCSS), perform the following two steps:
- Add the postcss syntax loader as a development dependency:
npm install postcss-scss --save-dev
- Add a filetype syntax mapping to the Babel plugin configuration
"filetypes":
And optionaly specify extra plugins
"filetypes":
Postcss plugins can have options specified by wrapping the name and an options object in an array inside your config
"plugins":
Custom Attribute Mapping
You can set your own attribute mapping rules using the attributeNames
option.
It's an object, where keys are source attribute names and values are destination attribute names.
For example, the <NavLink> component from React Router has a activeClassName
attribute to accept an additional class name. You can set "attributeNames": { "activeStyleName": "activeClassName" }
to transform it.
The default styleName
-> className
transformation will not be affected by an attributeNames
value without a styleName
key. Of course you can use { "styleName": "somethingOther" }
to change it, or use { "styleName": null }
to disable it.
Installation
When babel-plugin-react-css-modules
cannot resolve CSS module at a compile time, it imports a helper function (read Runtime styleName
resolution). Therefore, you must install babel-plugin-react-css-modules
as a direct dependency of the project.
npm install babel-plugin-react-css-modules --save
React Native
If you'd like to get this working in React Native, you're going to have to allow custom import extensions, via a rn-cli.config.js
file:
moduleexports = { return "scss"; }
Remember, also, that the bundler caches things like plugins and presets. If you want to change your .babelrc
(to add this plugin) then you'll want to add the --reset-cache
flag to the end of the package command.
Demo
git clone git@github.com:gajus/babel-plugin-react-css-modules.gitcd ./babel-plugin-react-css-modules/demonpm installnpm start
open http://localhost:8080/
Conventions
Anonymous reference
Anonymous reference can be used when there is only one stylesheet import.
Format: CSS module name
.
Example:
; // Imports "a" CSS module from ./foo1.css.<div styleName="a"></div>;
Named reference
Named reference is used to refer to a specific stylesheet import.
Format: [name of the import].[CSS module name]
.
Example:
;; // Imports "a" CSS module from ./foo1.css.<div styleName="foo.a"></div>; // Imports "a" CSS module from ./bar1.css.<div styleName="bar.a"></div>;
Example transpilations
styleName
resolution
Anonymous When styleName
is a literal string value, babel-plugin-react-css-modules
resolves the value of className
at the compile time.
Input:
; <div styleName="a"></div>;
Output:
; <div className="bar___a"></div>;
styleName
resolution
Named When a file imports multiple stylesheets, you must use a named reference.
Have suggestions for an alternative behaviour? Raise an issue with your suggestion.
Input:
;; <div styleName="foo.a"></div>;<div styleName="bar.a"></div>;
Output:
;; <div className="foo___a"></div>;<div className="bar___a"></div>;
styleName
resolution
Runtime When the value of styleName
cannot be determined at the compile time, babel-plugin-react-css-modules
inlines all possible styles into the file. It then uses getClassName
helper function to resolve the styleName
value at the runtime.
Input:
; <div styleName=Math > 5 ? 'a' : 'b'></div>;
Output:
;; const _styleModuleImportMap = foo: a: 'bar__a' b: 'bar__b' ; <div styleName=></div>;
Limitations
Have a question or want to suggest an improvement?
- Have a technical questions? Ask on Stack Overflow.
- Have a feature suggestion or want to report an issue? Raise an issues.
- Want to say hello to other
babel-plugin-react-css-modules
users? Chat on Gitter.
FAQ
How to migrate from react-css-modules to babel-plugin-react-css-modules?
Follow the following steps:
- Remove
react-css-modules
. - Add
babel-plugin-react-css-modules
. - Configure
.babelrc
(see Configuration). - Remove all uses of the
cssModules
decorator and/or HoC.
If you are still having problems, refer to one of the user submitted guides:
How to reference multiple CSS modules?
react-css-modules
had an option allowMultiple
. allowMultiple
allows multiple CSS module names in a styleName
declaration, e.g.
<div styleName='foo bar' />
This behaviour is enabled by default in babel-plugin-react-css-modules
.
How to live reload the CSS?
babel-plugin-react-css-modules
utilises webpack Hot Module Replacement (HMR) to live reload the CSS.
To enable live reloading of the CSS:
- Enable
webpackHotModuleReloading
babel-plugin-react-css-modules
configuration. - Configure
webpack
to use HMR. Use--hot
option if you are usingwebpack-dev-server
. - Use
style-loader
to load the style sheets.
Note:
This enables live reloading of the CSS. To enable HMR of the React components, refer to the Hot Module Replacement - React guide.
Note:
This is a webpack specific option. If you are using
babel-plugin-react-css-modules
in a different setup and require CSS live reloading, raise an issue describing your setup.