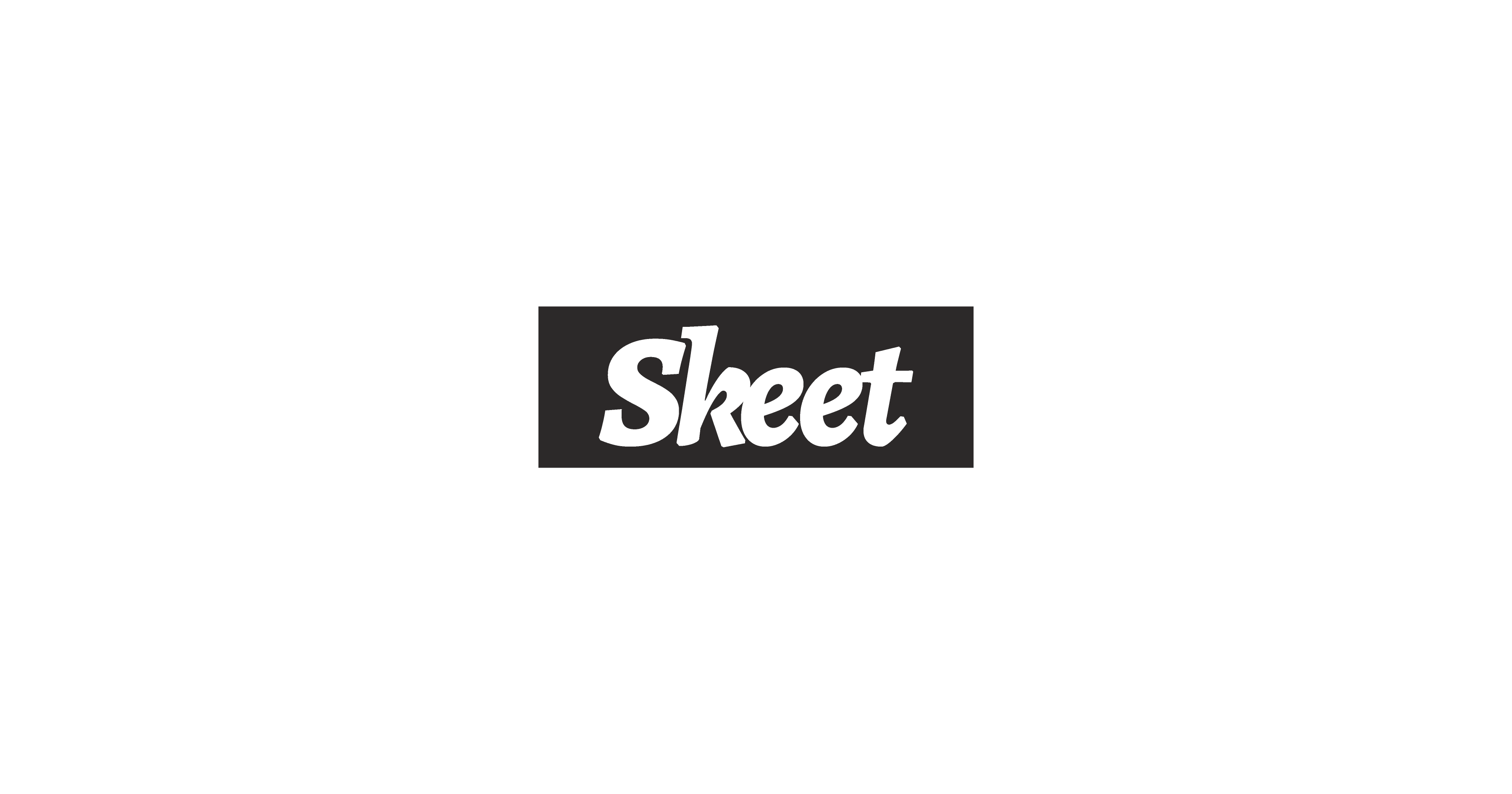
Skeet Firestore Plugin for CRUD Firestore operation with Firestore Converter. Type safe, easy to use, and easy to test. This plugin is for serverside with Firebase Admin SDK. (Client side helper functions for Firestore is in ./lib/skeet/firestore on the Skeet Client)
$ pnpm add @skeet-framework/firestore
All CRUD operations are supported with Firestore Converter. createdAt and updatedAt are automatically added to the document with Firebase ServerTimestamp.
- [x] Add Collection Item
- [x] Adds Collection Items
- [x] Get Collection Item
- [x] Query Collection Items
- [x] Update Collection Item
- [x] Delete Collection Item
import { applicationDefault, initializeApp } from 'firebase-admin/app'
import { getFirestore } from 'firebase-admin/firestore'
const firebaseApp = initializeApp({
credential: applicationDefault(),
})
export const db = getFirestore(firebaseApp)
import { add } from '@skeet-framework/firestore'
import { applicationDefault, initializeApp } from 'firebase-admin/app'
import { getFirestore } from 'firebase-admin/firestore'
const firebaseApp = initializeApp({
credential: applicationDefault(),
})
export const db = getFirestore(firebaseApp)
const data: User = {
name: 'John Doe',
age: 30,
}
async function run() {
try {
const path = 'Users'
const docRef = await add<User>(db, path, data)
console.log(`Document added with ID: ${docRef.id}`)
} catch (error) {
console.error(`Error adding document: ${error}`)
}
}
run()
import { adds } from '@skeet-framework/firestore'
import { applicationDefault, initializeApp } from 'firebase-admin/app'
import { getFirestore } from 'firebase-admin/firestore'
const firebaseApp = initializeApp({
credential: applicationDefault(),
})
export const db = getFirestore(firebaseApp)
const users: User[] = [
{ name: 'John Doe', age: 30 },
{ name: 'Jane Smith', age: 25 },
// ... more users ...
]
async function run() {
try {
const path = 'Users'
const results = await adds<User>(db, path, users)
console.log(`Added ${users.length} users in ${results.length} batches.`)
} catch (error) {
console.error(`Error adding documents: ${error}`)
}
}
run()
import { get } from '@skeet-framework/firestore'
import { applicationDefault, initializeApp } from 'firebase-admin/app'
import { getFirestore } from 'firebase-admin/firestore'
const firebaseApp = initializeApp({
credential: applicationDefault(),
})
export const db = getFirestore(firebaseApp)
async function run() {
try {
const path = 'Users'
const docId = 'user123'
const user = await get<User>(db, path, docId)
console.log(`User: ${JSON.stringify(user)}`)
} catch (error) {
console.error(`Error getting document: ${error}`)
}
}
run()
import { query, QueryCondition } from '@skeet-framework/firestore'
import { applicationDefault, initializeApp } from 'firebase-admin/app'
import { getFirestore } from 'firebase-admin/firestore'
const firebaseApp = initializeApp({
credential: applicationDefault(),
})
export const db = getFirestore(firebaseApp)
// Simple query to get users over 25 years old
const simpleConditions: QueryCondition[] = [
{ field: 'age', operator: '>', value: 25 },
]
// Advanced query to get users over 25 years old, ordered by desc
// Limitations: If you include a filter with a range comparison (<, <=, >, >=), your first ordering must be on the same field
// So we can't use multiple fields with a range comparison for now.
// https://firebase.google.com/docs/firestore/query-data/order-limit-data
const advancedConditions: QueryCondition[] = [
{ field: 'age', operator: '>', value: 25 },
{ field: 'age', orderDirection: 'desc' },
]
// Query to get users over 25 years old and limit the results to 5
const limitedConditions: QueryCondition[] = [
{ field: 'age', operator: '>', value: 25 },
{ limit: 5 },
]
async function run() {
try {
const path = 'Users'
// Using the simple conditions
const usersByAge = await query<User>(db, path, simpleConditions)
console.log(`Found ${usersByAge.length} users over 25 years old.`)
// Using the advanced conditions
const orderedUsers = await query<User>(db, path, advancedConditions)
console.log(
`Found ${orderedUsers.length} users over 25 years old, ordered by name.`,
)
// Using the limited conditions
const limitedUsers = await query<User>(db, path, limitedConditions)
console.log(
`Found ${limitedUsers.length} users over 25 years old, limited to 5.`,
)
} catch (error) {
console.error(`Error querying collection: ${error}`)
}
}
run()
import { update } from '@skeet-framework/firestore'
import { applicationDefault, initializeApp } from 'firebase-admin/app'
import { getFirestore } from 'firebase-admin/firestore'
const firebaseApp = initializeApp({
credential: applicationDefault(),
})
export const db = getFirestore(firebaseApp)
const updatedData: User = {
age: 38,
}
async function run() {
try {
const path = 'Users'
const docId = '123456'
const success = await update<User>(db, path, docId, updatedData)
if (success) {
console.log(`Document with ID ${docId} updated successfully.`)
}
} catch (error) {
console.error(`Error updating document: ${error}`)
}
}
run()
import { remove } from '@skeet-framework/firestore'
import { applicationDefault, initializeApp } from 'firebase-admin/app'
import { getFirestore } from 'firebase-admin/firestore'
const firebaseApp = initializeApp({
credential: applicationDefault(),
})
export const db = getFirestore(firebaseApp)
async function run() {
try {
const path = 'Users'
const docId = '123456'
const success = await remove(db, path, docId)
if (success) {
console.log(`Document with ID ${docId} removed successfully.`)
}
} catch (error) {
console.error(`Error deleting document: ${error}`)
}
}
Bug reports and pull requests are welcome on GitHub at https://github.com/elsoul/skeet This project is intended to be a safe, welcoming space for collaboration, and contributors are expected to adhere to the Contributor Covenant code of conduct.
The package is available as open source under the terms of the Apache-2.0 License.
Everyone interacting in the SKEET project’s codebases, issue trackers, chat rooms and mailing lists is expected to follow the code of conduct.